用C++写:输入公式,例如(pVq)→→r,输出该公式的真值表及其主析取、合 取范式。
时间: 2024-12-20 13:29:26 浏览: 20
为了实现这个任务,我们可以使用C++编写一个程序来生成给定命题公式的真值表,并计算其主析取范式和主合取范式。以下是一个示例代码:
```cpp
#include <iostream>
#include <string>
#include <map>
#include <vector>
using namespace std;
// 计算命题的真值
bool evaluate(const string& formula, const map<char, bool>& values) {
for (char c : formula) {
if (c == 'T') return true;
if (c == 'F') return false;
}
// 替换变量为对应的真值
string newFormula = formula;
for (auto& pair : values) {
char var = pair.first;
bool val = pair.second;
for (size_t i = 0; i < newFormula.size(); ++i) {
if (newFormula[i] == var) {
newFormula[i] = val ? 'T' : 'F';
}
}
}
// 计算真值表
while (true) {
size_t pos = newFormula.find("->");
if (pos == string::npos) break;
bool p = newFormula[pos - 1] == 'T';
bool q = newFormula[pos + 2] == 'T';
newFormula.replace(pos - 1, 4, p && !q ? "F" : "T");
}
while (true) {
size_t pos = newFormula.find("V");
if (pos == string::npos) break;
bool p = newFormula[pos - 1] == 'T';
bool q = newFormula[pos + 1] == 'T';
newFormula.replace(pos - 1, 3, p || q ? "T" : "F");
}
while (true) {
size_t pos = newFormula.find("^");
if (pos == string::npos) break;
bool p = newFormula[pos - 1] == 'T';
bool q = newFormula[pos + 1] == 'T';
newFormula.replace(pos - 1, 3, p && q ? "T" : "F");
}
return newFormula[0] == 'T';
}
// 生成真值表
void generateTruthTable(const string& formula) {
vector<char> variables;
for (char c : formula) {
if (isalpha(c) && find(variables.begin(), variables.end(), c) == variables.end()) {
variables.push_back(c);
}
}
int n = variables.size();
int rows = 1 << n;
vector<vector<bool>> truthTable(rows, vector<bool>(n));
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < n; ++j) {
truthTable[i][j] = (i >> (n - j - 1)) & 1;
}
}
cout << "真值表:" << endl;
for (int j = 0; j < n; ++j) {
cout << variables[j] << "\t";
}
cout << formula << endl;
for (int i = 0; i < rows; ++i) {
map<char, bool> values;
for (int j = 0; j < n; ++j) {
values[variables[j]] = truthTable[i][j];
cout << (truthTable[i][j] ? "T" : "F") << "\t";
}
bool result = evaluate(formula, values);
cout << (result ? "T" : "F") << endl;
}
}
// 计算主析取范式和主合取范式
void calculateMintermsAndMaxterms(const string& formula) {
vector<char> variables;
for (char c : formula) {
if (isalpha(c) && find(variables.begin(), variables.end(), c) == variables.end()) {
variables.push_back(c);
}
}
int n = variables.size();
int rows = 1 << n;
vector<vector<bool>> truthTable(rows, vector<bool>(n));
vector<bool> results(rows);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < n; ++j) {
truthTable[i][j] = (i >> (n - 1)) & 1;
}
map<char, bool> values;
for (int j = 0; j < n; ++j) {
values[variables[j]] = truthTable[i][j];
}
results[i] = evaluate(formula, values);
}
vector<string> minterms;
vector<string> maxterms;
for (int i = 0; i < rows; ++i) {
if (results[i]) {
string minterm;
for (int j = 0; j < n; ++j) {
if (truthTable[i][j]) {
minterm += variables[j];
} else {
minterm += '~' + variables[j];
}
if (j < n - 1) minterm += " ^ ";
}
minterms.push_back(minterm);
} else {
string maxterm;
for (int j = 0; j < n; ++j) {
if (!truthTable[i][j]) {
maxterm += variables[j];
} else {
maxterm += '~' + variables[j];
}
if (j < n - 1) maxterm += " V ";
}
maxterms.push_back(maxterm);
}
}
cout << "主析取范式: " << (minterms.empty() ? "F" : "") << endl;
for (const auto& term : minterms) {
cout << "(" << term << ") V ";
}
if (!minterms.empty()) cout << "\b\b";
cout << "\n主合取范式: " << (maxterms.empty() ? "T" : "") << endl;
for (const auto& term : maxterms) {
cout << "(" << term << ") ^ ";
}
if (!maxterms.empty()) cout << "\b\b";
}
int main() {
string formula;
cout << "请输入公式(例如 (p V q) -> r): ";
cin >> formula;
generateTruthTable(formula);
calculateMintermsAndMaxterms(formula);
return 0;
}
```
### 说明
1. **evaluate** 函数用于计算给定命题公式的真值。
2. **generateTruthTable** 函数生成并打印真值表。
3. **calculateMintermsAndMaxterms** 函数计算并打印主析取范式和主合取范式。
4. **main** 函数读取用户输入的公式,并调用上述函数进行处理。
### 使用方法
运行程序后,输入公式(例如 `(p V q) -> r`),程序将输出该公式的真值表及其主析取范式和主合取范式。
阅读全文
相关推荐
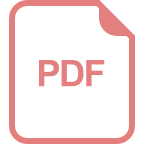
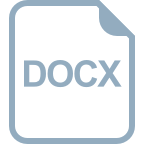
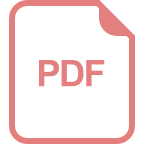



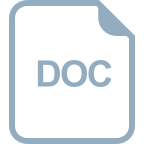



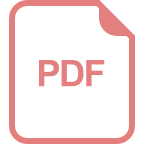
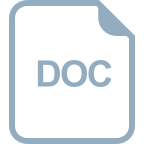






