高级数据结构探索:Trie树、平衡树和跳表的深入介绍
发布时间: 2024-09-10 18:45:21 阅读量: 43 订阅数: 23 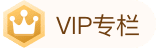
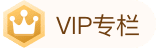
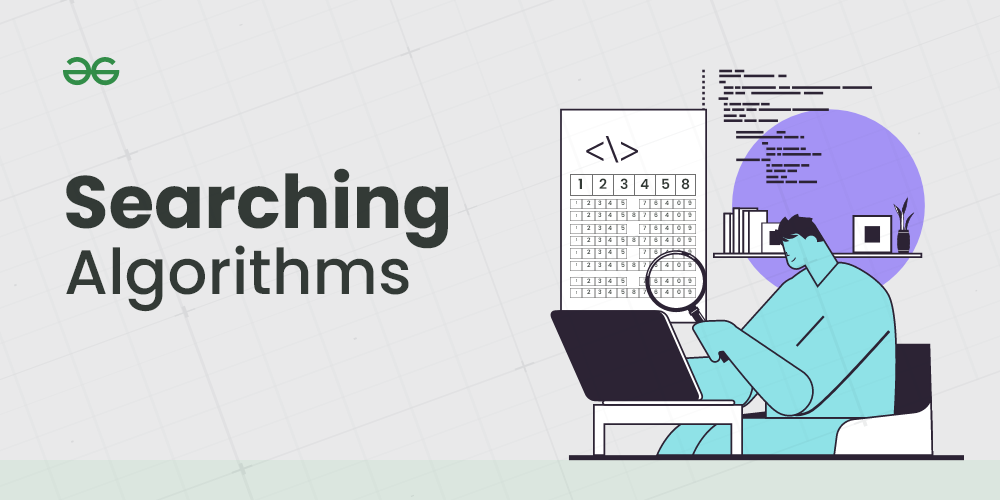
# 1. 数据结构概览与高级数据结构介绍
数据结构是计算机存储、组织数据的方式,它旨在提高数据处理的效率。在处理大量数据时,选择合适的数据结构能够显著优化算法性能和资源使用。本章我们将对常用的数据结构做一个概览,并介绍几种高级数据结构,包括Trie树、平衡树、跳表等。
## 数据结构的基本分类
数据结构通常分为线性结构和非线性结构。线性结构包括数组、链表、栈和队列等,而非线性结构包含树、图等。高级数据结构在实际应用中,如数据库索引、网络路由和搜索引擎中,有着不可替代的作用。
## 高级数据结构简介
- **Trie树(前缀树)**:用于处理字符串相关问题,如自动补全、拼写检查等。
- **平衡树**:自动调整以保持平衡,例如AVL树和红黑树,用于有序数据集合。
- **跳表**:一种支持快速插入、删除、查找操作的数据结构,类似于多级链表。
在后续章节中,我们将深入探讨这些高级数据结构的内部工作原理、实现细节以及它们在不同场景下的优化和应用。理解这些高级数据结构对于设计高性能软件系统至关重要。
# 2. Trie树基础与应用
## 2.1 Trie树的概念与特点
### 2.1.1 Trie树的定义
Trie树,也称为前缀树或字典树,是一种用于快速检索字符串数据集中的键的树形数据结构。它是以字符串为键,多用于搜索提示和自动完成系统中。Trie树的每个节点通常包含一个字符集和一个标志位,表示该节点是否是一个键的结束。
Trie树的核心优势在于其高效的插入和查找速度,尤其是当数据集包含大量字符串时。每个节点不仅存储单个字符,还可以存储整个字符串的前缀路径,这种结构化的设计允许Trie树在处理诸如文本分析等任务时,表现出卓越的性能。
### 2.1.2 Trie树的基本操作
Trie树的基本操作包括插入(insert)、查询(search)、和删除(delete)。插入操作将字符串添加到树中,从根节点开始,按照字符串的每个字符,沿着树向下遍历,为每个新字符创建新的节点。如果路径中已经存在该字符的节点,则直接移动到该节点继续插入。查询操作则相反,用于检查某个字符串是否存在于树中。删除操作相对复杂,需要找到待删除字符串的节点,并进行回溯处理,以保持树的结构完整性。
Trie树结构的关键在于能够快速找到任何给定前缀的所有键。在实现时,我们通常为每个节点分配一个布尔变量来表示一个字符串是否在此终止,以及一个数组来存储子节点(即26个英文字母的映射)。
## 2.2 Trie树的实现
### 2.2.1 Trie树的数据结构设计
Trie树的节点是实现Trie树的基础。每个节点通常包含以下部分:
- 一个字符数组,用于存储指向子节点的指针(或索引)
- 一个标志位,表示该节点是否是某个字符串的结尾
- 可选地,一个计数器,记录经过该节点的字符串数量
下面是一个简单的Trie树节点的示例代码:
```python
class TrieNode:
def __init__(self):
self.children = {} # 子节点映射
self.is_end_of_word = False # 是否为字符串结束的标志
```
### 2.2.2 Trie树的插入和查询算法
#### 插入算法
插入操作开始于根节点,根据要插入字符串的每个字符进行递归。如果当前字符对应的子节点不存在,则创建一个新的TrieNode。重复这个过程直到字符串的最后一个字符,然后将结束标志位设置为True。
```python
def insert(root, word):
node = root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
```
#### 查询算法
查询操作与插入类似,从根节点开始遍历直到字符串结束,如果遇到某个节点的结束标志位为True,则表示该字符串存在于Trie树中。此外,也可以用来查询某个前缀是否存在于树中。
```python
def search(root, word):
node = root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
```
## 2.3 Trie树的高级应用
### 2.3.1 字符串前缀匹配
Trie树能够高效地匹配字符串的前缀。这种能力特别适用于搜索引擎中的关键词匹配,或者在开发过程中需要根据部分输入提示可能的完整输入场景。Trie树可以迅速地遍历到特定前缀下的所有可能的字符串,从而实现前缀匹配。
### 2.3.2 自动补全系统
自动补全是Trie树应用中较为复杂的场景。系统可以根据用户的输入,提供可能的补全建议。这是通过递归遍历Trie树实现的,从根节点开始,选择与用户输入匹配的节点,继续递归直到找到所有以该输入为前缀的字符串。
### 2.3.3 字符串搜索优化
在进行大量字符串搜索时,Trie树能够显著减少搜索时间。尤其是当字符串数据集很大时,Trie树的优势更为明显。通过将字符串预处理并存储在Trie树中,可以实现对数据集的快速搜索,这对于需要快速反馈搜索结果的应用程序来说至关重要。
在实现优化时,可以考虑使用哈希表来快速定位到具体字符的子节点,减少遍历次数。同时,在构建Trie树时,采用适当的压缩机制,比如只存储非空子节点的指针,可以进一步优化空间使用。
在实际应用中,Trie树的这些高级特性可以带来诸多益处,包括但不限于提升检索效率、加快前缀匹配速度以及改进自动补全功能。这些优势在需要处理大量文本数据的场景中尤为突出,如搜索引擎、数据库索引、拼写检查器等。
# 3. 平衡树的原理与实践
## 3.1 平衡树的概念与性质
### 3.1.1 平衡树的定义
平衡树是一类特殊的二叉搜索树,其主要特征在于任何节点的两个子树的高度差不会超过一,这意味着从任何一个节点出发到达树的最深层的路径长度的差值不会超过一。这种严格的平衡特性使得平衡树在动态数据集合上维护了较优的搜索性能,从而避免了最坏情况下的线性时间复杂度。
### 3.1.2 平衡树的平衡条件
为了维持树的平衡,平衡树通常会实施一系列的调整操作,这些操作被称为旋转。当插入或删除节点导致树的平衡性被破坏时,通过旋转操作可以重新将树调整为平衡状态。旋转操作分为单旋转和双旋转,它们是保持树平衡的关键机制。
## 3.2 AVL树的实现与特性
### 3.2.1 AVL树的旋转操作
AVL树作为最早被发明的自平衡二叉搜索树,它通过严格的平衡因子标准来实现旋转。每个节点的平衡因子是其左子树的高度减去右子树的高度,AVL树要求这个平衡因子只能是-1、0或1。当这个条件不满足时,就需要进行旋转操作。具体来说,AVL树的旋转操作包括四种情况:
- 右旋(Right Rotation)
- 左旋(Left Rotation)
- 左-右双旋(Left-Right Rotation)
- 右-左双旋(Right-Left Rotation)
### 3.2.2 AVL树的平衡因子计算
在实现AVL树时,需要为每个节点维护一个平衡因子属性。这个属性在每
0
0
相关推荐
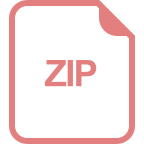
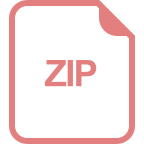
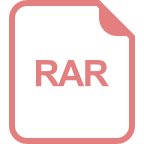
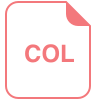
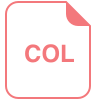


