基于opencv开启摄像头检测追踪小球并给出小球坐标c++代码
时间: 2023-08-01 07:08:00 浏览: 135
好的,这里给你提供一个基于OpenCV的C++代码实现检测追踪小球并给出小球坐标的功能。
```
#include <opencv2/opencv.hpp>
using namespace cv;
int main()
{
// 打开摄像头
VideoCapture cap(0);
if (!cap.isOpened())
{
std::cout << "Failed to open the camera!" << std::endl;
return -1;
}
// 定义颜色范围
Scalar lower_red(0, 50, 50);
Scalar upper_red(10, 255, 255);
while (true)
{
// 读取帧
Mat frame;
cap >> frame;
// 将图像从BGR转换为HSV颜色空间
cvtColor(frame, frame, COLOR_BGR2HSV);
// 提取小球的颜色
Mat mask;
inRange(frame, lower_red, upper_red, mask);
// 查找小球的轮廓
std::vector<std::vector<Point>> contours;
std::vector<Vec4i> hierarchy;
findContours(mask, contours, hierarchy, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 找到最大的轮廓
if (contours.size() > 0)
{
int max_contour_index = 0;
double max_contour_area = 0;
for (int i = 0; i < contours.size(); i++)
{
double contour_area = contourArea(contours[i]);
if (contour_area > max_contour_area)
{
max_contour_index = i;
max_contour_area = contour_area;
}
}
// 找到小球的圆心和半径
Point2f center;
float radius;
minEnclosingCircle(contours[max_contour_index], center, radius);
// 在图像中标记出小球的位置
circle(frame, center, radius, Scalar(0, 255, 0), 2);
putText(frame, "Ball", Point(center.x - 10, center.y - 10), FONT_HERSHEY_SIMPLEX, 0.5, Scalar(0, 255, 0), 2);
// 输出小球的坐标
std::cout << "Ball position: (" << center.x << ", " << center.y << ")" << std::endl;
}
// 显示图像
imshow("frame", frame);
// 按下q键退出程序
if (waitKey(1) == 'q')
{
break;
}
}
// 释放资源
cap.release();
destroyAllWindows();
return 0;
}
```
这里的代码实现和前面的Python代码实现非常相似,只是语法不同。你可以参考一下这个C++代码,并根据自己的需要进行修改。
阅读全文
相关推荐
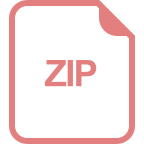
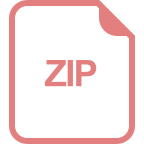
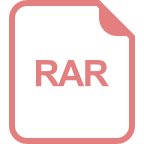















