动态规划与数据结构大解析:分析算法与数据结构的相互作用
发布时间: 2024-08-24 14:25:13 阅读量: 22 订阅数: 34 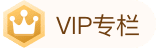
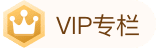
# 1. 动态规划简介
动态规划是一种解决复杂问题的算法技术,它将问题分解为更小的子问题,并通过存储和重用子问题的解决方案来避免重复计算。动态规划算法适用于具有重叠子问题和最优子结构这两个特性的问题。
动态规划算法的基本原理是将问题分解为一系列重叠的子问题,并为每个子问题计算一个最优解。这些最优解存储在一个表中,以便在需要时可以快速访问。通过重用子问题的最优解,动态规划算法可以避免重复计算,从而提高算法的效率。
# 2. 动态规划算法理论基础
### 2.1 动态规划的基本原理
动态规划是一种自底向上的算法设计方法,它将复杂问题分解成一系列较小的子问题,并通过逐步求解这些子问题来解决原问题。其基本原理如下:
1. **子问题重叠:**问题可以分解成多个子问题,并且这些子问题之间存在重叠。
2. **最优子结构:**子问题的最优解可以由其子问题的最优解组合而成。
3. **记忆化:**对已经求解过的子问题进行存储,避免重复计算。
### 2.2 动态规划算法的类型
动态规划算法根据子问题的求解方式分为两类:
1. **自顶向下:**从问题根节点出发,递归求解子问题,并在求解过程中将子问题的解进行存储。
2. **自底向上:**从子问题的最底层开始,逐步求解子问题,并存储子问题的解。
### 2.3 动态规划算法的复杂度分析
动态规划算法的复杂度取决于子问题的数量和求解每个子问题的复杂度。一般情况下,动态规划算法的时间复杂度为 O(n^k),其中 n 为问题规模,k 为子问题的数量。
```
// 动态规划算法复杂度分析示例
// 斐波那契数列的动态规划算法
int fib(int n) {
if (n <= 1) {
return n;
}
int[] memo = new int[n + 1];
return fib(n - 1, memo) + fib(n - 2, memo);
}
int fib(int n, int[] memo) {
if (n <= 1) {
return n;
}
if (memo[n] != 0) {
return memo[n];
}
memo[n] = fib(n - 1, memo) + fib(n - 2, memo);
return memo[n];
}
// 复杂度分析
// 子问题的数量:n
// 求解每个子问题的复杂度:O(1)
// 因此,总的时间复杂度为 O(n)
```
# 3.1 背包问题
背包问题是一个经典的动态规划问题,它涉及在给定的容量限制下,从一组物品中选择最大价值的子集。
#### 问题描述
给定一个背包容量为 `W`,和 `n` 件物品,每件物品有重量 `w[i]` 和价值 `v[i]`。背包问题是找到一个物品子集,使得它们的总重量不超过 `W`,并且总价值最大。
#### 动态规划算法
背包问题的动态规划算法基于以下状态转移方程:
```python
dp[i][j] = max(dp[i-1][j], dp[i-1][j-w[i]] + v[
```
0
0
相关推荐








