c++基于栈的中缀表达式
时间: 2023-11-20 08:55:27 浏览: 67
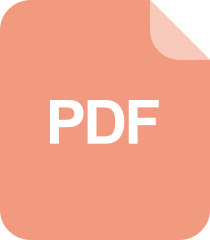
C++利用栈实现中缀表达式转后缀表达式
以下是C++基于栈的中缀表达式求值的示例代码:
```cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 定义运算符优先级
int priority(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else {
return 0;
}
}
// 中缀表达式转后缀表达式
string infixToPostfix(string infix) {
stack<char> s;
string postfix = "";
for (int i = 0; i < infix.length(); i++) {
char c = infix[i];
if (isdigit(c)) {
postfix += c;
} else if (c == '(') {
s.push(c);
} else if (c == ')') {
while (!s.empty() && s.top() != '(') {
postfix += s.top();
s.pop();
}
s.pop();
} else {
while (!s.empty() && priority(s.top()) >= priority(c)) {
postfix += s.top();
s.pop();
}
s.push(c);
}
}
while (!s.empty()) {
postfix += s.top();
s.pop();
}
return postfix;
}
// 计算后缀表达式的值
int evaluatePostfix(string postfix) {
stack<int> s;
for (int i = 0; i < postfix.length(); i++) {
char c = postfix[i];
if (isdigit(c)) {
s.push(c - '0');
} else {
int operand2 = s.top();
s.pop();
int operand1 = s.top();
s.pop();
switch (c) {
case '+':
s.push(operand1 + operand2);
break;
case '-':
s.push(operand1 - operand2);
break;
case '*':
s.push(operand1 * operand2);
break;
case '/':
s.push(operand1 / operand2);
break;
}
}
}
return s.top();
}
int main() {
string infix = "1+(2-3)*4+10/5";
string postfix = infixToPostfix(infix);
cout << "Postfix expression: " << postfix << endl;
int result = evaluatePostfix(postfix);
cout << "Result: " << result << endl;
return 0;
}
```
阅读全文
相关推荐
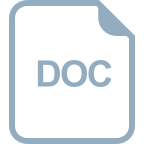
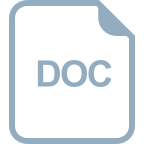
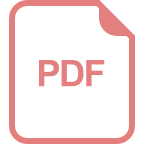

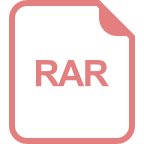
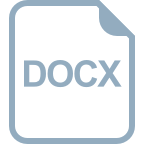
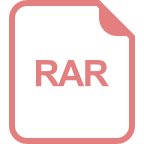
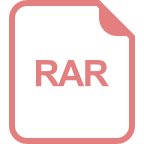
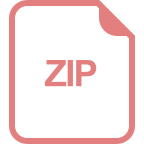




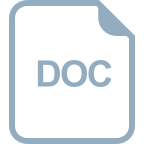
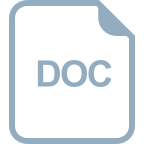