实现一个4*4的对称数组的压缩存储C语言版
时间: 2024-10-26 18:16:49 浏览: 30
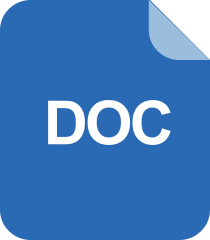
特殊矩阵的压缩存储算法的实现 课程设计说明书.doc
在C语言中,我们可以使用一个一维数组和一个偏移量来实现4x4对称数组的压缩存储。对称数组意味着数组的左上到右下的元素是相同的,比如:
```
[0][0] [0][1] [0][2] [0][3]
[1][0] [1][1] [1][2] [1][3]
[2][0] [2][1] [2][2] [2][3]
[3][0] [3][1] [3][2] [3][3]
等价于:
[0][0] [0][1] [0][2] [0][3] -> [0][0], [1][1], [2][2], [3][3]
[1][0] [1][1] [1][2] [1][3] -> [0][1], [1][1], [2][2], [3][1]
[2][0] [2][1] [2][2] [2][3] -> [0][2], [1][2], [2][2], [3][0]
[3][0] [3][1] [3][2] [3][3] -> [0][3], [1][3], [2][3], [3][2]
```
我们可以只存储前两行或前两列的值,然后通过计算偏移量获取其余位置的值。例如:
```c
int compressed_array[8]; // 存储四个对角线元素
int offset = 0; // 初始偏移
// 压缩存储
compressed_array[0] = array[0][0];
compressed_array[1] = array[1][1];
compressed_array[2] = array[0][1]; // 对应于原数组的右上角
compressed_array[3] = array[1][0]; // 对应于原数组的左下角
compressed_array[4] = array[0][2]; // 可由0,1的偏移计算得出
compressed_array[5] = array[2][0];
compressed_array[6] = array[0][3];
compressed_array[7] = array[3][0];
// 读取时
int value;
if (index <= 4) {
value = compressed_array[index];
} else {
index -= 4; // 跳过对角线元素
if (index % 2 == 0) { // 如果索引位于对称轴左侧
value = compressed_array[index / 2] + offset;
} else {
value = compressed_array[index / 2 - 1] + offset;
}
}
```
阅读全文
相关推荐
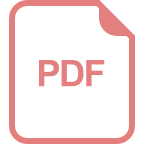
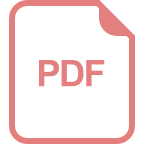


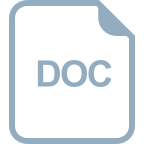
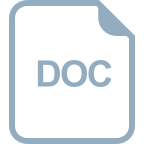
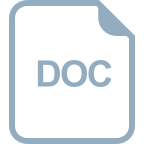
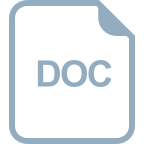
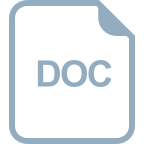
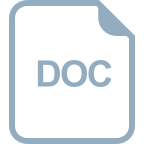
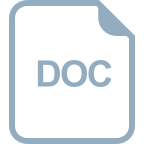






