linux void*thread(void*arg)
时间: 2023-06-18 15:08:24 浏览: 151
这是一个Linux下创建线程的函数,其函数原型为:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
```
该函数的参数解释如下:
- `thread`:指向线程标识符的指针,用于存储新创建线程的标识符。
- `attr`:线程属性,通常设为 NULL。
- `start_routine`:指向线程函数的指针,新创建的线程将从该函数的起始地址开始执行。
- `arg`:传递给线程函数的参数。
函数返回值为 0 表示线程创建成功,否则返回错误码。
需要注意的是,线程函数必须返回 `void*` 类型的指针,并且参数也是 `void*` 类型的指针。在线程函数内部,可以通过强制类型转换将参数还原为其实际类型。例如:
```c
void *my_thread_func(void *arg) {
int my_arg = *((int *) arg);
// ...
return NULL;
}
```
相关问题
编写以简单程序实现下列功能:主进程实现每个一秒输出字母A、B、C、D…等10个字母,创建一个线程,该线程实现每秒输出数字1、2、3…等10个数字//参考例题1:thread1.c#include <stdio.h>#include <unistd.h>#include <stdlib.h>#include <string.h>#include <pthread.h>void *thread_function(void *arg);char message[] = "Hello World";void *thread_function(void *arg) {printf("thread_function is running. Argument was %s\n", (char *)arg);sleep(3);strcpy(message, "Bye!");pthread_exit("Thank you for the CPU time");}int main() {int res;pthread_t a_thread;void *thread_result;res = pthread_create(&a_thread, NULL, thread_function, (void *)message);if (res != 0) {perror("Thread creation failed");exit(EXIT_FAILURE);}printf("Waiting for thread to finish...\n");res = pthread_join(a_thread, &thread_result);if (res != 0) {perror("Thread join failed");exit(EXIT_FAILURE);}printf("Thread joined, it returned %s\n", (char *)thread_result);printf("Message is now %s\n", message);exit(EXIT_SUCCESS);}
下面是实现题目要求的简单程序:
```c
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
void *print_letters(void *arg) {
int i;
for (i = 0; i < 10; i++) {
printf("%c ", 'A' + i);
fflush(stdout); // 刷新缓冲区
sleep(1);
}
pthread_exit(NULL);
}
void *print_numbers(void *arg) {
int i;
for (i = 1; i <= 10; i++) {
printf("%d ", i);
fflush(stdout); // 刷新缓冲区
sleep(1);
}
pthread_exit(NULL);
}
int main() {
pthread_t letter_thread, number_thread;
pthread_create(&letter_thread, NULL, print_letters, NULL);
pthread_create(&number_thread, NULL, print_numbers, NULL);
pthread_join(letter_thread, NULL);
pthread_join(number_thread, NULL);
return 0;
}
```
程序中使用了两个线程,一个用于输出字母,一个用于输出数字。两个线程都是通过sleep函数暂停1秒钟来实现每秒输出一个字符或数字。注意在输出字符和数字时要使用fflush(stdout)刷新缓冲区,否则可能出现输出不及时的情况。最后用pthread_join函数等待两个线程结束,释放资源。
void Game::game() { _beginthread([](void* arg) { Game::key_thread(arg); }, 0, NULL); _beginthread([](void* arg) { Game::timer_thread(arg); }, 0, NULL); Sleep(10000000000000000000); }显示非静态成员函数不能在lanbda体中引用怎么改
lambda表达式可以访问静态成员函数和静态成员变量,但是无法访问非静态成员函数和非静态成员变量,因为它没有this指针。所以,你需要将成员函数中需要访问的非静态成员变量作为参数传递给lambda表达式。修改后的代码如下:
```
void Game::game() {
int* arg1 = new int(0); // 参数1:key_thread函数需要的参数,需要在堆上分配内存
int* arg2 = new int(0); // 参数2:timer_thread函数需要的参数,需要在堆上分配内存
_beginthread([](void* arg) { Game::key_thread(arg); }, 0, arg1);
_beginthread([](void* arg) { Game::timer_thread(arg); }, 0, arg2);
Sleep(10000000000000000000);
}
```
在这里,我们为key_thread和timer_thread函数分别分配了一个int类型的参数,然后将参数传递给lambda表达式。在成员函数中,可以使用这个参数来访问非静态成员变量。需要注意的是,需要在不再需要这个参数时,手动释放内存,否则会造成内存泄漏。
阅读全文
相关推荐
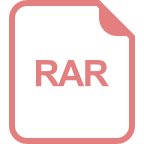
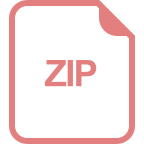
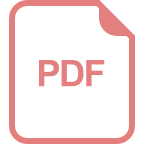












