qt中用dijkstra算法计算最短路径编程,并注释
时间: 2024-06-12 17:04:15 浏览: 113
以下是使用Dijkstra算法计算最短路径的Qt C++示例代码(包含注释):
```
#include <QtDebug>
#include <QVector>
#include <limits>
// 定义无穷大的距离值
const int INF = std::numeric_limits<int>::max();
// 定义一个节点结构体,包含节点编号和从起点到该节点的距离
struct Node {
int id;
int distance;
Node(int _id, int _distance) : id(_id), distance(_distance) {}
};
// 定义边结构体,包含起点、终点和边权值
struct Edge {
int from;
int to;
int weight;
Edge(int _from, int _to, int _weight) : from(_from), to(_to), weight(_weight) {}
};
// Dijkstra算法求最短路径
QVector<int> dijkstra(const QVector<QVector<Edge>> &graph, int start, int end)
{
// 初始化距离数组和前驱数组
QVector<int> distance(graph.size(), INF);
QVector<int> predecessor(graph.size(), -1);
// 将起点的距离初始化为0
distance[start] = 0;
// 定义未访问节点集合
QVector<Node> unvisited;
for (int i = 0; i < graph.size(); ++i) {
unvisited.append(Node(i, distance[i]));
}
// Dijkstra算法主循环
while (!unvisited.isEmpty()) {
// 从未访问节点集合中选择距离最短的节点
int current = -1;
int minDistance = INF;
for (const Node &node : unvisited) {
if (node.distance < minDistance) {
current = node.id;
minDistance = node.distance;
}
}
// 如果当前节点是终点,则直接返回最短路径
if (current == end) {
QVector<int> path;
while (current != -1) {
path.prepend(current);
current = predecessor[current];
}
return path;
}
// 从未访问节点集合中移除当前节点
unvisited.erase(std::remove_if(unvisited.begin(), unvisited.end(), [&](const Node &node) {
return node.id == current;
}), unvisited.end());
// 遍历当前节点的所有邻居节点,更新距离和前驱
for (const Edge &edge : graph[current]) {
int neighbor = edge.to;
int newDistance = distance[current] + edge.weight;
if (newDistance < distance[neighbor]) {
distance[neighbor] = newDistance;
predecessor[neighbor] = current;
// 更新未访问节点集合中邻居节点的距离
for (Node &node : unvisited) {
if (node.id == neighbor) {
node.distance = newDistance;
break;
}
}
}
}
}
// 如果未访问节点集合为空但终点仍未被访问,则不存在从起点到终点的路径
return QVector<int>();
}
// 示例用法
int main()
{
// 定义一个有向加权图,共5个节点,7条有向边
QVector<QVector<Edge>> graph(5);
graph[0].append(Edge(0, 1, 10));
graph[0].append(Edge(0, 3, 5));
graph[1].append(Edge(1, 2, 1));
graph[1].append(Edge(1, 3, 2));
graph[2].append(Edge(2, 4, 4));
graph[3].append(Edge(3, 1, 3));
graph[3].append(Edge(3, 2, 9));
graph[3].append(Edge(3, 4, 2));
// 计算从节点0到节点4的最短路径
QVector<int> path = dijkstra(graph, 0, 4);
// 输出最短路径
if (!path.isEmpty()) {
qDebug() << "Shortest path from node 0 to node 4:";
for (int i = 0; i < path.size(); ++i) {
qDebug() << path[i];
if (i < path.size() - 1) {
qDebug() << "->";
}
}
} else {
qDebug() << "There is no path from node 0 to node 4.";
}
return 0;
}
```
阅读全文
相关推荐












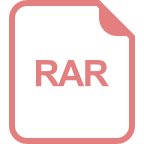

