内存管理大师:堆与栈的区别及内存分配机制深度解析
发布时间: 2024-09-10 18:03:11 阅读量: 98 订阅数: 42 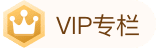
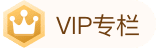
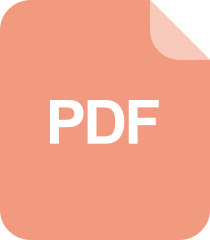
代码简化大师:C语言中的typedef关键字深度解析
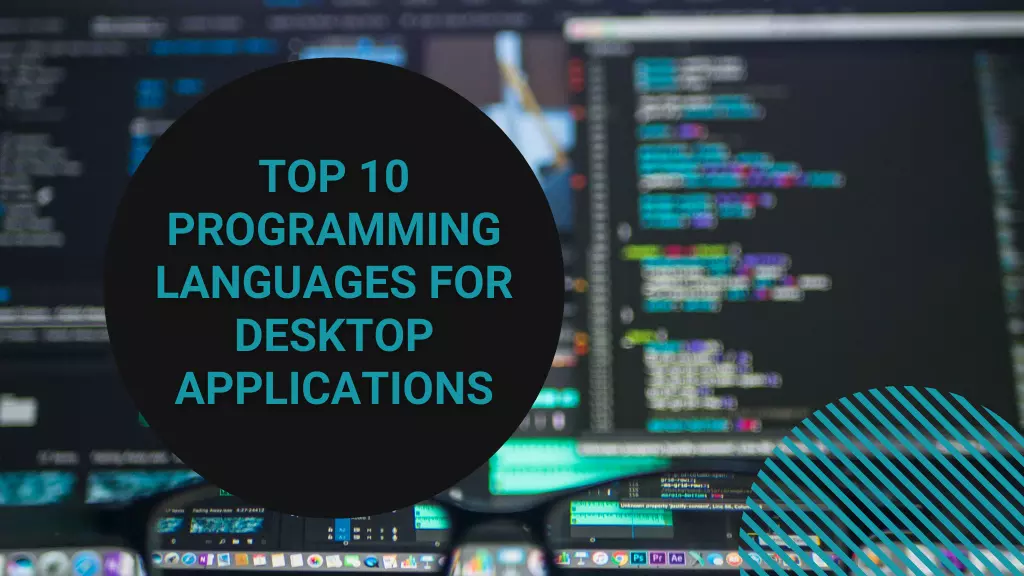
# 1. 堆与栈的基本概念
堆(Heap)和栈(Stack)是计算机内存管理中的两个核心概念,它们在数据结构和程序运行时起到了至关重要的作用。为了深入理解这两个概念,我们需要从其定义开始探讨,逐步深入到它们在程序执行中的实际作用和差异。
## 1.1 堆的概念
在计算机科学中,堆是一种特殊的树形数据结构。在内存管理中,堆是一个大块的内存空间,用来存放动态分配的对象。堆空间的分配是动态的,通常由程序员通过代码显式申请和释放。堆的特点是分配灵活,但伴随开销较大,因为需要在运行时确定内存大小和位置。
## 1.2 栈的概念
栈是一种后进先出(Last In, First Out, LIFO)的数据结构,常用于程序中变量的存储和函数调用时的环境记录。在内存管理中,栈是一种用于存储局部变量、返回地址、参数传递等信息的内存区域。栈的分配速度快,但其容量有限且分配规则严格。
通过下一章对内存管理角色的探讨,我们将进一步揭示堆与栈在内存分配中的不同策略和用途。
# 2. 堆与栈在内存管理中的角色
## 2.1 堆和栈的数据存储特性
### 2.1.1 栈的数据存储原理
在计算机科学中,栈是一种数据结构,用于存储临时变量,并且按照后进先出(LIFO)的顺序来访问。当一个程序执行时,CPU 会将函数调用压入栈中,并在函数执行完毕后释放这些调用栈空间。每个函数调用都会在栈上创建一个栈帧(Stack Frame),其中存储了函数的局部变量、返回地址和传递给函数的参数。
在x86架构的处理器中,栈空间通常由ESP(扩展栈指针寄存器)所指向,而EBP(扩展基指针寄存器)用于定位函数内的局部变量。当一个函数被调用时,其参数会从右到左压入栈中,然后控制权被转移到被调用的函数,该函数的栈帧通过EBP和ESP确定。函数执行完毕后,ESP会增加以释放栈帧空间,这样先前的栈帧就恢复为当前栈帧,控制权也返回到调用函数。
```assembly
push ebp ; 保存当前栈帧的基指针
mov ebp, esp ; 设置新的栈帧基指针
sub esp, 10h ; 分配空间给局部变量
; ... 函数代码 ...
mov esp, ebp ; 清除局部变量分配的空间
pop ebp ; 恢复先前的栈帧基指针
ret ; 返回到调用者
```
### 2.1.2 堆的数据存储原理
与栈不同,堆是一个动态的内存存储区域,通常用于存储程序运行时需要创建的对象。堆是由操作系统管理的内存池,其内存分配通常不受栈那样的限制,可以根据需要动态分配和释放。
堆内存分配机制允许程序员申请一块内存,并在不再需要时显式释放。这使得堆适合于存储生命周期不确定的数据,如动态数组和对象实例。然而,堆内存的分配和释放可能会导致内存碎片化问题,影响程序性能。
```c
int* array = (int*)malloc(10 * sizeof(int)); // 动态申请堆内存
if (array != NULL) {
// ... 使用内存 ...
free(array); // 释放堆内存
}
```
## 2.2 堆与栈的内存分配策略
### 2.2.1 静态内存分配
静态内存分配是程序编译时确定的内存分配方式。在C/C++等语言中,静态内存主要用于全局变量和静态局部变量。这些变量的生命周期从程序开始执行时一直持续到程序结束,存储在程序的静态数据区。静态分配的变量不需要动态管理内存,也不会有栈或堆中的问题,如内存碎片化或内存泄漏。
### 2.2.2 动态内存分配
动态内存分配是一种在程序运行时进行的内存分配方式。在C语言中,常见的动态内存分配函数有`malloc`、`calloc`、`realloc`和`free`,而在C++中则有`new`和`delete`操作符。动态分配的内存通常从堆中分配,程序员需要手动管理这些内存的生命周期。
### 2.2.3 内存分配的生命周期管理
在C语言中,内存分配后必须由程序员负责释放。如果内存分配后未释放或者提前释放,将导致内存泄漏或双重释放的问题。因此,良好的编程习惯是使用堆内存时确保每个`malloc`或`new`都有对应的`free`或`delete`。
在现代编程语言中,如Java和Python,垃圾回收机制自动管理内存,简化了内存分配的生命周期管理。然而,即使在这些语言中,了解底层的内存管理机制对于编写高效的代码依然至关重要。
```java
Object obj = new Object(); // 自动垃圾回收的语言中的内存分配
// ... 使用obj ...
// 不需要手动释放obj,垃圾回收器会自动处理
```
在下一章节中,我们将通过实际应用案例来深入探讨堆和栈的具体应用,并通过代码和逻辑分析来展示堆与栈在不同类型内存管理中的实际运用。
# 3. 堆与栈的实际应用案例分析
### 3.1 堆在大型数据处理中的应用
在处理大量数据时,堆内存的动态分配机制显得尤为关键。堆内存可以分配和回收大量的空间,用于存储大型数据结构,如集合、列表和对象等。
#### 3.1.1 堆在动态内存分配中的应用实例
动态内存分配是在程序运行时根据需要申请内存的过程,典型例子包括在C++中使用`new`关键字分配对象或数据结构:
```cpp
int main() {
// 使用堆分配一个动态数组
int* array = new int[100];
// 使用数组
for (int i = 0; i < 100; ++i) {
array[i] = i;
}
// 完成使用后释放堆内存
delete[] array;
return 0;
}
```
执行逻辑说明:在上述代码中,`new`用于在堆上分配一个整数数组,数组大小为100。在使用完毕后,必须通过`delete[]`来释放这块内存,以避免内存泄漏。
参数说明:`new`后面紧跟类型`int`和数组大小`[100]`,表示要分配的内存量。
动态内存分配的灵活性允许程序在运行时根据实际需求扩展或收缩内存使用,但这也要求程序员必须手动管理内存,确保每次`new`后都有对应的`delete`操作。
#### 3.1.2 堆内存溢出的预防和处理
堆内存溢出(也称为内存泄漏)是常见的内存管理问题,可能会导致程序性能下降甚至崩溃。预防堆内存溢出的方法包括:
- **智能指针**:在现代C++中,使用智能指针如`std::unique_ptr`和`std::shared_ptr`可以自动管理内存释放。
- **内存池**:创建内存池来预先分配大量内存块,并在需要时从中分配和回收,这样可以减少分配次数,提高效率。
- **垃圾回收**:在一些高级语言中,如Java或Python,有内置的垃圾回收机制,可以自动回收不再使用的内存。
### 3.2 栈在函数调用中的应用
栈内存通常用于函数调用过程中的局部变量存储,其优势在于能够自动管理内存,减少手动分配和释放的负担。
#### 3.2.1 栈在局部变量存储中的作用
局部变量在栈上创建,当函数调用发生时,会压栈;函数返回时,局部变量所占的空间会自动弹出,其生命周期被严格控制。
```c
void function() {
```
0
0
相关推荐
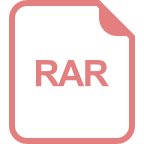
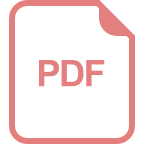
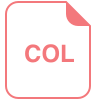
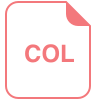
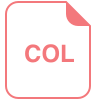
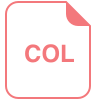
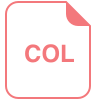
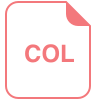