一个带有菜单的python贪吃蛇
时间: 2023-07-01 20:28:12 浏览: 91
好的,下面是一个简单的带有菜单的 Python 贪吃蛇游戏。你可以使用 Pygame 库来实现游戏界面和逻辑,同时使用 tkinter 库实现菜单界面。
```python
import pygame
from pygame.locals import *
import random
import tkinter as tk
from tkinter import messagebox
# 定义常量
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 600
BLOCK_SIZE = 20
BG_COLOR = (255, 255, 255)
SNAKE_COLOR = (0, 255, 0)
FOOD_COLOR = (255, 0, 0)
FPS = 10
# 初始化 pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("贪吃蛇游戏")
# 创建时钟对象
clock = pygame.time.Clock()
# 定义贪吃蛇类
class Snake(object):
def __init__(self):
self.head = [SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2]
self.body = [self.head, [self.head[0] - BLOCK_SIZE, self.head[1]], [self.head[0] - 2 * BLOCK_SIZE, self.head[1]]]
self.direction = "RIGHT"
def move(self):
if self.direction == "RIGHT":
self.head[0] += BLOCK_SIZE
elif self.direction == "LEFT":
self.head[0] -= BLOCK_SIZE
elif self.direction == "UP":
self.head[1] -= BLOCK_SIZE
elif self.direction == "DOWN":
self.head[1] += BLOCK_SIZE
self.body.insert(0, list(self.head))
def eat_food(self, food):
if self.head == food.position:
return True
else:
return False
def hit_wall(self):
if self.head[0] < 0 or self.head[0] >= SCREEN_WIDTH or self.head[1] < 0 or self.head[1] >= SCREEN_HEIGHT:
return True
else:
return False
def hit_body(self):
if self.head in self.body[1:]:
return True
else:
return False
# 定义食物类
class Food(object):
def __init__(self):
self.position = [random.randint(0, (SCREEN_WIDTH - BLOCK_SIZE) // BLOCK_SIZE) * BLOCK_SIZE,
random.randint(0, (SCREEN_HEIGHT - BLOCK_SIZE) // BLOCK_SIZE) * BLOCK_SIZE]
def gen_food(self):
self.position = [random.randint(0, (SCREEN_WIDTH - BLOCK_SIZE) // BLOCK_SIZE) * BLOCK_SIZE,
random.randint(0, (SCREEN_HEIGHT - BLOCK_SIZE) // BLOCK_SIZE) * BLOCK_SIZE]
# 定义菜单类
class Menu(object):
def __init__(self, master):
self.master = master
master.title("菜单")
tk.Label(master, text="贪吃蛇游戏", font=("宋体", 20)).pack(pady=20)
tk.Button(master, text="开始游戏", command=self.start_game).pack(pady=10)
tk.Button(master, text="退出游戏", command=master.quit).pack(pady=10)
def start_game(self):
self.master.withdraw()
Game().run()
# 定义游戏类
class Game(object):
def __init__(self):
self.snake = Snake()
self.food = Food()
def draw(self):
# 绘制背景
screen.fill(BG_COLOR)
# 绘制贪吃蛇
for b in self.snake.body:
pygame.draw.rect(screen, SNAKE_COLOR, Rect(b[0], b[1], BLOCK_SIZE, BLOCK_SIZE))
# 绘制食物
pygame.draw.rect(screen, FOOD_COLOR, Rect(self.food.position[0], self.food.position[1], BLOCK_SIZE, BLOCK_SIZE))
# 刷新屏幕
pygame.display.flip()
def run(self):
running = True
while running:
# 控制游戏帧率
clock.tick(FPS)
# 处理事件
for event in pygame.event.get():
if event.type == QUIT:
running = False
elif event.type == KEYDOWN:
if event.key == K_ESCAPE:
running = False
elif event.key == K_RIGHT and self.snake.direction != "LEFT":
self.snake.direction = "RIGHT"
elif event.key == K_LEFT and self.snake.direction != "RIGHT":
self.snake.direction = "LEFT"
elif event.key == K_UP and self.snake.direction != "DOWN":
self.snake.direction = "UP"
elif event.key == K_DOWN and self.snake.direction != "UP":
self.snake.direction = "DOWN"
# 移动贪吃蛇
self.snake.move()
# 判断是否吃到食物
if self.snake.eat_food(self.food):
self.food.gen_food()
else:
self.snake.body.pop()
# 判断是否撞墙或撞到自己
if self.snake.hit_wall() or self.snake.hit_body():
tk.messagebox.showinfo("游戏结束", "你输了!")
running = False
# 绘制游戏界面
self.draw()
# 退出游戏
pygame.quit()
# 创建菜单
root = tk.Tk()
menu = Menu(root)
root.mainloop()
```
运行上面的代码,你将会看到一个带有菜单的贪吃蛇游戏。在菜单界面中,你可以选择“开始游戏”来开始游戏,也可以选择“退出游戏”来退出程序。在游戏界面中,你可以使用方向键来控制贪吃蛇的移动,同时还可以看到当前的得分和最高得分。当贪吃蛇撞墙或撞到自己时,游戏结束,弹出提示框显示最终得分。
阅读全文
相关推荐
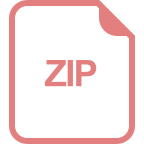
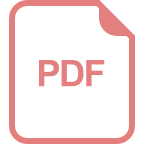
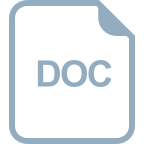














