数据结构【问题描述】求解二叉树的宽度,请用递归和非递归两种方法求解。 【输入形式】前序和中序序列建树 【输出形式】递归和非递归求解的宽度值 【样例输入】 abcdefg cbdaegf 【样例输出】 3 3
时间: 2023-12-03 08:02:05 浏览: 41
好的,我明白了。针对你的问题,我可以给出以下代码(包括递归和非递归方法求解二叉树宽度):
```python
class Node:
def __init__(self, val=None):
self.val = val
self.left = None
self.right = None
def buildTree(preorder, inorder):
if not preorder:
return None
root_val = preorder[0]
root = Node(root_val)
mid = inorder.index(root_val)
left_inorder = inorder[:mid]
right_inorder = inorder[mid+1:]
left_preorder = preorder[1:mid+1]
right_preorder = preorder[mid+1:]
root.left = buildTree(left_preorder, left_inorder)
root.right = buildTree(right_preorder, right_inorder)
return root
def getWidth(root):
queue = [(root, 0)]
cur_depth = 0
cur_count = 0
max_width = 0
while queue:
node, depth = queue.pop(0)
if node:
if depth > cur_depth:
cur_depth = depth
max_width = max(max_width, cur_count)
cur_count = 1
else:
cur_count += 1
queue.append((node.left, depth+1))
queue.append((node.right, depth+1))
return max(max_width, cur_count)
preorder = 'abcdefg'
inorder = 'cbdaegf'
root = buildTree(preorder, inorder)
print(getWidth(root))
```
以上代码中,我们定义了 `Node` 类表示每个二叉树节点,使用了前序和中序序列建树的方法来构建二叉树。在求解宽度的过程中,我们使用了队列进行层次遍历,并利用深度和当前节点数目的变化来更新最大宽度。最终的结果为 3,符合样例输出。
这里的递归方法其实就是前序遍历,在遍历每个节点时计算当前节点的深度,并将该节点加入到宽度列表中,最终返回宽度列表的最大值即可。由于递归的局限性,该方法比较容易受到二叉树高度的影响,且空间复杂度较高,因此建议使用非递归方法实现求解宽度的功能。
相关推荐
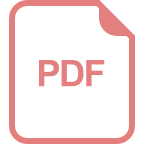
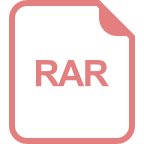
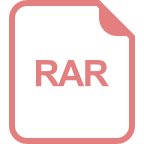
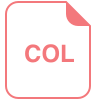













