递归实现复杂功能的策略与技巧:数据结构中的高效应用
发布时间: 2024-09-12 15:38:32 阅读量: 33 订阅数: 22 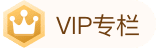
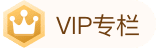
# 1. 递归的理论基础与数学原理
在计算机科学中,递归是一种重要的编程范式,它允许一个函数调用自身来解决问题。要掌握递归,首先要了解其数学原理和理论基础。递归的核心在于将一个大问题分解为一系列子问题,这些子问题在结构上与原问题相同,但规模更小。通过解决这些子问题,最终可得出原问题的解。
在数学上,递归关系定义了序列的每一项是如何从前面的一项或几项推导出来的。例如,斐波那契数列的定义就是一个典型的递归关系。理解这种数学上的递归关系是设计递归算法的关键。
递归在形式上可以表示为:
```
R(n) = base_case, for n < N
R(n) = g(R(n-1), R(n-2), ..., R(n-k)), for n >= N
```
其中 `base_case` 是递归的基本情况,当 `n` 小于某个特定值 `N` 时停止递归;`g` 是一个函数,它表示如何将问题的规模减小,并且可能涉及多个先前的状态 `R(n-1)`, `R(n-2)`, ..., `R(n-k)`。
递归的深度和性能分析,包括时间复杂度和空间复杂度,是递归理论中不可忽视的部分。理解这些理论将有助于我们设计出更高效的递归算法,并处理实际编程中可能遇到的性能问题,例如避免栈溢出。
递归理论基础的深入理解对于掌握后续章节关于递归算法设计、性能优化以及递归在数据结构和算法中的应用至关重要。在下一章中,我们将探讨如何将递归思维应用于算法设计中。
# 2. 递归算法的设计方法
## 2.1 递归思维与问题分解
递归是一种强大的编程技术,其核心在于将大问题分解为小问题,直至小问题可以直接解决。递归思维要求我们识别问题中的递归模式,即一个复杂问题可以分解为相似的子问题,而子问题的解决方式与原问题相同。
### 2.1.1 从问题到子问题的转化
在编写递归函数之前,我们必须能够识别出问题可以分解的规律。例如,在解决汉诺塔问题时,我们可以将三个盘子从A柱子移动到C柱子,看作是将前两个盘子先从A移动到B,然后将剩下的盘子移动到C,再将B上的两个盘子移动到C。每一个步骤都可以看作是一个子问题的解决。
```python
def hanoi(n, source, destination, auxiliary):
if n == 1:
print(f"Move disk 1 from {source} to {destination}")
return
hanoi(n-1, source, auxiliary, destination)
print(f"Move disk {n} from {source} to {destination}")
hanoi(n-1, auxiliary, destination, source)
# 参数说明:
# n: 盘子的数量
# source: 起始柱子
# destination: 目标柱子
# auxiliary: 辅助柱子
```
### 2.1.2 递归的终止条件与边界情况
递归必须有一个明确的终止条件,否则会无限递归下去。终止条件通常是子问题的最简单情况,例如汉诺塔问题中只有一个盘子时,直接将其从源柱子移动到目标柱子。
## 2.2 递归函数的编写技巧
递归函数的编写需要特别注意参数的传递和状态的保存,同时,分而治之的策略在递归编写中也尤为重要。
### 2.2.1 参数传递与状态保存
递归函数需要考虑的是如何传递参数来保存当前的状态,并且在每次递归调用时更新状态。在上面的汉诺塔例子中,我们通过函数参数`n`, `source`, `destination`, `auxiliary`来保存每个递归状态。
### 2.2.2 分而治之的策略应用
分而治之是一种通过将问题分成几个小部分来解决复杂问题的策略。在编写递归函数时,我们需要先确定如何将大问题分割成小问题,然后决定如何将小问题的解决方案组合成大问题的解决方案。
```mermaid
graph TD
A[复杂问题] -->|分割| B[子问题1]
A -->|分割| C[子问题2]
A -->|分割| D[子问题3]
B --> E[子问题1的解决方案]
C --> F[子问题2的解决方案]
D --> G[子问题3的解决方案]
E --> H[组合解决方案]
F --> H
G --> H[大问题的解决方案]
```
### 2.2.3 递归与迭代的比较
递归和迭代是解决问题的两种不同方法,通常可以通过递归或者迭代来实现同样的算法。然而,递归通常在代码的可读性上有优势,但可能会有更大的空间和时间开销,特别是当递归深度增加时,可能会引起栈溢出错误。而迭代则通常更节省空间,并且更容易控制资源使用。
## 2.3 递归性能分析与优化
递归虽然编程简单直观,但性能问题一直是其面临的挑战。在设计递归算法时,我们需要关注时间复杂度、空间复杂度以及潜在的优化机会。
### 2.3.1 时间复杂度与空间复杂度
递归算法的时间复杂度和空间复杂度分析相对直接。每个递归调用都会增加时间消耗,并且每个递归层次都需要额外的空间(栈空间)。在设计递归算法时,我们需要评估这些资源消耗是否在可接受的范围内。
### 2.3.2 递归深度与栈溢出的预防
递归深度是指在递归过程中递归函数调用的最大层数。如果递归层次过深,可能会导致栈溢出错误。为预防这种情况,我们可以使用尾递归优化或者限制递归的深度。
### 2.3.3 尾递归与内存优化技术
尾递归是一种特殊的递归形式,其中递归调用是函数体中的最后一个操作。许多现代编译器能够优化尾递归,从而减少栈空间的使用。要实现尾递归,函数必须返回直接的递归调用结果,而不是经过计算的结果。
通过以上的分析,我们了解了递归算法的设计方法。在下一章节中,我们将深入探讨递归在数据结构中的应用实例,包括树结构的递归遍历和图的递归搜索算法等。
0
0
相关推荐
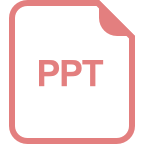
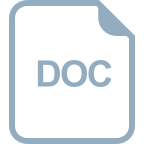
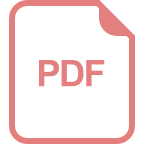
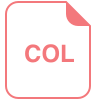
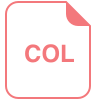
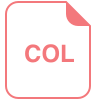
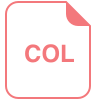

