矩阵运算在数据科学中的广泛应用:从数据分析到机器学习
发布时间: 2024-07-10 08:39:02 阅读量: 50 订阅数: 22 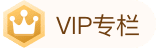
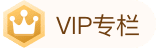
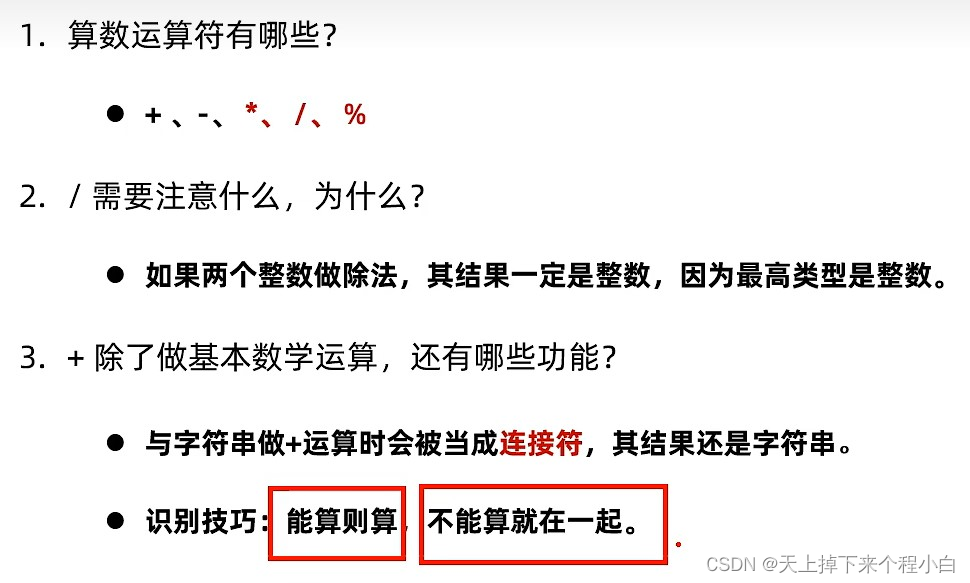
# 1. 矩阵运算在数据科学中的基础
矩阵运算在数据科学中扮演着至关重要的角色,它为数据分析、机器学习和深度学习等任务提供了强大的数学基础。矩阵是一种矩形数组,其元素可以是数字、符号或其他数学对象。矩阵运算涉及对这些元素进行各种操作,如加法、减法、乘法和求逆。
矩阵运算在数据科学中的应用广泛,包括:
- 数据预处理和特征工程:矩阵运算用于数据归一化、标准化、特征选择和降维。
- 数据探索和可视化:矩阵运算用于主成分分析(PCA)和奇异值分解(SVD),以帮助探索数据并创建有意义的可视化。
# 2. 矩阵运算在数据分析中的应用
### 2.1 数据预处理和特征工程
**2.1.1 数据归一化和标准化**
数据归一化和标准化是数据预处理中常用的技术,用于将不同范围和单位的数据映射到一个统一的范围,消除数据之间的量纲差异。
**归一化**将数据映射到[0, 1]范围内,公式为:
```python
normalized_data = (data - min(data)) / (max(data) - min(data))
```
**标准化**将数据映射到均值为0、标准差为1的范围内,公式为:
```python
standardized_data = (data - mean(data)) / std(data)
```
**代码逻辑分析:**
* `min(data)`和`max(data)`分别计算数据的最小值和最大值。
* `mean(data)`计算数据的均值。
* `std(data)`计算数据的标准差。
**参数说明:**
* `data`:需要归一化或标准化的数据。
**2.1.2 特征选择和降维**
特征选择和降维是数据分析中常用的技术,用于选择对模型训练有用的特征并减少数据的维度。
**特征选择**通过评估特征与目标变量之间的相关性来选择最相关的特征。常用的特征选择方法包括:
* **过滤法:**根据特征的统计量(如相关系数、信息增益)进行特征选择。
* **包裹法:**通过训练多个模型来选择特征子集,以最大化模型性能。
* **嵌入法:**在模型训练过程中同时进行特征选择。
**降维**通过将高维数据投影到低维空间来减少数据的维度。常用的降维方法包括:
* **主成分分析(PCA):**将数据投影到方差最大的方向上。
* **奇异值分解(SVD):**将数据分解为奇异值、左奇异向量和右奇异向量的乘积。
**代码逻辑分析:**
* **特征选择:**使用`SelectKBest`或`SelectFromModel`等特征选择器来选择特征。
* **降维:**使用`PCA`或`SVD`等降维算法来投影数据。
**参数说明:**
* `data`:需要进行特征选择或降维的数据。
* `k`:特征选择中要选择的特征数量。
* `n_components`:降维中要投影到的维度数量。
### 2.2 数据探索和可视化
**2.2.1 主成分分析(PCA)**
PCA是一种降维技术,通过将数据投影到方差最大的方向上,可以有效地减少数据的维度并保留主要信息。
**代码逻辑分析:**
```python
pca = PCA(n_components=2)
pca.fit(data)
```
* `PCA(n_components=2)`创建一个PCA对象,指定投影到2维空间。
* `fit(data)`将数据拟合到PCA模型中。
**参数说明:**
* `data`:需要进行PCA的数据。
* `n_components`:投影到的维度数量。
**2.2.2 奇异值分解(SVD)**
SVD是一种降维技术,将数据分解为奇异值、左奇异向量和右奇异向量的乘积。SVD可以用于数据降维、特征提取和图像处理。
**代码逻辑分析:**
```python
u, s, vh = np.linalg.svd(data, full_matrices=False)
```
* `np.linalg.svd(data, full_matrices=False)`对数据进行SVD分解。
* `u`:左奇异向量。
* `s`:奇异值。
* `vh`:右奇异向量。
**参数说明:**
* `data`:需要进行SVD分解的数据。
* `full_matrices`:指定是否返回完整的奇异值矩阵。
# 3.1 线性回归和逻辑回归
#### 3.1.1 矩阵运算在模型训练中的作用
**线性回归**
线性回归是一种监督学习算法,用于预测连续值的目标变量。其模型方程为:
```python
y = w0 + w1 * x1 + w2 * x2 + ... + wn * xn
```
其中:
* y 是目标变量
* x1, x2, ..., xn 是自变量
* w0, w1, ..., wn 是模型权重
在矩阵运算中,线性回归模型可以表示为:
```python
y = X * w
```
其中:
* X 是一个 m x n 的矩阵,其中 m 是样本数量,n 是自变量数量
* w 是一个 n x 1 的权重向量
通过矩阵运算,我们可以使用最小二乘法来估计模型权重:
```python
w = (X^T * X)^-1 * X^T * y
```
**逻辑回归**
逻辑回归是一种监督学习算法,用于预测二分类的目标变量。其模型方程为:
```python
p = 1 / (1 + exp(-(w0 + w1 * x1 + w2 * x2 + ... + wn * xn)))
```
其中:
* p 是目标变量的概率
* x1, x2, ..., xn 是自变量
* w0, w1, ..., wn 是模型权重
在矩阵运算中,逻辑回归模型可以表示为:
```python
p = 1 / (1 + exp(-X * w))
```
其中:
* X 是一个 m x n 的矩阵,其中 m 是样本数量,n 是自变量数量
* w 是一个 n x 1 的权重向量
通过矩阵运算,我们可以使用极大似然估计来估计模型权重:
```python
w = (X^T * X)^-1 * X^T * log(y / (1 - y))
```
#### 3.1.2 矩阵运算在模型评估中的应用
**均方误差 (MSE)**
MSE 是衡量回归模型预测值与真实值之间差异的指标。其计算公式为:
```python
MSE = 1 / m * Σ(y_pred - y)^2
```
其中:
* y_pred 是预测值
* y 是真实值
0
0
相关推荐
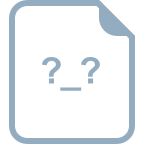
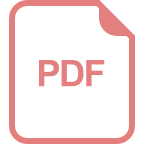
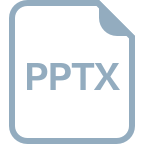
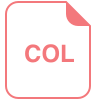
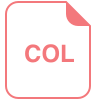
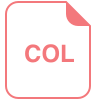
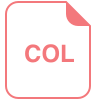

