Implement+the+following+function+that+returns+a+suffix+of+an+integer+with+a+specified+number+of+digi
时间: 2024-01-09 15:22:49 浏览: 152
根据提供的引用内容,以下是一个实现返回指定位数的整数后缀的函数的示例代码:
```go
func getSuffix(num int, digits int) int {
divisor := 1
for i := 0; i < digits; i++ {
divisor *= 10
}
suffix := num % divisor
return suffix
}
```
这个函数接受两个参数:num表示要获取后缀的整数,digits表示要获取的后缀的位数。函数首先计算出一个除数,该除数是10的digits次方,然后使用取模运算符将num除以除数得到后缀。
以下是一个示例调用该函数的代码:
```go
num := 123456789
digits := 3
suffix := getSuffix(num, digits)
fmt.Println(suffix) // 输出:789
```
相关问题
Write+a+Python+function+to+implement+a+recursive+algorithm+which+counts+the+number+of+ nodes+in+a+s
以下是一个用Python实现递归算法来计算树中节点数量的函数:
```python
def count_nodes(tree):
if tree is None:
return 0
else:
return 1 + count_nodes(tree.left) + count_nodes(tree.right)
```
这个函数接受一个树作为参数,并返回树中节点的数量。它使用递归的方式来计算节点数量。如果树为空,则返回0。否则,返回1加上左子树和右子树中节点的数量之和。
Write+code+for+a+function+with+the+following+prototype: /*+Addition+that+saturates+to+TMin+or+TMax+*
Sure! Here's an implementation of the function you requested:
```c++
#include <limits.h>
int saturating_add(int x, int y) {
int sum = x + y;
int neg_over = x < 0 && y < 0 && sum >= 0;
int pos_over = x >= 0 && y >= 0 && sum < 0;
int is_overflow = neg_over || pos_over;
int tmin = INT_MIN;
int tmax = INT_MAX;
int result = is_overflow ? (sum < 0 ? tmin : tmax) : sum;
return result;
}
```
This function takes two integer arguments `x` and `y`, and returns their sum. However, if the sum overflows the range of representable integers, the function "saturates" the result to either the minimum or maximum representable integer value, depending on the sign of the operands.
To implement this behavior, we first compute the sum of `x` and `y`. We then check whether the sum overflows by comparing the signs of `x`, `y`, and `sum`. If `x` and `y` have the same sign and `sum` has the opposite sign, then overflow has occurred. We store this information in the `is_overflow` variable.
If overflow has occurred, we set the result to either the minimum or maximum representable integer value, depending on whether the sum is negative or positive. Otherwise, we set the result to the sum itself.
I hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐
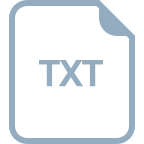














