C++实现1、先序遍历的顺序建立二叉树。 2、计算二叉树的深度。 3、统计二叉树中节点的个数。
时间: 2023-11-13 12:25:07 浏览: 42
好的,我会回答你的问题。
1、C++实现先序遍历的顺序建立二叉树:
```c++
#include <iostream>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
TreeNode* buildTree() {
int val;
cin >> val;
if (val == -1) {
return NULL;
}
TreeNode* root = new TreeNode(val);
root->left = buildTree();
root->right = buildTree();
return root;
}
void preOrder(TreeNode* root) {
if (root == NULL) {
return;
}
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
}
int main() {
cout << "请输入先序遍历序列(-1表示空节点):" << endl;
TreeNode* root = buildTree();
cout << "先序遍历结果为:" << endl;
preOrder(root);
return 0;
}
```
2、C++实现计算二叉树的深度:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
int maxDepth(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return max(leftDepth, rightDepth) + 1;
}
int main() {
cout << "请输入先序遍历序列(-1表示空节点):" << endl;
TreeNode* root = buildTree();
int depth = maxDepth(root);
cout << "二叉树的深度为:" << depth << endl;
return 0;
}
```
3、C++实现统计二叉树中节点的个数:
```c++
#include <iostream>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
int countNodes(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftCount = countNodes(root->left);
int rightCount = countNodes(root->right);
return leftCount + rightCount + 1;
}
int main() {
cout << "请输入先序遍历序列(-1表示空节点):" << endl;
TreeNode* root = buildTree();
int count = countNodes(root);
cout << "二叉树中节点的个数为:" << count << endl;
return 0;
}
```
相关推荐
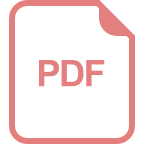
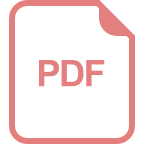
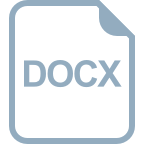














