解决Spring Security中的常见问题:权限不足和配置错误案例分析的快速指南
发布时间: 2024-10-22 12:36:20 阅读量: 33 订阅数: 44 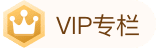
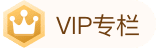
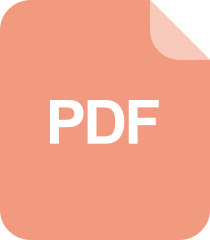
Spring Security3 中文版 张卫滨 推荐

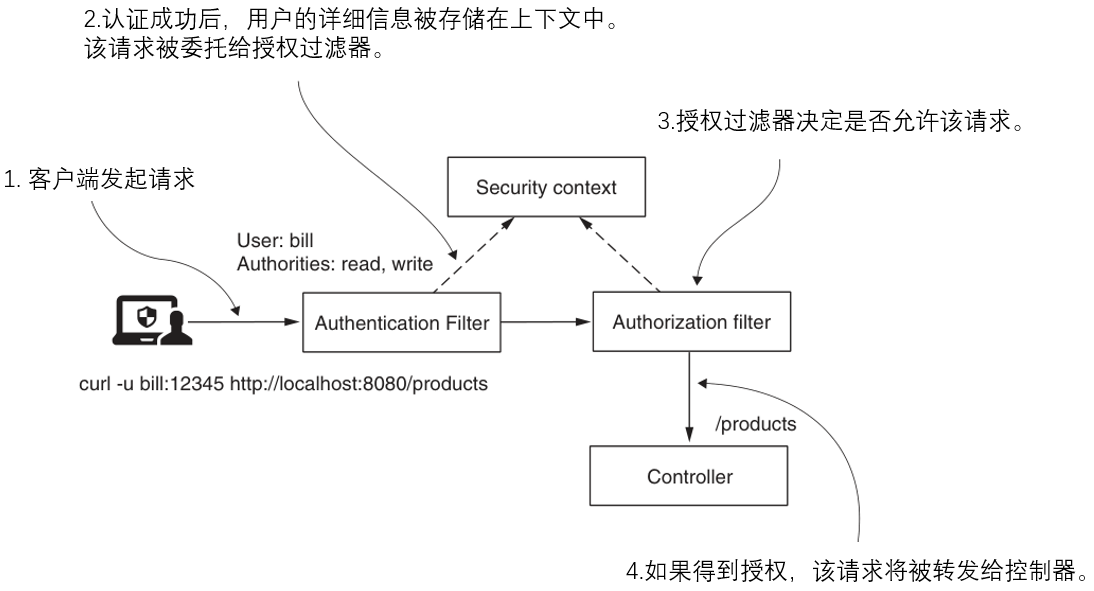
# 1. Spring Security简介与核心概念
## Spring Security概述
Spring Security是为Java应用提供声明式安全访问控制和认证的一个框架,广泛用于Web应用安全、方法安全和企业级认证。它的核心在于提供了一套灵活且可扩展的安全机制,允许开发者自定义安全策略来满足特定的安全需求。
## 核心组件
Spring Security的核心组件包括认证(Authentication)、授权(Authorization)、CSRF防护等。认证是确认用户身份的过程,而授权则是确定用户可以访问哪些资源。CSRF防护是为了防止跨站请求伪造攻击。
## 核心概念
- **认证**:通常是通过用户名和密码进行,Spring Security支持多种认证方式,如表单登录、LDAP、OAuth等。
- **授权**:在认证后,Spring Security根据用户的角色和权限来决定访问控制。它提供了一系列的接口来实现复杂的权限逻辑。
- **过滤器链**:Spring Security使用过滤器链来处理HTTP请求,每个过滤器负责安全的某一部分功能,如认证、方法安全等。
通过本章的学习,读者将对Spring Security有一个宏观的理解,并为进一步探索其核心功能和高级应用打下基础。接下来章节将深入探讨权限不足问题及其解决方案。
# 2. 权限不足问题的诊断与解决方案
### 2.1 权限不足问题的常见原因
#### 2.1.1 认证流程分析
在处理权限不足问题时,首先需要理解Spring Security的认证流程。认证流程是用户访问系统资源前,系统对用户身份的确认过程。在Spring Security中,认证通常涉及以下几个核心组件:
- **AuthenticationManager**:负责认证流程的入口点,接收认证请求并返回一个填充了认证信息的`Authentication`对象。
- **UserDetailsService**:从存储介质中加载用户详细信息,如用户名、密码和权限等。
- **AuthenticationProvider**:使用`UserDetailsService`提供的用户详细信息,对用户提交的认证信息进行验证。
- **GrantedAuthority**:代表用户被授予的权限。
认证流程中的错误配置或逻辑缺陷往往是导致权限不足的首要原因。例如,如果`AuthenticationProvider`的实现逻辑错误,或者`UserDetailsService`返回了错误的用户信息,都可能导致认证失败。
**代码块示例**:
```java
@Service
public class CustomAuthenticationProvider implements AuthenticationProvider {
@Autowired
private UserDetailsService userDetailsService;
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
// 获取用户信息
UserDetails userDetails = userDetailsService.loadUserByUsername(authentication.getName());
// 验证用户密码等信息
if (passwordEncoder.matches(authentication.getCredentials().toString(), userDetails.getPassword())) {
return new UsernamePasswordAuthenticationToken(userDetails, userDetails.getPassword(), userDetails.getAuthorities());
}
throw new BadCredentialsException("Authentication Failed.");
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
```
**代码逻辑分析**:
- `authenticate`方法是实现用户认证的关键方法,需要接收`Authentication`对象作为输入参数,并在验证通过后返回该对象。
- `supports`方法用于指定当前`AuthenticationProvider`支持的`Authentication`类型,这里指定了用户名/密码认证方式。
#### 2.1.2 权限控制配置不当
权限控制通常依赖于`SecurityConfig`配置,而配置不当可能导致权限分配错误。在Spring Security中,`WebSecurityConfigurerAdapter`是常用的配置基类,通过重写`configure(HttpSecurity http)`方法来设置权限规则。
权限配置的问题可能包括:
- 不恰当的HTTP安全规则,例如对公共资源使用`authenticated()`要求认证。
- 资源访问控制表达式错误,比如使用错误的权限名称或角色。
- 方法级别的权限控制未正确配置或与URL级别的权限控制冲突。
**代码块示例**:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN") // 限制只有管理员可以访问/admin路径
.antMatchers("/public/**").permitAll() // 允许所有人访问/public路径
.anyRequest().authenticated() // 其他所有请求都需要认证
.and()
.formLogin() // 使用表单登录
.and()
.logout() // 配置登出
.permitAll();
}
}
```
**参数说明**:
- `antMatchers`定义了对特定URL路径的访问控制规则。
- `hasRole`和`permitAll`用于声明访问权限,分别对应"只有具有特定角色的用户"和"所有人都可以访问"。
- `anyRequest().authenticated()`确保所有其他请求都需要通过认证。
### 2.2 实践中的权限管理优化
#### 2.2.1 基于角色的访问控制(RBAC)
基于角色的访问控制(RBAC)是一种权限管理策略,它根据用户的角色分配访问权限。在Spring Security中实现RBAC时,可以使用`SimpleAuthorityUtils`或自定义`GrantedAuthority`来管理用户角色与权限的关系。
**代码块示例**:
```java
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Override
@Transactional
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
// 假设从数据库加载用户信息
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
List<GrantedAuthority> authorities = user.getRoles().stream()
.flatMap(role -> role.getPermissions().stream())
.map(permission -> new SimpleGrantedAuthority(permission.getName()))
.collect(Collectors.toList());
return new org.springframework.security.core.userdetails.User(
user.getUsername(), user.getPassword(), authorities);
}
}
```
**代码逻辑分析**:
- 在`loadUserByUsername`方法中,首先从数据库加载用户信息。
- 接着,将用户角色的权限名称转换为`SimpleGrantedAuthority`实例列表。
- 最后,返回填充了权限信息的`UserDetails`对象。
#### 2.2.2 方法级别的安全配置
除了URL级别的权限控制之外,Spring Security还支持通过注解来进行方法级别的安全配置。使用`@Secured`、`@PreAuthorize`和`@PostAuthorize`等注解,可以直接在方法前声明访问控制逻辑。
**代码块示例**:
```java
@RestController
@RequestMapping("/api")
public class ExampleController {
@PreAuthorize("hasRole('USER')")
@GetMapping("/user")
public ResponseEntity<String> getUserData() {
return ResponseEntity.ok("User data");
}
@Secured("ROLE_ADMIN")
@PostMapping("/admin")
public ResponseEntity<String> postAdminData() {
return ResponseEntity.ok("Admin data");
}
}
```
**逻辑分析**:
- 在`getUserData`方法上使用`@PreAuthorize`注解确保调用此方法的用户必须拥有`ROLE_USER`。
- 在`postAdminData`方法上使用`@Secured`注解,限制只有`ROLE_ADMIN`的用户可以执行此方法。
#### 2.2.3 自定义权限评估策略
在某些高级用例中,Spring Security允许开发者自定义权限评估策略。这涉及到扩展`AccessDecisionManager`,它负责基于当前`Authentication`对象和`ConfigAttribute`集合来做出访问决策。
**代码块示例**:
```java
@Component
public class CustomAccessDecisionManager extends AccessDecisionManager {
@Override
public void decide(Authentication authentication, Object object, Collection<ConfigAttribute> configAttributes) throws AccessDeniedException {
for (ConfigAttribute attribute : configAttributes) {
if (this.suppor
```
0
0
相关推荐
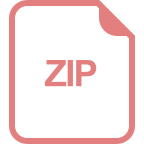
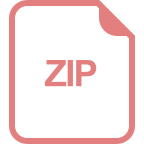
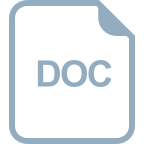
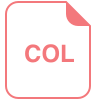
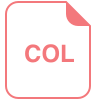
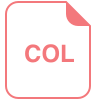
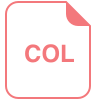
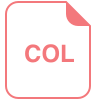