C++面向对象贪吃蛇全部代码
时间: 2023-10-21 20:03:40 浏览: 58
好的,以下是一个简单的C++面向对象贪吃蛇游戏的完整代码:
```cpp
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
#include <conio.h>
using namespace std;
class Snake {
public:
vector<pair<int, int>> body;
int length;
int direction;
Snake(int x, int y, int len, int dir) {
length = len;
direction = dir;
for (int i = 0; i < len; i++) {
body.push_back(make_pair(x - i, y));
}
}
void move() {
pair<int, int> head = body[0];
switch (direction) {
case 0: // Up
head.second--;
break;
case 1: // Down
head.second++;
break;
case 2: // Left
head.first--;
break;
case 3: // Right
head.first++;
break;
default:
break;
}
body.insert(body.begin(), head);
body.pop_back();
}
bool is_dead(int width, int height) {
pair<int, int> head = body[0];
if (head.first < 0 || head.first >= width || head.second < 0 || head.second >= height) {
return true;
}
for (int i = 1; i < body.size(); i++) {
if (head == body[i]) {
return true;
}
}
return false;
}
void eat() {
pair<int, int> head = body[0];
switch (direction) {
case 0: // Up
head.second--;
break;
case 1: // Down
head.second++;
break;
case 2: // Left
head.first--;
break;
case 3: // Right
head.first++;
break;
default:
break;
}
body.insert(body.begin(), head);
length++;
}
};
class Food {
public:
pair<int, int> position;
Food() {
position = make_pair(0, 0);
}
void generate(int width, int height) {
srand(time(NULL));
position.first = rand() % width;
position.second = rand() % height;
}
};
class Game {
public:
int width;
int height;
Snake snake;
Food food;
Game(int w, int h) {
width = w;
height = h;
snake = Snake(width / 2, height / 2, 3, 3);
food = Food();
}
void init() {
snake = Snake(width / 2, height / 2, 3, 3);
food.generate(width, height);
}
void run() {
char key = ' ';
while (key != 'q') {
system("cls");
// Draw the map
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (i == 0 || i == height - 1 || j == 0 || j == width - 1) {
cout << "*";
} else if (snake.body[0] == make_pair(j, i)) {
cout << "O";
} else if (food.position == make_pair(j, i)) {
cout << "X";
} else {
bool is_body = false;
for (int k = 1; k < snake.body.size(); k++) {
if (snake.body[k] == make_pair(j, i)) {
cout << "o";
is_body = true;
break;
}
}
if (!is_body) {
cout << " ";
}
}
}
cout << endl;
}
// Handle user input
if (_kbhit()) {
key = _getch();
switch (key) {
case 'w':
if (snake.direction != 1) {
snake.direction = 0;
}
break;
case 's':
if (snake.direction != 0) {
snake.direction = 1;
}
break;
case 'a':
if (snake.direction != 3) {
snake.direction = 2;
}
break;
case 'd':
if (snake.direction != 2) {
snake.direction = 3;
}
break;
default:
break;
}
}
// Move the snake
snake.move();
// Check if the snake is dead
if (snake.is_dead(width, height)) {
cout << "Game over!" << endl;
break;
}
// Check if the snake eats the food
if (snake.body[0] == food.position) {
snake.eat();
food.generate(width, height);
}
// Sleep for a while
Sleep(100);
}
}
};
int main() {
Game game(20, 10);
game.init();
game.run();
return 0;
}
```
这个代码实现了一个基本的贪吃蛇游戏,包含了移动、判断死亡、吃到食物等功能。你可以根据需要进一步扩展和优化。
相关推荐
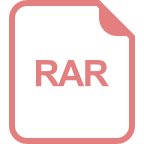















