python批量提取多个浮点型高光谱文件平均反射率并存储到csv
时间: 2023-05-27 08:01:57 浏览: 58
可以使用Python中的pandas和numpy库来实现批量提取高光谱文件的平均反射率,并将结果存储为csv文件。
首先,需要引入所需库:
```python
import os
import pandas as pd
import numpy as np
```
然后,定义一个函数,用于读取高光谱文件并计算平均反射率。
```python
def process_file(filepath):
data = pd.read_csv(filepath, header=None, delim_whitespace=True)
# 这里根据数据格式修改header和delim_whitespace参数
average_reflectance = np.average(data.iloc[:, 1:].values, axis=1)
# 取第2列到最后一列的数据计算平均值
return average_reflectance
```
接着,定义一个函数来扫描指定目录下的所有高光谱文件,并将计算得到的平均反射率存储到一个列表中。
```python
def batch_process_files(folder):
file_list = os.listdir(folder)
average_reflectances = []
for file in file_list:
filepath = os.path.join(folder, file)
if os.path.isfile(filepath) and file.endswith('.txt'):
average_reflectance = process_file(filepath)
average_reflectances.append(average_reflectance)
print(f'Processed {filepath}')
return average_reflectances
```
最后,将结果存储到csv文件中。
```python
folder = 'path/to/files/'
output_filename = 'output.csv'
average_reflectances = batch_process_files(folder)
output_data = pd.DataFrame(average_reflectances)
output_data.to_csv(output_filename, index=False, header=False)
print(f'Saved output to {output_filename}')
```
完整代码:
```python
import os
import pandas as pd
import numpy as np
def process_file(filepath):
data = pd.read_csv(filepath, header=None, delim_whitespace=True)
average_reflectance = np.average(data.iloc[:, 1:].values, axis=1)
return average_reflectance
def batch_process_files(folder):
file_list = os.listdir(folder)
average_reflectances = []
for file in file_list:
filepath = os.path.join(folder, file)
if os.path.isfile(filepath) and file.endswith('.txt'):
average_reflectance = process_file(filepath)
average_reflectances.append(average_reflectance)
print(f'Processed {filepath}')
return average_reflectances
folder = 'path/to/files/'
output_filename = 'output.csv'
average_reflectances = batch_process_files(folder)
output_data = pd.DataFrame(average_reflectances)
output_data.to_csv(output_filename, index=False, header=False)
print(f'Saved output to {output_filename}')
```
相关推荐
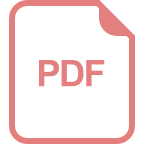
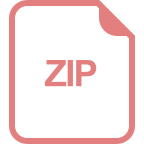














