解析一下 ~mystring() { if (str != nullptr) { delete [] str; } }
时间: 2024-02-15 07:44:15 浏览: 150
这是一个析构函数,用于释放对象中动态分配的内存。在这个析构函数中,首先进行了一个判断,判断str指针是否为nullptr,如果不是,说明这个对象中有动态分配的内存,需要将其释放。释放的方法是使用delete [] str,这表示释放str指针所指向的数组内存。需要注意的是,这里使用了delete [],而不是delete,因为str指针指向的是一个数组,而不是单个对象。使用delete []可以确保释放整个数组,而不是只释放第一个元素。
相关问题
完成 MyString 类的实现,使程序正确运行 #include <iostream> #include <cstring> using namespace std; class MyString { public: // 无参构造函数 MyString(); // 构造函数,传入一个C语言风格字符串 MyString(const char *s); // 拷贝构造函数 MyString(const MyString &s); // 析构函数 ~MyString(); // 将C语言风格的字符串赋值给MyString对象 MyString& operator=(const char *s); // 将一个MyString对象赋值给另一个MyString对象 MyString& operator=(const MyString &s); // 将这个MyString对象与C语言风格的字符串相连 MyString& operator+=(const char *s); // 将这个MyString对象与另一个MyString对象相连 MyString& operator+=(const MyString &s); // 返回下标为pos的字符 char& operator[](int pos); // 返回子串 [pos, pos+count) // 若请求的子串越过字符串的结尾,即count大于size() - pos,则返回的子串为[pos, size()) // 若pos不在字符串的下标范围内,返回空的MyString对象 // 若count == -1,返回子串[pos, size()) MyString substr(int pos = 0, int count = -1); // 字符串的长度 int size() const; // 返回C语言风格的字符串 const char* c_str() const; private: char *m_buf; int m_size; }; // 请在此处填写 int main() { char s[101]; cin.getline(s, 101); int pos, count; // 创建、拷贝、赋值 MyString s1(s), s2(s1), s3, s4, s5; s3 = s; s4 = s1; cout << "s1: " << s1.c_str() << endl; cout << "s2: " << s2.c_str() << endl; cout << "s3: " << s3.c_str() << endl; cout << "s4: " << s4.c_str() << endl; // 拼接 s3 += s; s4 += s1; cout << "s3 += s: " << s3.c_str() << endl; cout << "s4 += s1: " << s4.c_str() << endl; // 下标 cin >> pos; s4[pos] = 'm'; cout << "s4[pos] = 'm': " << s4.c_str() << endl; // 取子串 cin >> pos >> count; s5 = s4.substr(pos, count); cout << "s5: " << s5.c_str() << endl; // 以后还可以重载<<直接输出一个MyString对象哦 TODO return 0; }
实现如下:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class MyString {
public:
// 无参构造函数
MyString() : m_buf(nullptr), m_size(0) {}
// 构造函数,传入一个C语言风格字符串
MyString(const char *s) : m_buf(nullptr), m_size(0) {
if (s != nullptr) {
m_size = strlen(s);
m_buf = new char[m_size + 1];
strcpy(m_buf, s);
}
}
// 拷贝构造函数
MyString(const MyString &s) : m_buf(nullptr), m_size(s.m_size) {
if (m_size > 0) {
m_buf = new char[m_size + 1];
strcpy(m_buf, s.m_buf);
}
}
// 析构函数
~MyString() {
delete[] m_buf;
}
// 将C语言风格的字符串赋值给MyString对象
MyString& operator=(const char *s) {
if (s == nullptr) {
delete[] m_buf;
m_buf = nullptr;
m_size = 0;
}
else {
int len = strlen(s);
if (len > m_size) {
delete[] m_buf;
m_buf = new char[len + 1];
m_size = len;
}
strcpy(m_buf, s);
}
return *this;
}
// 将一个MyString对象赋值给另一个MyString对象
MyString& operator=(const MyString &s) {
if (this != &s) {
if (s.m_buf == nullptr) {
delete[] m_buf;
m_buf = nullptr;
m_size = 0;
}
else {
int len = s.m_size;
if (len > m_size) {
delete[] m_buf;
m_buf = new char[len + 1];
m_size = len;
}
strcpy(m_buf, s.m_buf);
}
}
return *this;
}
// 将这个MyString对象与C语言风格的字符串相连
MyString& operator+=(const char *s) {
int len = strlen(s);
char *new_buf = new char[m_size + len + 1];
if (m_buf != nullptr) {
strcpy(new_buf, m_buf);
delete[] m_buf;
}
strcpy(new_buf + m_size, s);
m_buf = new_buf;
m_size += len;
return *this;
}
// 将这个MyString对象与另一个MyString对象相连
MyString& operator+=(const MyString &s) {
return (*this += s.c_str());
}
// 返回下标为pos的字符
char& operator[](int pos) {
return m_buf[pos];
}
// 返回子串 [pos, pos+count)
// 若请求的子串越过字符串的结尾,即count大于size() - pos,则返回的子串为[pos, size())
// 若pos不在字符串的下标范围内,返回空的MyString对象
// 若count == -1,返回子串[pos, size())
MyString substr(int pos = 0, int count = -1) {
if (pos < 0 || pos >= m_size) {
return MyString();
}
if (count == -1) {
count = m_size - pos;
}
else if (count > m_size - pos) {
count = m_size - pos;
}
char *new_buf = new char[count + 1];
strncpy(new_buf, m_buf + pos, count);
new_buf[count] = '\0';
MyString res(new_buf);
delete[] new_buf;
return res;
}
// 字符串的长度
int size() const {
return m_size;
}
// 返回C语言风格的字符串
const char* c_str() const {
return m_buf;
}
private:
char *m_buf;
int m_size;
};
int main() {
char s[101];
cin.getline(s, 101);
int pos, count;
// 创建、拷贝、赋值
MyString s1(s), s2(s1), s3, s4, s5;
s3 = s;
s4 = s1;
cout << "s1: " << s1.c_str() << endl;
cout << "s2: " << s2.c_str() << endl;
cout << "s3: " << s3.c_str() << endl;
cout << "s4: " << s4.c_str() << endl;
// 拼接
s3 += s;
s4 += s1;
cout << "s3 += s: " << s3.c_str() << endl;
cout << "s4 += s1: " << s4.c_str() << endl;
// 下标
cin >> pos;
s4[pos] = 'm';
cout << "s4[pos] = 'm': " << s4.c_str() << endl;
// 取子串
cin >> pos >> count;
s5 = s4.substr(pos, count);
cout << "s5: " << s5.c_str() << endl;
return 0;
}
```
设计一个mystring类,包括数据成员char * pstr; 和 int length; 通过运算符重载实现字符串的输入>>、输出<<、连接+=、赋值=、关系运算(==、!=、>、<)、下标[]等运算。
好的,我理解了您的要求。以下是设计的mystring类:
```cpp
#include <iostream>
#include <cstring>
class mystring {
private:
char *pstr;
int length;
public:
// 构造函数
mystring() {
pstr = nullptr;
length = 0;
}
// 带参构造函数
mystring(const char *str) {
if (str == nullptr) {
pstr = nullptr;
length = 0;
} else {
length = strlen(str);
pstr = new char[length + 1];
strcpy(pstr, str);
}
}
// 拷贝构造函数
mystring(const mystring &other) {
if (other.pstr == nullptr) {
pstr = nullptr;
length = 0;
} else {
length = other.length;
pstr = new char[length + 1];
strcpy(pstr, other.pstr);
}
}
// 析构函数
~mystring() {
delete[] pstr;
}
// 运算符重载
friend std::ostream& operator<<(std::ostream& os, const mystring& str) {
os << str.pstr;
return os;
}
friend std::istream& operator>>(std::istream& is, mystring& str) {
char buffer[1024];
is >> buffer;
if (str.pstr != nullptr) {
delete[] str.pstr;
}
str.length = strlen(buffer);
str.pstr = new char[str.length + 1];
strcpy(str.pstr, buffer);
return is;
}
mystring& operator=(const mystring& other) {
if (this == &other) {
return *this;
}
if (pstr != nullptr) {
delete[] pstr;
}
if (other.pstr == nullptr) {
pstr = nullptr;
length = 0;
} else {
length = other.length;
pstr = new char[length + 1];
strcpy(pstr, other.pstr);
}
return *this;
}
mystring operator+(const mystring& other) {
mystring result;
result.length = length + other.length;
result.pstr = new char[result.length + 1];
strcpy(result.pstr, pstr);
strcat(result.pstr, other.pstr);
return result;
}
bool operator==(const mystring& other) {
return strcmp(pstr, other.pstr) == 0;
}
bool operator!=(const mystring& other) {
return strcmp(pstr, other.pstr) != 0;
}
bool operator>(const mystring& other) {
return strcmp(pstr, other.pstr) > 0;
}
bool operator<(const mystring& other) {
return strcmp(pstr, other.pstr) < 0;
}
char operator[](int index) {
if (index >= 0 && index < length) {
return pstr[index];
} else {
return '\0'; // 空字符表示越界或者字符串为空
}
}
};
```
您可以在代码中按需修改数据成员的名称和类型,或者添加其他需要实现的功能。但请保证数据成员和运算符重载的定义与本示例代码的要求一致,以确保代码能够正确运行。
阅读全文
相关推荐
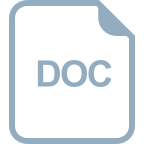
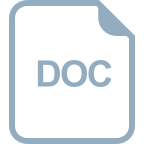
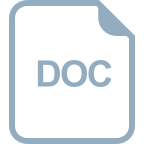












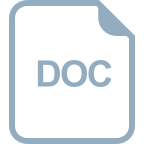
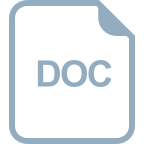