openmv分别识别到红蓝白圆形图案并分别返回0,1,2的代码
时间: 2023-08-31 14:28:50 浏览: 46
### 回答1:
以下是使用OpenMV识别红蓝白圆形图案并返回0、1、2的代码示例:
```python
import sensor, image, time
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
# 设置圆形检测参数
red_threshold = (50, 70, 30, 80, 10, 70)
blue_threshold = (10, 50, -40, -10, -50, -10)
white_threshold = (70, 100, -10, 10, -10, 10)
min_radius = 20
max_radius = 100
while(True):
img = sensor.snapshot()
# 检测红色圆形
circles = img.find_circles(threshold = 5000, x_margin = 20, y_margin = 20, r_margin = 20, r_min = min_radius, r_max = max_radius, roi = (0, 0, img.width(), img.height()), robust = True, r_step = 2)
if circles:
img.draw_circle(circles[0].x(), circles[0].y(), circles[0].r(), color = (255, 0, 0))
print("红色圆形检测到,返回0")
# 检测蓝色圆形
circles = img.find_circles(threshold = 5000, x_margin = 20, y_margin = 20, r_margin = 20, r_min = min_radius, r_max = max_radius, roi = (0, 0, img.width(), img.height()), robust = True, r_step = 2, threshold_cb=lambda x: blue_threshold)
if circles:
img.draw_circle(circles[0].x(), circles[0].y(), circles[0].r(), color = (0, 0, 255))
print("蓝色圆形检测到,返回1")
# 检测白色圆形
circles = img.find_circles(threshold = 5000, x_margin = 20, y_margin = 20, r_margin = 20, r_min = min_radius, r_max = max_radius, roi = (0, 0, img.width(), img.height()), robust = True, r_step = 2, threshold_cb=lambda x: white_threshold)
if circles:
img.draw_circle(circles[0].x(), circles[0].y(), circles[0].r(), color = (255, 255, 255))
print("白色圆形检测到,返回2")
```
以上代码使用了OpenMV的find_circles函数来检测圆形,并使用了不同的阈值来区分不同颜色的圆形,最后根据检测到的圆形的颜色返回0、1、2。代码中还使用了摄像头来实时获取图像,并将检测到的圆形在图像上用圆形标出。
### 回答2:
要使用OpenMV来分别识别红蓝白圆形图案并返回相应的代码,可以使用颜色识别和形状识别的功能来实现。下面是一个可能的代码示例:
```python
import sensor
import image
import time
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
# 颜色阈值
red_threshold = (30, 100, 15, 127, 15, 127)
blue_threshold = (0, 50, -128, -40, -128, 0)
white_threshold = (200, 255)
# 循环读取图像并进行识别
while True:
img = sensor.snapshot()
# 检测红色圆形
red_circles = img.find_circles(threshold = 1000, x_margin = 10, y_margin = 10, r_margin = 10,
r_min = 10, r_max = 100, r_step = 2)
if red_circles:
# 返回0代表识别到红色圆形
print(0)
# 检测蓝色圆形
blue_circles = img.find_circles(color_thresholds = blue_threshold, threshold = 1000, x_margin = 10, y_margin = 10, r_margin = 10,
r_min = 10, r_max = 100, r_step = 2)
if blue_circles:
# 返回1代表识别到蓝色圆形
print(1)
# 检测白色圆形
white_circles = img.find_circles(color_thresholds = white_threshold, threshold = 1000, x_margin = 10, y_margin = 10, r_margin = 10,
r_min = 10, r_max = 100, r_step = 2)
if white_circles:
# 返回2代表识别到白色圆形
print(2)
```
这段代码首先初始化了摄像头,然后在一个循环中不断读取图像,并使用`find_circles()`函数来检测红色、蓝色和白色圆形。根据是否检测到相应的圆形,分别打印出0、1、2,以表示不同的识别结果。
### 回答3:
下面是使用OpenMV识别红色、蓝色和白色圆形图案,并分别返回0、1、2的代码:
```python
import sensor, image
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QQVGA)
sensor.skip_frames(time = 2000)
red_threshold = (30, 100, 15, 127, 15, 127) # 红色阈值
blue_threshold = (0, 30, -128, -25, -5, 40) # 蓝色阈值
white_threshold = (200, 255) # 白色阈值
while(True):
img = sensor.snapshot() # 获取图像快照
red_blobs = img.find_blobs([red_threshold]) # 检测红色色块
blue_blobs = img.find_blobs([blue_threshold]) # 检测蓝色色块
white_blobs = img.find_blobs([white_threshold]) # 检测白色色块
# 根据识别到的色块数量及颜色返回相应的结果
if len(red_blobs) > 0:
print("识别到红色圆形,返回0")
elif len(blue_blobs) > 0:
print("识别到蓝色圆形,返回1")
elif len(white_blobs) > 0:
print("识别到白色圆形,返回2")
else:
print("未识别到圆形")
```
运行该代码后,摄像头会实时获取图像并识别其中的红色、蓝色和白色圆形。如果识别到了红色圆形,则在控制台打印"识别到红色圆形,返回0";如果识别到了蓝色圆形,则打印"识别到蓝色圆形,返回1";如果识别到了白色圆形,则打印"识别到白色圆形,返回2";如果没有识别到圆形,则打印"未识别到圆形"。
相关推荐
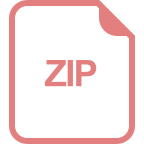














