用单链表表示一元多项式,并实现输入、输出、加、减运算。…… 要求实现: (1)创建多项式 (2)多项式加法 (3)输出多项式 (4)多项式减法 用c语言
时间: 2024-11-15 16:33:56 浏览: 23
在C语言中,我们可以使用结构体和指针来表示一元多项式。首先,我们需要定义一个结构体`Term`,它包含系数(coefficient)和指数(exponent):
```c
typedef struct {
int coefficient; // 系数
int exponent; // 指数
} Term;
```
接着,我们定义多项式的结构体`Polynomial`,并包含一个指向`Term`类型的指针数组和长度:
```c
typedef struct Polynomial {
Term* terms;
int degree; // 最大指数
int size; // 非零项的数量
} Polynomial;
```
接下来,分别实现各项功能:
1. **创建多项式** (构造函数)
```c
Polynomial* createPolynomial(int* coefficients, int degree) {
Polynomial* poly = malloc(sizeof(Polynomial));
poly->terms = malloc(degree * sizeof(Term));
for (int i = 0; i < degree; ++i) {
poly->terms[i].coefficient = coefficients[i];
poly->terms[i].exponent = i;
if (coefficients[i] != 0) {
poly->size++;
}
}
poly->degree = degree;
return poly;
}
```
2. **多项式加法**
```c
Polynomial* addPolynomials(Polynomial* a, Polynomial* b) {
if (a == NULL || b == NULL) return a; // 如果有一个是NULL,返回另一个
int maxDegree = MAX(a->degree, b->degree);
Polynomial* result = malloc(sizeof(Polynomial));
result->terms = malloc(maxDegree * sizeof(Term));
result->degree = maxDegree;
result->size = 0;
for (int i = 0; i <= maxDegree; ++i) {
Term termA = a->terms[i], termB = b->terms[i];
result->terms[result->size++] = (termA.coefficient + termB.coefficient) > 0 ?
{abs(termA.coefficient + termB.coefficient), i} : {0, i};
}
free(a->terms);
free(b->terms);
return result;
}
```
3. **输出多项式**
```c
void printPolynomial(Polynomial* poly) {
for (int i = 0; i <= poly->degree && poly->terms[i].coefficient != 0; ++i) {
printf("%d*x^%d ", poly->terms[i].coefficient, poly->terms[i].exponent);
}
printf("\n");
}
```
4. **多项式减法**
```c
Polynomial* subtractPolynomials(Polynomial* a, Polynomial* b) {
if (a == NULL || b == NULL) return a; // 如果有一个是NULL,返回另一个
Polynomial* result = malloc(sizeof(Polynomial));
result->terms = malloc(MAX(a->degree, b->degree) * sizeof(Term));
result->degree = MAX(a->degree, b->degree);
result->size = 0;
for (int i = 0; i <= a->degree && i <= b->degree; ++i) {
Term termA = a->terms[i], termB = b->terms[i];
result->terms[result->size++] = termA.coefficient - termB.coefficient > 0 ?
{termA.coefficient - termB.coefficient, i} : {0, i};
}
free(a->terms);
free(b->terms);
return result;
}
```
记得处理内存分配失败的情况,并在不需要时释放内存。
阅读全文
相关推荐
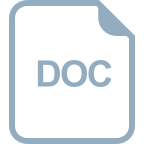
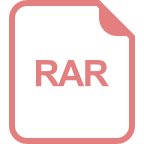
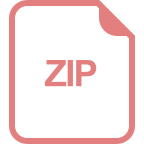



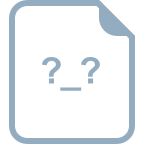
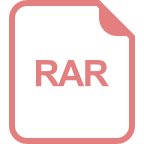
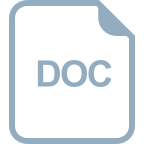






