pygame炮弹以抛物线飞至指定坐标
时间: 2023-06-17 11:06:22 浏览: 139
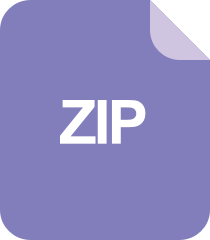
Pygame经典飞机大战(双人版)源码

要让炮弹按照抛物线飞行至指定坐标,可以使用物理公式来计算出炮弹的运动轨迹。假设炮弹的初始度为v0,发射角度为theta,重力加速度为g,炮弹的位置坐标为(x,y),运动时间为t,则可以使用以下公式计算炮弹的位置:
x = v0 * t * cos(theta)
y = v0 * t * sin(theta) - 0.5 * g * t * t
为了让炮弹飞行到指定的坐标(x_target, y_target),我们需要先计算出炮弹的发射角度theta,然后不断调整初始速度v0,直到计算出的轨迹能够到达指定坐标。
下面是一个使用pygame实现的示例代码:
```
import pygame
import math
# 初始化pygame
pygame.init()
# 设置屏幕大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置炮弹的初始位置和速度
x = 50
y = screen_height - 50
v0 = 100
# 设置目标坐标
x_target = screen_width - 50
y_target = screen_height - 200
# 计算发射角度
theta = math.atan2(y_target - y, x_target - x)
# 计算炮弹的运动轨迹
t = 0
g = 9.8
while True:
# 计算炮弹的位置
x = int(v0 * t * math.cos(theta))
y = int(screen_height - (v0 * t * math.sin(theta) - 0.5 * g * t * t))
# 绘制炮弹
pygame.draw.circle(screen, (255, 0, 0), (x, y), 10)
# 更新时间
t += 0.1
# 判断是否到达目标坐标
if x >= x_target and y <= y_target:
break
# 更新屏幕
pygame.display.update()
# 限制帧率
pygame.time.Clock().tick(30)
# 等待退出
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
```
阅读全文
相关推荐
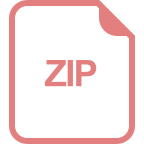
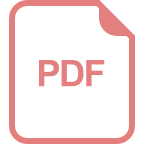

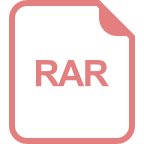
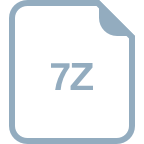
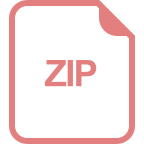
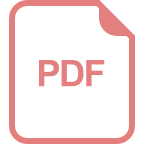
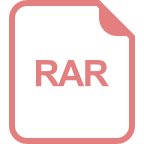
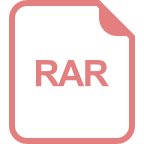
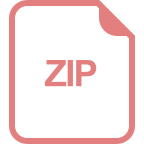
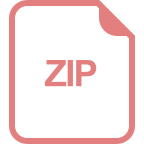
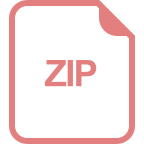
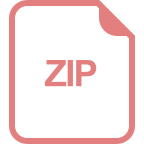
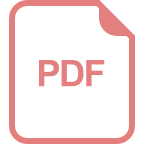
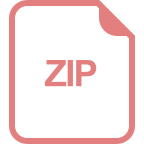
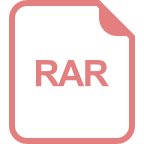
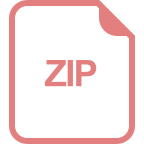