ORB算法实战指南:图像匹配与物体识别的秘诀
发布时间: 2024-08-14 18:13:27 阅读量: 13 订阅数: 15 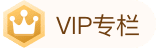
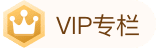
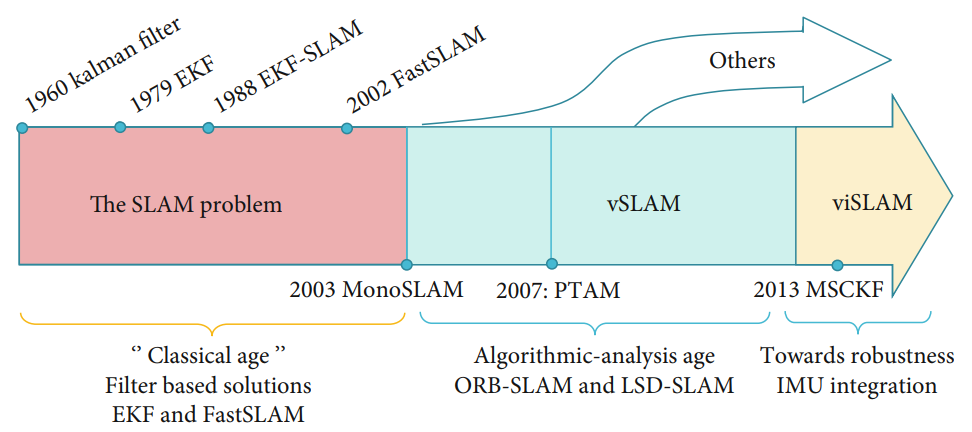
# 1. ORB算法理论基础
ORB(Oriented FAST and Rotated BRIEF)算法是一种高效的特征点检测和描述算法,广泛应用于图像匹配和物体识别领域。本节将介绍ORB算法的理论基础,包括其关键步骤和数学原理。
ORB算法的核心思想是将FAST角点检测器与BRIEF描述子相结合。FAST算法通过比较像素灰度值来快速检测角点,而BRIEF算法则利用二进制模式生成描述子,具有较强的鲁棒性和计算效率。ORB算法通过将BRIEF描述子与FAST角点关联,获得了既具有鲁棒性又高效的特征点描述能力。
# 2. ORB算法实现与优化
ORB算法的实现涉及特征提取、匹配和优化三个主要步骤。本节将详细介绍ORB算法的实现细节,并探讨优化策略以提高算法的性能。
### 2.1 ORB算法的特征提取
ORB算法的特征提取包括FAST角点检测和BRIEF描述子生成两个步骤。
#### 2.1.1 FAST角点检测
FAST(Features from Accelerated Segment Test)算法是一种快速、高效的角点检测算法。它通过比较图像像素的强度值来识别角点。具体步骤如下:
```python
def FAST(image, threshold):
"""
FAST角点检测算法
参数:
image: 输入图像
threshold: 角点阈值
返回:
角点列表
"""
corners = []
for x in range(1, image.shape[0] - 1):
for y in range(1, image.shape[1] - 1):
# 检查16个像素的强度值
intensities = [image[x + i, y + j] for i, j in [(0, -3), (1, -3), (2, -2), (3, -1),
(3, 0), (3, 1), (2, 2), (1, 3),
(0, 3), (-1, 3), (-2, 2), (-3, 1),
(-3, 0), (-3, -1), (-2, -2), (-1, -3)]]
# 至少有12个像素的强度值与中心像素的差值大于阈值
if sum(map(lambda x: abs(x - image[x, y]) > threshold, intensities)) >= 12:
corners.append((x, y))
return corners
```
**代码逻辑逐行解读:**
* 遍历图像中每个像素(除边界像素外)。
* 对于每个像素,检查其周围16个像素的强度值。
* 如果至少有12个像素的强度值与中心像素的差值大于阈值,则该像素被标记为角点。
#### 2.1.2 BRIEF描述子生成
BRIEF(Binary Robust Independent Elementary Features)描述子是一种二进制描述子,它通过比较图像像素的强度值来生成一组二进制位。具体步骤如下:
```python
def BRIEF(image, keypoints, patch_size):
"""
BRIEF描述子生成算法
参数:
image: 输入图像
keypoints: 角点列表
patch_size: 描述子补丁大小
返回:
描述子矩阵
"""
descriptors = np.zeros((len(keypoints), patch_size ** 2), dtype=np.uint8)
for i, keypoint in enumerate(keypoints):
x, y = keypoint
patch = image[x - patch_size // 2:x + patch_size // 2 + 1, y - patch_size // 2:y + patch_size // 2 + 1]
for j in range(patch_size ** 2):
row, col = j // patch_size, j % patch_size
descriptors[i, j] = (patch[row, col] > patch[row, col + 1])
return descriptors
```
**代码逻辑逐行解读:**
* 遍历角点列表。
* 对于每个角点,提取其周围一个正方形补丁。
* 对于补丁中的每个像素,将其与相邻像素的强度值进行比较,并生成一个二进制位。
* 将所有二进制位连接起来形成一个描述子。
### 2.2 ORB算法的匹配和优化
ORB算法的匹配和优化包括暴力匹配、近似最近邻匹配和匹配优化策略。
#### 2.2.1 暴力匹配
暴力匹配是一种简单但耗时的匹配策略,它遍历所有可能的图像对并计算它们的距离。
```python
def brute_force_match(descriptors1, descriptors2, threshold):
"""
暴力匹配算法
参数:
descriptors1: 第一张图像的描述子
descriptors2: 第二张图像的描述子
threshold: 匹配阈值
返回:
匹配点对列表
"""
matches = []
for i in range(descriptors1.shape[0]):
for j in range(descriptors2.shape[0]):
if np.linalg.norm(descriptors1[i] - descriptors2[j]) < threshold:
matches.append((i, j))
return matches
```
**代码逻辑逐行解读:**
* 遍历第一张图像中的所有描述子。
* 对于每个描述子,遍历第二张图像中的所有描述子。
* 如果两个描述子之间的距离小于阈值,则将它们匹配起来。
#### 2.2.2 近似最近邻匹配
近似最近邻匹配(ANN)是一种更有效的匹配策略,它使用数据结构(如KD树)来快速查找最近的邻域。
```python
def ANN_match(descriptors1, descriptors2, threshold):
"""
近似最近邻匹配算法
参数:
descriptors1: 第一张图像的描述子
descriptors2: 第二张图像的描述子
threshold: 匹配阈值
返回:
匹配点对列表
"""
tree = KDTree(descriptors2)
matches = []
for i in range(descriptors1.shape[0]):
idx, dist = tree.query(descriptors1[i], k=1)
if dist < threshold:
matches.append((i, idx))
return matches
```
**代码逻辑逐行解读:**
* 使用KD树构建第二张图像描述子的索引。
* 遍历第一张图像中的所有描述子。
* 对于每个描述子,使用KD树查找其最近邻域。
* 如果最近邻域的距离小于阈值,则将它们匹配起来。
#### 2.2.3 匹配优化策略
匹配优化策略可以进一步提高匹配的准确性。常用的策略包括:
* **RANSAC(Random Sample Consensus):**从匹配点对中随机抽取子集,并计算模型参数。如果模型参数满足一定条件,则认为子集中的匹配点对是正确的。
* **局部相似性度量:**检查匹配点对周围的区域,如果区域内有足够的相似点对,则认为匹配点对是正确的。
* **几何一致性检查:**根据匹配点对的几何关系,检查它们是否符合透视或仿射变换模型。
# 3.1 图像拼接
图像拼接是将多幅图像组合成一幅全景图像的过程。ORB算法在图像拼接中发挥着重要作用,因为它可以快速准确地提取和匹配图像特征点。
#### 3.1.1 图像配准
图像配准是图像拼接的关键步骤,其目的是将多幅图像对齐到同一个坐标系中。ORB算法可以通过提取和匹配特征点来实现图像配准。
```python
import cv2
# 加载图像
image1 = cv2.imread('image1.jpg')
image2 = cv2.imread('image2.jpg')
# ORB特征提取
orb = cv2.ORB_create()
kp1, des1 = orb.detectAndCompute(image1, None)
kp2, des2 = orb.detectAndCompute(image2, None)
# 特征点匹配
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
matches = bf.match(des1, des2)
# 获取匹配点坐标
points1 = np.array([kp1[m.queryIdx].pt for m in matches])
points2 = np.array([kp2[m.trainIdx].pt for m in matches])
# 计算仿射变换矩阵
H, _ = cv2.findHomography(points1, points2, cv2.RANSAC, 5.0)
# 图像配准
image2_aligned = cv2.warpPerspective(image2, H, (image1.shape[1] + image2.shape[1], image1.shape[0]))
```
上述代码展示了如何使用ORB算法进行图像配准。首先,使用ORB提取器提取图像特征点和描述子。然后,使用暴力匹配器匹配两幅图像中的特征点。最后,使用RANSAC算法计算仿射变换矩阵,并使用该矩阵对第二幅图像进行配准。
#### 3.1.2 图像融合
图像融合是将多幅配准后的图像融合成一幅全景图像的过程。ORB算法可以帮助确定图像之间的重叠区域,并指导图像融合过程。
```python
import cv2
# 加载配准后的图像
image1_aligned = cv2.imread('image1_aligned.jpg')
image2_aligned = cv2.imread('image2_aligned.jpg')
# 图像融合
stitcher = cv2.Stitcher_create()
(status, stitched) = stitcher.stitch([image1_aligned, image2_aligned])
# 检查融合状态
if status == cv2.STITCHER_OK:
cv2.imwrite('panorama.jpg', stitched)
print('图像拼接成功')
else:
print('图像拼接失败')
```
上述代码展示了如何使用ORB算法辅助图像融合。首先,加载配准后的图像。然后,使用Stitcher类进行图像融合。最后,检查融合状态并保存拼接后的全景图像。
# 4. ORB算法在物体识别中的实践
### 4.1 基于ORB算法的物体识别系统设计
#### 4.1.1 系统架构
基于ORB算法的物体识别系统通常采用分层架构,包括以下主要模块:
- **图像预处理:**对输入图像进行预处理,包括图像缩放、灰度转换、噪声去除等。
- **特征提取:**使用ORB算法提取图像中的特征点和描述子。
- **特征匹配:**将提取的特征点与训练集中的特征点进行匹配,找到具有相似特征的图像。
- **物体识别:**根据匹配结果,对物体进行识别和分类。
#### 4.1.2 算法选择
在物体识别系统中,ORB算法的选用主要基于以下优势:
- **鲁棒性强:**ORB算法对图像旋转、缩放、光照变化等因素具有较强的鲁棒性。
- **计算效率高:**ORB算法的特征提取和匹配过程相对高效,可以满足实时性要求。
- **描述子区分度高:**ORB算法的BRIEF描述子具有较高的区分度,可以有效区分不同物体。
### 4.2 基于ORB算法的物体识别系统实现
#### 4.2.1 数据集准备
为了训练和评估物体识别系统,需要准备一个包含不同物体图像的数据集。数据集应包含各种光照条件、背景复杂度和物体姿态的图像。
#### 4.2.2 模型训练和评估
**模型训练:**
1. 使用ORB算法从训练集图像中提取特征点和描述子。
2. 将提取的特征点和描述子聚类,形成视觉词典。
3. 使用支持向量机(SVM)或其他分类算法,基于视觉词典训练物体识别模型。
**模型评估:**
1. 使用测试集图像对训练好的模型进行评估。
2. 计算模型的准确率、召回率、F1分数等指标。
3. 根据评估结果,优化模型参数或选择不同的算法。
```python
# 导入必要的库
import cv2
import numpy as np
from sklearn.svm import SVC
# 加载训练集图像
images = []
for image_path in training_image_paths:
image = cv2.imread(image_path)
images.append(image)
# 使用ORB算法提取特征点和描述子
orb = cv2.ORB_create()
keypoints = []
descriptors = []
for image in images:
kp, des = orb.detectAndCompute(image, None)
keypoints.append(kp)
descriptors.append(des)
# 聚类形成视觉词典
kmeans = cv2.BOWKMeansTrainer(100)
kmeans.add(descriptors)
visual_dictionary = kmeans.cluster()
# 使用SVM训练物体识别模型
svm = SVC()
svm.fit(np.array(descriptors), np.array(labels))
```
# 5. ORB算法的扩展与展望
### 5.1 ORB算法的变体
ORB算法自提出以来,研究人员对其进行了大量的扩展和改进,衍生出多种变体,以满足不同应用场景的需求。
**5.1.1 ORB-SLAM**
ORB-SLAM(Simultaneous Localization and Mapping)是基于ORB算法的视觉SLAM(Simultaneous Localization and Mapping)系统。它利用ORB特征点进行实时定位和建图,具有鲁棒性高、计算效率快等优点。ORB-SLAM在移动机器人、无人机等领域得到了广泛应用。
**5.1.2 ORB2**
ORB2是ORB算法的升级版本,它在以下方面进行了改进:
- **特征点提取:**ORB2采用了新的FAST角点检测算法,提高了特征点的鲁棒性和重复性。
- **描述子生成:**ORB2使用了一种新的二进制描述子,称为BRISK(Binary Robust Invariant Scalable Keypoints),它具有更高的区分能力和抗噪性。
- **匹配策略:**ORB2采用了新的匹配策略,结合了暴力匹配和近似最近邻匹配,提高了匹配精度和效率。
### 5.2 ORB算法的未来发展方向
随着计算机视觉技术的不断发展,ORB算法也在不断演进,未来主要的发展方向包括:
**5.2.1 算法精度提升**
提高ORB算法的特征提取和匹配精度是未来研究的重点。这可以通过改进角点检测算法、描述子生成方法和匹配策略来实现。
**5.2.2 实时性优化**
ORB算法的实时性对于某些应用场景至关重要。未来将重点研究如何优化ORB算法的计算效率,使其能够在更低功耗的设备上实时运行。
0
0
相关推荐
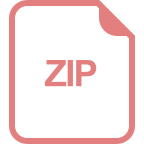
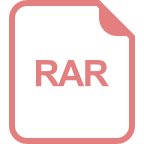
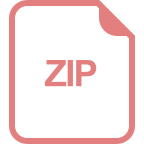
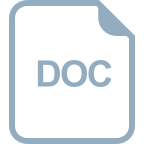
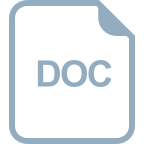
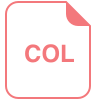
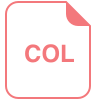
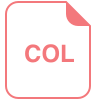
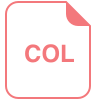