java结合opcua遍历树形节点
时间: 2023-07-07 10:38:43 浏览: 57
要结合Java和OPCUA遍历树形节点,首先需要使用OPCUA的Java SDK(如Eclipse Milo)与OPCUA服务器进行通信。然后,可以使用递归算法遍历节点树。
下面是一个简单的示例代码,可以使用Eclipse Milo遍历OPCUA服务器中的节点树:
```java
import org.eclipse.milo.opcua.sdk.client.OpcUaClient;
import org.eclipse.milo.opcua.sdk.client.api.UaNodeCache;
import org.eclipse.milo.opcua.sdk.client.util.DataTypeTree;
import org.eclipse.milo.opcua.stack.core.Identifiers;
import org.eclipse.milo.opcua.stack.core.UaException;
import org.eclipse.milo.opcua.stack.core.types.builtin.DataValue;
import org.eclipse.milo.opcua.stack.core.types.builtin.NodeId;
import org.eclipse.milo.opcua.stack.core.types.builtin.QualifiedName;
import org.eclipse.milo.opcua.stack.core.types.builtin.Variant;
import org.eclipse.milo.opcua.stack.core.types.enumerated.NodeClass;
import org.eclipse.milo.opcua.stack.core.types.structured.BrowseResult;
import org.eclipse.milo.opcua.stack.core.types.structured.ReferenceDescription;
import java.util.List;
import java.util.concurrent.CompletableFuture;
public class OpcUaNodeTreeTraversal {
private static final NodeId ROOT_NODE_ID = Identifiers.RootFolder;
public static void main(String[] args) throws Exception {
// Connect to OPCUA server
OpcUaClient client = connectToOpcUaServer();
// Traverse the node tree
traverseNodeTree(client, ROOT_NODE_ID);
// Disconnect from OPCUA server
client.disconnect();
}
private static OpcUaClient connectToOpcUaServer() throws Exception {
// Create and configure OPCUA client
OpcUaClient client = null; // TODO: Configure client
// Connect to OPCUA server
client.connect().get();
return client;
}
private static void traverseNodeTree(OpcUaClient client, NodeId nodeId) throws Exception {
// Browse the node
BrowseResult browseResult = client.browse(nodeId).get();
List<ReferenceDescription> references = browseResult.getReferences();
// Get node information
UaNodeCache nodeCache = client.getAddressSpace().getNode(nodeId);
NodeClass nodeClass = nodeCache.getNodeClass();
QualifiedName browseName = nodeCache.getBrowseName();
DataTypeTree dataTypeTree = nodeCache.getDataTypeTree();
DataValue value = client.readValue(nodeId).get();
// Print node information
System.out.println("Node: " + nodeId);
System.out.println("Node Class: " + nodeClass);
System.out.println("Browse Name: " + browseName);
System.out.println("Data Type Tree: " + dataTypeTree);
System.out.println("Value: " + value);
// Traverse child nodes
for (ReferenceDescription reference : references) {
NodeId childNodeId = reference.getNodeId().toNodeId();
traverseNodeTree(client, childNodeId);
}
}
}
```
在这个示例中,我们首先连接到OPCUA服务器,然后从根节点开始遍历节点树。对于每个节点,我们打印节点信息,然后递归遍历其子节点。递归算法会一直遍历到叶子节点,然后返回到父节点并继续遍历其它子节点。
请注意,这只是一个基本示例,实际应用中可能需要处理更多的异常情况和性能问题。
相关推荐
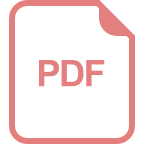












