现在我想用python实现∂ϕ /∂t = λ∇2ϕ − 2ϕ(1 − ϕ)f(ϕ, σ), ∂σ /∂t = η∇2σ + Sh(1 − ϕ) + Scϕ − (γh(1 − ϕ) + γcϕ)σ (2) f(ϕ, σ) = M(1 − 2ϕ − 3m(σ)) ,m(σ) = mref ( (ρ + A )/2 + (ρ − A) /π arctan( (σ − σl)/ σr ))
时间: 2023-08-05 17:04:57 浏览: 47
To implement these equations in Python, you will need to discretize the spatial and temporal domains, and then use numerical methods to solve the resulting system of ordinary differential equations (ODEs). One possible approach is to use a finite difference method to approximate the spatial derivatives, and then use an ODE solver such as the scipy.integrate.odeint function to integrate the resulting system of ODEs over time.
Here is some sample code that demonstrates this approach:
```python
import numpy as np
from scipy.integrate import odeint
# Define the parameters
L = 1.0 # Length of the domain
N = 100 # Number of grid points
dx = L / (N - 1) # Grid spacing
dt = 0.01 # Time step
t_final = 1.0 # Final time
lambda_ = 0.1 # Diffusion coefficient
eta = 0.1 # Diffusion coefficient
Sh = 0.5 # Advection coefficient
Sc = 0.5 # Advection coefficient
gamma_h = 0.1 # Decay coefficient
gamma_c = 0.1 # Decay coefficient
M = 1.0 # Reaction coefficient
rho = 1.0 # Density
A = 1.0 # Density
sigma_l = 0.1 # Threshold
sigma_r = 0.2 # Saturation
m_ref = 0.5 # Reference value
# Define the initial conditions
phi0 = np.zeros(N)
sigma0 = np.zeros(N)
phi0[N//2] = 1.0
# Define the reaction function
def f(phi, sigma):
m = m_ref * ((rho + A) / 2 + (rho - A) / np.pi * np.arctan((sigma - sigma_l) / sigma_r))
return M * (1 - 2 * phi - 3 * m)
# Define the right-hand side of the ODE system
def rhs(y, t):
phi = y[:N]
sigma = y[N:]
dphi = np.zeros(N)
dsigma = np.zeros(N)
dphi[1:-1] = lambda_ / dx**2 * (phi[2:] - 2 * phi[1:-1] + phi[:-2]) - 2 * phi[1:-1] * (1 - phi[1:-1]) * f(phi[1:-1], sigma[1:-1])
dsigma[1:-1] = eta / dx**2 * (sigma[2:] - 2 * sigma[1:-1] + sigma[:-2]) + Sh * (1 - phi[1:-1]) - Sc * phi[1:-1] - (gamma_h * (1 - phi[1:-1]) + gamma_c * phi[1:-1]) * sigma[1:-1]
return np.concatenate([dphi, dsigma])
# Integrate the ODE system
t = np.linspace(0, t_final, int(t_final / dt) + 1)
y0 = np.concatenate([phi0, sigma0])
y = odeint(rhs, y0, t)
# Extract the solution
phi = y[:, :N]
sigma = y[:, N:]
# Plot the solution
import matplotlib.pyplot as plt
plt.imshow(phi.T, origin='lower', extent=[0, L, 0, t_final], aspect='auto')
plt.xlabel('x')
plt.ylabel('t')
plt.title('Phi')
plt.colorbar()
plt.show()
plt.imshow(sigma.T, origin='lower', extent=[0, L, 0, t_final], aspect='auto')
plt.xlabel('x')
plt.ylabel('t')
plt.title('Sigma')
plt.colorbar()
plt.show()
```
Note that this is just one possible implementation, and there are many variations and improvements that can be made depending on the specific requirements of your problem.
相关推荐
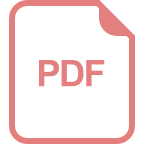
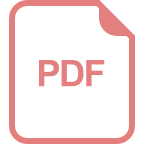















