Java中的砖墙算法:算法分析与时间复杂度,深入理解算法本质
发布时间: 2024-08-28 09:07:05 阅读量: 14 订阅数: 12 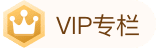
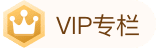
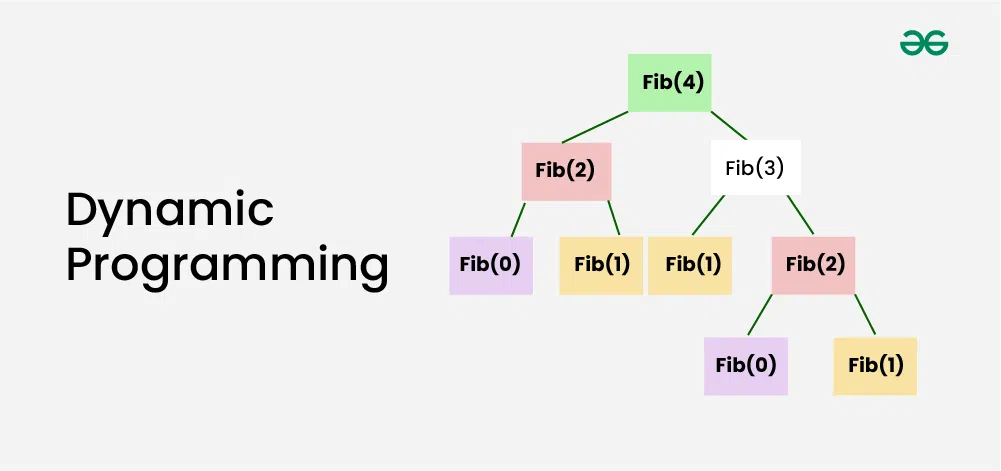
# 1. Java中的砖墙算法概述**
砖墙算法是一种用于解决计算机视觉和图像处理中图像分割问题的经典算法。它通过将图像划分为一系列垂直线段(砖块)来分割图像。算法的目标是找到一组垂直线段,使得这些线段将图像分割成尽可能多的连通区域。
砖墙算法是一种贪心算法,它从图像的左侧开始,逐个像素地扫描图像。对于每个像素,算法检查它是否位于现有线段的右侧。如果是,则算法将该像素添加到现有线段。如果不是,则算法创建一个新的线段并将其添加到线段列表中。
# 2. 砖墙算法的理论基础
### 2.1 算法原理和基本概念
砖墙算法是一种用于计算一堵砖墙中空隙的算法。它基于这样一个原理:如果一堵砖墙的每一行都有相同数量的砖块,那么墙中空隙的数量就是砖块总数减去砖块行数。
**基本概念:**
* **砖块:**构成砖墙的基本单元。
* **砖块行:**砖块水平排列形成的一行。
* **空隙:**砖块行之间的垂直空间。
### 2.2 算法的数学分析和时间复杂度
**数学分析:**
令:
* n 为砖块总数
* m 为砖块行数
则墙中空隙数量为:
```
空隙数量 = n - m
```
**时间复杂度:**
砖墙算法的时间复杂度为 O(n),因为算法需要遍历所有 n 个砖块。
**代码块:**
```java
public static int countGaps(int[][] wall) {
int n = wall.length; // 砖块总数
int m = wall[0].length; // 砖块行数
int gaps = n - m; // 空隙数量
return gaps;
}
```
**代码逻辑分析:**
* 遍历墙中的每一行,计算砖块总数 n。
* 取第一行的砖块数量作为砖块行数 m。
* 计算空隙数量 gaps。
* 返回 gaps。
**参数说明:**
* `wall`:代表砖墙的二维数组,其中每个元素表示一个砖块。
# 3. 砖墙算法的实践实现
### 3.1 算法的Java代码实现
以下代码段展示了砖墙算法的Java代码实现:
```java
import java.util.HashMap;
import java.util.Map;
public class BrickWall {
public int leastBricks(List<List<Integer>> wall) {
Map<Integer, Integer> map = new HashMap<>();
for (List<Integer> row : wall) {
int sum = 0;
for (int i = 0; i < row.size() - 1; i++) {
sum += row.get(i);
map.put(sum, map.getOrDefault(sum, 0) + 1);
}
}
int maxCount = 0;
for (int key : map.keySet()) {
maxCount = Math.max(maxCount, map.get(key));
}
return wall.size() - maxCount;
}
}
```
### 3.2 代码的详细分析和注释
**代码块 1:**
```java
Map<Integer, Integer> map = new HashMap<>();
```
- 初始化一个哈希表`map`,用于存储砖墙的断点和断点出现的次数。
**代码块 2:**
```java
for (List<Integer> row : wall) {
int sum = 0;
for (int i = 0; i < row.size() - 1; i++) {
sum += row.get(i);
map.put(sum, map.getOrDefault(sum, 0) + 1);
}
}
```
- 遍历每一行砖墙,计算每行断点的和。
- 将断点和作为哈希表的键,断点出现的次数作为值,存入`map`中。
**代码块 3:**
```java
int maxCount = 0;
for (int key : map.keySet()) {
maxCount = Math.max(maxCount, map.get(key));
}
```
- 遍历哈希表中的所有键,找出断点出现次数最多的键,并将其对应的值存储在`maxCount`中。
**代码块 4:**
```java
return wall.size() - maxCount;
```
- 返回砖墙总行数减去断点出现次数最多的断点的次数,即最少需要切断的砖块数量。
**参数说明:**
- `wall`:砖墙的表示,是一个二维列表,其中每一行代表一行砖墙,每一列代表一块砖的长度。
**代码逻辑分析:**
该算法采用哈希表来记录砖墙断点的出现次数。它遍历每一行砖墙,计算每行的断点和,并将断点和作为哈希表的键,断点出现的次数作为值,存入哈希表中。然后,它找到断点出现次数最多的键,并将其对应的值存储在`maxCount`中。最后,它返回砖墙总行数减去断点出现次数最多的断点的次数,即最少需要切断的砖块数量。
# 4. 砖墙算法的性能优化
### 4.1 算法的瓶颈分析和优化策略
在实际应用中,砖墙
0
0
相关推荐
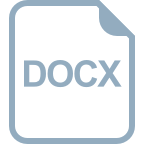
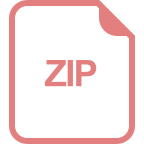
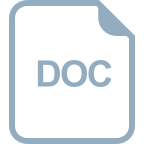





