决策树模型参数调优:网格搜索与贝叶斯优化的最佳实践
发布时间: 2024-09-04 22:05:39 阅读量: 141 订阅数: 48 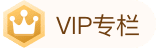
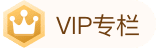
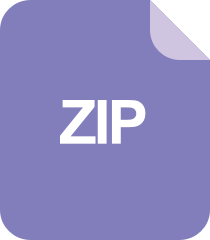
【java毕业设计】智慧社区教育服务门户.zip
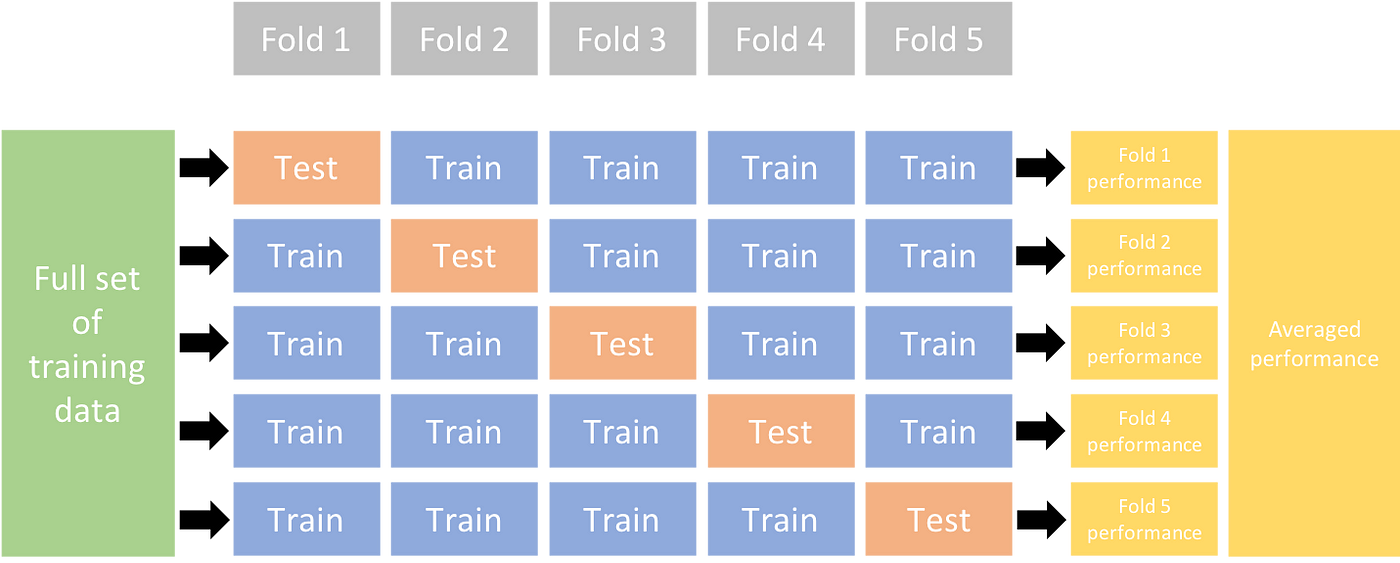
# 1. 决策树模型参数调优基础
决策树模型因其简单直观而广泛应用于分类和回归问题。然而,为了达到最佳的预测效果,对其进行参数调优是必不可少的步骤。本章将探讨决策树参数调优的基础知识,包括关键参数的作用、如何正确设置参数以防止过拟合或欠拟合等问题。我们将从以下几个方面展开:
## 1.1 决策树模型参数概述
决策树模型有许多参数,主要可以分为三类:树结构参数、剪枝参数和叶节点的不纯度度量参数。结构参数如`max_depth`、`min_samples_split`和`min_samples_leaf`影响树的深度和复杂性,剪枝参数如`max_leaf_nodes`和`min_impurity_decrease`用于控制树的过度拟合。不纯度度量参数如`criterion`定义了如何测量节点不纯度。
## 1.2 参数调优的基本策略
调优参数时,首先需要确定参数搜索的范围和可能的值,可以使用网格搜索方法,以穷举的方式测试所有组合。另一种方法是随机搜索,它在预定义的参数分布中随机选择参数值。此外,还可以根据经验或先前的实验结果缩小搜索范围,采用启发式方法,如贝叶斯优化来更智能地选择参数。
## 1.3 避免过拟合和欠拟合
避免决策树的过拟合和欠拟合是参数调优的关键目标。通过设置适当的`max_depth`和`min_samples_split`可以避免过拟合,而适当的`min_samples_leaf`可以平衡模型的复杂度和泛化能力。通过交叉验证来评估模型性能,是检测和避免这些问题的有效方法。
了解了决策树模型参数调优的基础,第二章我们将深入探讨网格搜索的理论与实践,如何通过Python实现参数优化。
# 2. 网格搜索的理论与实践
## 2.1 网格搜索概念及原理
### 2.1.1 参数调优的目标和意义
在机器学习中,模型的性能往往依赖于正确的参数选择。这些参数,又称为超参数,包括但不限于学习率、树的数量、树的深度等。超参数的选取对模型的性能有着决定性的影响。参数调优的目标是找到这些超参数的最佳组合,从而使得模型在给定的数据集上具有最佳的泛化能力。
调优的意义在于:
1. 提高模型的准确率:通过找到最佳的超参数组合,可以显著提高模型对未知数据的预测准确性。
2. 减少模型的过拟合风险:合适的参数可以帮助模型更好地泛化,避免在训练数据上过度拟合。
3. 优化计算资源:合理选择参数可以减少模型训练的时间和计算资源,提高开发和部署效率。
### 2.1.2 网格搜索的工作流程
网格搜索(Grid Search)是一种参数调优的方法,其基本思想是定义一个参数的网格,然后遍历这些参数的所有组合,使用交叉验证的方法来评估每一个参数组合,最后选出最优参数组合。
网格搜索的工作流程如下:
1. 设计参数网格:确定要优化的参数和每个参数可能取值的集合。
2. 生成参数组合:穷举所有可能的参数组合。
3. 交叉验证评估:对每个参数组合使用交叉验证来评估模型的性能。
4. 选择最优参数:根据交叉验证的结果,选取最优的参数组合。
### 2.1.3 代码实现与逻辑分析
下面是一个使用Python的`GridSearchCV`模块进行网格搜索的代码示例:
```python
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import load_digits
# 加载数据集
digits = load_digits()
X, y = digits.data, digits.target
# 定义模型
model = RandomForestClassifier()
# 定义参数网格
param_grid = {
'n_estimators': [100, 200, 300],
'max_depth': [10, 20, 30],
'min_samples_split': [2, 5, 10]
}
# 创建GridSearchCV实例
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5)
# 拟合GridSearchCV
grid_search.fit(X, y)
# 输出最优参数组合
print("Best parameters found: ", grid_search.best_params_)
```
代码逻辑分析:
- `from sklearn.model_selection import GridSearchCV`:导入`GridSearchCV`模块,这是进行网格搜索的核心工具。
- `from sklearn.ensemble import RandomForestClassifier`:导入随机森林分类器模型,我们将使用这个模型进行参数优化。
- `digits = load_digits()`:加载sklearn内置的手写数字数据集。
- `param_grid = {'n_estimators': [100, 200, 300], 'max_depth': [10, 20, 30], 'min_samples_split': [2, 5, 10]}`:定义参数网格,包括森林中的树的数量、树的最大深度和内部节点再划分所需的最小样本数。
- `grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5)`:创建一个`GridSearchCV`的实例,其中`estimator`是要进行参数优化的模型,`param_grid`是参数网格,`cv=5`表示使用5折交叉验证。
- `grid_search.fit(X, y)`:对数据集应用网格搜索,拟合模型。
- `print("Best parameters found: ", grid_search.best_params_)`:输出找到的最优参数组合。
## 2.2 网格搜索的实现方法
### 2.2.1 使用Python的GridSearchCV
`GridSearchCV`是scikit-learn库提供的一个强大的参数调优工具,它通过组合不同的参数值,自动对这些参数值的所有组合进行模型的训练和评估,最终返回最佳的参数组合。
使用`GridSearchCV`的关键步骤如下:
1. 导入`GridSearchCV`模块,并指定要优化的模型。
2. 定义参数网格,为每个超参数指定一系列可能的值。
3. 实例化`GridSearchCV`类,传入模型、参数网格和交叉验证的折数。
4. 调用`fit`方法,让`GridSearchCV`遍历所有参数组合,并对每个组合进行交叉验证评估。
5. 使用`best_params_`属性获取最优参数组合。
### 2.2.2 自定义网格搜索策略
虽然`GridSearchCV`非常方便,但在某些情况下可能需要更灵活的搜索策略。自定义网格搜索涉及到手动遍历参数组合,并对每个组合进行交叉验证。
以下是自定义网格搜索的一个例子:
```python
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
# 假设的参数网格
param_grid = {
'n_estimators': [100, 200],
'max_depth': [10, 20]
}
# 定义模型
model = RandomForestClassifier()
# 自定义网格搜索
best_score = -1
best_params = {}
for n_estimators in param_grid['n_estimators']:
for max_depth in param_grid['max_depth']:
model.set_params(n_estimators=n_estimators, max_depth=max_depth)
scores = cross_val_score(model, X, y, cv=5)
mean_score = scores.mean()
if mean_score > best_score:
best_score = mean_score
best_params = {'n_estimators': n_estimators, 'max_depth': max_depth}
print("Best parameters found: ", best_params)
```
在这段代码中,我们手动遍历参数网格,对于每一个参数组合,使用`cross_val_score`函数进行5折交叉验证,并记录下最佳的参数组合。自定义网格搜索方法给予了开发者更大的灵活性,但同时也需要更多的代码实现工作。
## 2.3 网格搜索的性能优化
### 2.3.1 减少搜索空间的策略
由于网格搜索需要遍历所有参数组合,参数的数量越多,搜索空间就越大,计算成本也越高。因此,采取措施减少搜索空间是性能优化的关键。减少搜索空间的策略包括:
- 使用知识或经验来缩小参数范围。
- 通过预实验或快速原型评估确定最有希望的参数范围。
- 使用更精细的步长在重点区域搜索
0
0
相关推荐
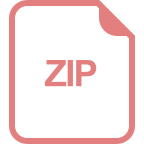
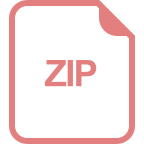
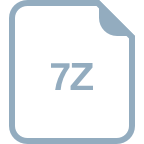
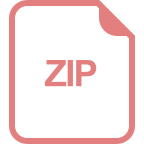
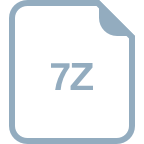
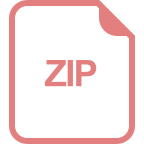