矩阵运算在计算机图形学中的重要性:构建虚拟世界的数学基石
发布时间: 2024-07-10 08:36:22 阅读量: 88 订阅数: 42 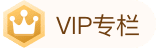
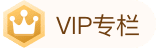
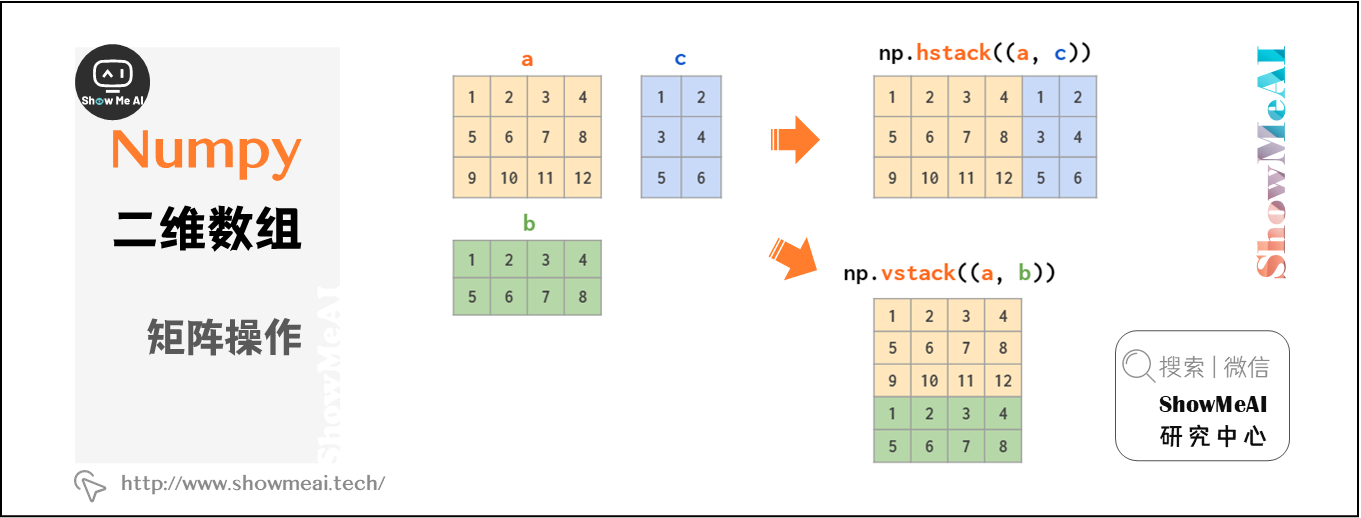
# 1. 矩阵运算基础
矩阵运算在计算机图形学中扮演着至关重要的角色,它提供了对几何图形进行变换、投影和视口转换的数学基础。本章将介绍矩阵运算的基本概念,包括矩阵的定义、运算和性质。
矩阵是一种矩形数组,由元素排列而成。矩阵运算涉及对这些元素进行各种操作,例如加法、减法、乘法和转置。矩阵的性质,例如可逆性和行列式,对于理解矩阵运算在计算机图形学中的应用至关重要。
# 2. 矩阵运算在计算机图形学中的应用
### 2.1 几何变换
几何变换是计算机图形学中对物体进行操作和转换的基本技术。通过矩阵运算,我们可以对物体进行平移、旋转和缩放等变换,从而实现各种视觉效果。
#### 2.1.1 平移变换
平移变换将物体沿指定方向移动一定距离。其变换矩阵如下:
```
T = [1 0 0 Tx]
[0 1 0 Ty]
[0 0 1 Tz]
[0 0 0 1]
```
其中,`Tx`、`Ty`、`Tz` 分别表示沿 x、y、z 轴的平移距离。
**代码块:**
```python
import numpy as np
# 定义平移向量
Tx = 10
Ty = 20
Tz = 30
# 构建平移矩阵
T = np.array([[1, 0, 0, Tx],
[0, 1, 0, Ty],
[0, 0, 1, Tz],
[0, 0, 0, 1]])
# 应用平移变换
vertices = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
transformed_vertices = np.dot(T, vertices.T).T
# 输出变换后的顶点坐标
print(transformed_vertices)
```
**逻辑分析:**
* `numpy.dot()` 函数用于执行矩阵乘法。
* `vertices.T` 将顶点坐标从行向量转置为列向量,以便与平移矩阵相乘。
* `transformed_vertices` 存储了变换后的顶点坐标。
#### 2.1.2 旋转变换
旋转变换将物体绕指定轴旋转一定角度。其变换矩阵如下:
**绕 x 轴旋转:**
```
Rx = [1 0 0 0]
[0 cos(theta) -sin(theta) 0]
[0 sin(theta) cos(theta) 0]
[0 0 0 1]
```
**绕 y 轴旋转:**
```
Ry = [cos(theta) 0 sin(theta) 0]
[0 1 0 0]
[-sin(theta) 0 cos(theta) 0]
[0 0 0 1]
```
**绕 z 轴旋转:**
```
Rz = [cos(theta) -sin(theta) 0 0]
[sin(theta) cos(theta) 0 0]
[0 0 1 0]
[0 0 0 1]
```
其中,`theta` 表示旋转角度。
**代码块:**
```python
import numpy as np
import math
# 定义旋转角度
theta_x = math.radians(30)
theta_y = math.radians(45)
theta_z = math.radians(60)
# 构建旋转矩阵
Rx = np.array([[1, 0, 0, 0],
[0, np.cos(theta_x), -np.sin(theta_x), 0],
[0, np.sin(theta_x), np.cos(theta_x), 0],
[0, 0, 0, 1]])
Ry = np.array([[np.cos(theta_y), 0, np.sin(theta_y), 0],
[0, 1, 0, 0],
[-np.sin(theta_y), 0, np.cos(theta_y), 0],
[0, 0, 0, 1]])
Rz = np.array([[np.cos(theta_z), -np.sin(theta_z), 0, 0],
[np.sin(theta_z), np.cos(theta_z), 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1]])
# 应用旋转变换
vertices = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
transformed_vertices = np.dot(Rz, np.dot(Ry, np.dot(Rx, vertices.T))).T
# 输出变换后的顶点坐标
print(transformed_vertices)
```
**逻辑分析:**
* `math.radians()` 函数将角度从度数转换为弧度。
* 旋转矩阵按照绕 z 轴、y 轴、x 轴的顺序相乘,实现复合旋转。
* `transformed_vertices` 存储了变换后的顶点坐标。
#### 2.1.3 缩放变换
缩放变换将物体沿指定方向放大或缩小。其变换矩阵如下:
```
S = [Sx 0 0 0]
[0 Sy 0 0]
```
0
0
相关推荐
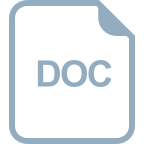
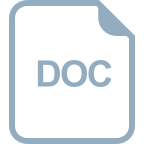
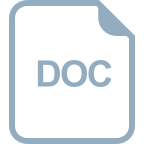
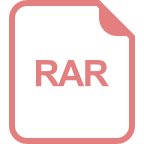
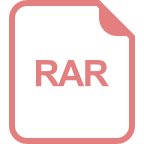
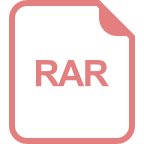
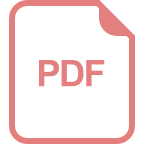
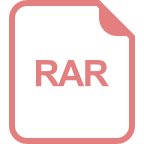
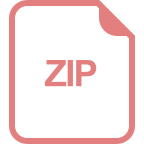