【基础】Python中的字符串处理与常用方法
发布时间: 2024-06-24 11:59:15 阅读量: 87 订阅数: 122 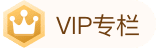
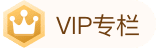
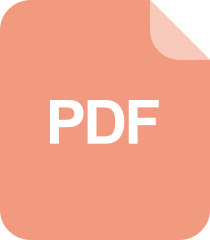
Python 基础知识之字符串处理
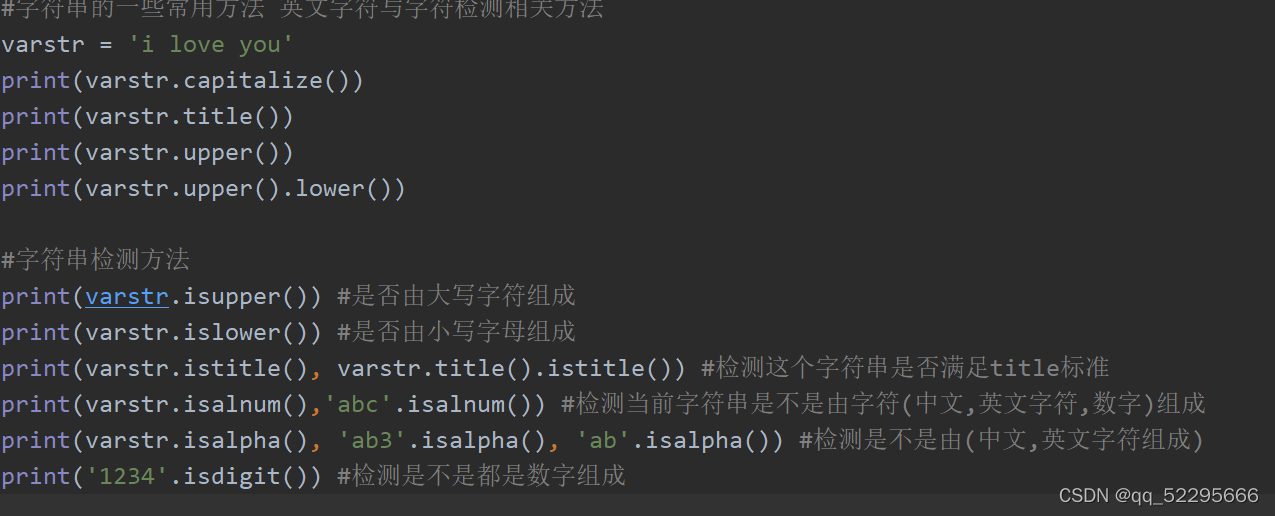
# 2.1 字符串的切片和连接
### 2.1.1 字符串切片的基本语法
字符串切片是一种提取字符串中特定部分的强大技术。它的语法如下:
```python
string[start:end:step]
```
其中:
* `start`:切片的起始索引(包括在内)
* `end`:切片的结束索引(不包括在内)
* `step`:切片步长,指定每次跳过多少个字符
例如,以下代码提取字符串 "Hello World" 中从索引 2 到索引 6(不包括)的字符:
```python
"Hello World"[2:6]
```
输出:
```
"llo "
```
# 2. Python字符串处理高级技巧
### 2.1 字符串的切片和连接
#### 2.1.1 字符串切片的基本语法
字符串切片是一种获取字符串中指定部分的方法,其语法如下:
```python
string[start:end:step]
```
其中:
* `start`:切片的起始索引(包括在内)
* `end`:切片的结束索引(不包括在内)
* `step`:切片的步长(默认为1)
例如:
```python
>>> s = "Hello World"
>>> s[0:5]
'Hello'
>>> s[2:7]
'llo W'
>>> s[::2]
'HloWrd'
```
#### 2.1.2 字符串连接的各种方法
字符串连接是指将多个字符串合并成一个新的字符串。Python提供了多种连接字符串的方法:
* **`+`运算符:**直接将字符串相加
* **`join()`方法:**将可迭代对象中的元素连接成一个字符串,并使用指定的分隔符
* **`format()`方法:**将格式化字符串与其他对象连接
例如:
```python
>>> s1 = "Hello"
>>> s2 = "World"
>>> s1 + s2
'HelloWorld'
>>> ', '.join(['a', 'b', 'c'])
'a, b, c'
>>> "My name is {} {}".format(s1, s2)
'My name is Hello World'
```
### 2.2 字符串的查找和替换
#### 2.2.1 字符串查找的常用函数
Python提供了多种查找字符串中特定子字符串的函数:
* **`find()`方法:**返回子字符串在字符串中首次出现的索引,如果没有找到则返回-1
* **`rfind()`方法:**返回子字符串在字符串中最后一次出现的索引,如果没有找到则返回-1
* **`index()`方法:**与`find()`方法类似,但如果子字符串不存在则抛出`ValueError`异常
* **`rindex()`方法:**与`rfind()`方法类似,但如果子字符串不存在则抛出`ValueError`异常
例如:
```python
>>> s = "Hello World"
>>> s.find("World")
6
>>> s.rfind("World")
6
>>> s.index("World")
6
```
#### 2.2.2 字符串替换的灵活运用
Python提供了两种替换字符串中特定子字符串的方法:
* **`replace()`方法:**将字符串中所有匹配的子字符串替换为指定的替换字符串
* **`translate()`方法:**将字符串中的字符映射到指定的替换字符
例如:
```python
>>> s = "Hello World"
>>> s.replace("World", "Universe")
'Hello Universe'
>>> s.translate({ord('o'): 'a', ord('l'): 'i'})
'Hailai Wairld'
```
### 2.3 字符串的格式化和转换
#### 2.3.1 字符串格式化的不同方式
Python提供了多种格式化字符串的方法:
* **`%`格式化:**使用`%`符号和格式说明符指定格式
* **`str.format()`方法:**使用大括号和格式说明符指定格式
* **`f-strings`:**使用`f`前缀和表达式指定格式
例如:
```python
>>> name = "John"
>>> age = 30
>>> "%s is %d years old" % (name, age)
'John is 30 years old'
>>> "My name is {name} and I am {age} years old".format(name=name, age=age)
'My name is John and I am 30 years old'
>>> f"My name is {name} and I am {age} years old"
'My name is John and I am 30 years old'
```
#### 2.3.2 字符串转换的常用函数
Python提供了多种转换字符串的方法:
* **`int()`函数:**将字符串转换为整数
* **`float()`函数:**将字符串转换为浮点数
* **`bool()`函数:**将字符串转换为布尔值
* **`str()`函数:**将
0
0
相关推荐






