``` dotProduct(self.normalized(),other.normalized())```修改这行代码
时间: 2024-09-14 16:05:05 浏览: 26
这行代码是计算两个向量的点积,其中`self.normalized()`和`other.normalized()`分别表示将当前对象(`self`)和其他对象(`other`)标准化后得到的单位向量。要修改这行代码,你可以根据需要调整向量的处理方式或计算其他类型的点积。
例如,如果你想计算两个向量的点积而不进行标准化,可以直接使用原始向量:
```python
dotProduct(self, other)
```
如果你想计算两个向量的点积,但在计算之前对它们进行其他处理,可以修改代码如下:
```python
# 假设有一个函数processVector用于处理向量
processed_self = processVector(self)
processed_other = processVector(other)
dotProduct(processed_self, processed_other)
```
如果你想要计算两个向量的点积,但只对其中一个向量进行标准化,可以这样写:
```python
dotProduct(self.normalized(), other)
```
请根据你的具体需求选择合适的修改方式。
相关问题
class LayerNorm(nn.Module): def __init__(self, normalized_shape, eps=1e-6, data_format="channels_last"): super(LayerNorm, self).__init__() self.weight = nn.Parameter(torch.ones(normalized_shape), requires_grad=True) self.bias = nn.Parameter(torch.zeros(normalized_shape), requires_grad=True) self.eps = eps self.data_format = data_format if self.data_format not in ['channels_last', 'channels_first']: raise ValueError(f"not support data format'{self.data_format}'") self.normalized_shape = (normalized_shape,) def forward(self, x: torch.Tensor) -> torch.Tensor: if self.data_format == 'channels_last': return F.layer_norm(x, self.normalized_shape, self.weight, self.bias, self.eps) elif self.data_format == 'channels_first': # [B,C,H,W] mean = x.mean(1, keepdim=True) var = (x - mean).pow(2).mean(1, keepdim=True) x = (x - mean) / torch.sqrt(var + self.eps) x = self.weight[:, None, None] * x + self.bias[:, None, None] return x
这段代码实现了Layer Normalization(层归一化)的功能。Layer Normalization是一种用于神经网络的归一化方法,可以在训练过程中稳定神经网络的学习。
在代码中,LayerNorm类继承自nn.Module,并实现了初始化方法和前向传播方法。
在初始化方法中,normalized_shape参数指定了归一化的维度大小,eps参数用于防止除零错误,data_format参数用于指定输入数据的格式('channels_last'或'channels_first')。
前向传播方法中,根据输入数据的格式进行不同的处理。当data_format为'channels_last'时,调用了PyTorch中的F.layer_norm函数进行归一化操作。当data_format为'channels_first'时,首先计算输入数据的均值和方差,然后进行归一化操作,并使用参数weight和bias进行缩放和偏移。
最后返回归一化后的结果。
class ImageViewer(QGraphicsView): def init(self, parent=None): super().init(parent) self.setScene(QGraphicsScene(self)) self.image_item = QGraphicsPixmapItem() self.select_rect_item = SelectRectItem() self.scene().addItem(self.image_item) self.scene().addItem(self.select_rect_item) self.start_point = None self.end_point = None def set_image(self, image): pixmap = QPixmap.fromImage(image) self.image_item.setPixmap(pixmap) self.setSceneRect(pixmap.rect()) def mousePressEvent(self, event): if event.button() == Qt.LeftButton: self.start_point = self.mapToScene(event.pos()) self.select_rect_item.set_rect(QRectF(self.start_point, QSizeF())) event.accept() else: super().mousePressEvent(event) def mouseReleaseEvent(self, event): if event.button() == Qt.LeftButton: self.end_point = self.mapToScene(event.pos()) rect = QRectF(self.start_point, self.end_point).normalized() x, y, w, h = rect.getRect() print("Selected rectangle: ({}, {}, {}, {})".format(x, y, w, h)) self.select_rect_item.setVisible(False) event.accept() else: super().mouseReleaseEvent(event)),每一行代码是什么意思
这段代码实现了一个基于 QGraphicsView 的图像查看器,可以用鼠标框选图像上的区域,并输出所选区域的坐标。
1. `class ImageViewer(QGraphicsView):`:定义了一个名为 ImageViewer 的类,继承自 QGraphicsView。
2. `def __init__(self, parent=None):`:定义了类的构造方法。
3. `super().init(parent)`:调用父类 QGraphicsView 的构造方法。
4. `self.setScene(QGraphicsScene(self))`:创建一个 QGraphicsScene 对象,并将其设置为视图的场景。
5. `self.image_item = QGraphicsPixmapItem()`:创建一个 QGraphicsPixmapItem 对象,用于显示图像。
6. `self.select_rect_item = SelectRectItem()`:创建一个 SelectRectItem 对象,用于显示鼠标选框。
7. `self.scene().addItem(self.image_item)`:将图像对象添加到场景中。
8. `self.scene().addItem(self.select_rect_item)`:将选框对象添加到场景中。
9. `self.start_point = None`:用于记录鼠标按下时的位置。
10. `self.end_point = None`:用于记录鼠标释放时的位置。
11. `def set_image(self, image):`:定义了一个方法,用于设置要显示的图像。
12. `pixmap = QPixmap.fromImage(image)`:将 QImage 对象转换为 QPixmap 对象。
13. `self.image_item.setPixmap(pixmap)`:将 QPixmap 对象设置为图像对象的显示内容。
14. `self.setSceneRect(pixmap.rect())`:将场景范围设置为图像的大小。
15. `def mousePressEvent(self, event):`:定义了鼠标按下事件的处理方法。
16. `if event.button() == Qt.LeftButton:`:判断是否按下左键。
17. `self.start_point = self.mapToScene(event.pos())`:将鼠标点击位置转换为场景坐标,并保存为起始点位置。
18. `self.select_rect_item.set_rect(QRectF(self.start_point, QSizeF()))`:设置选框对象的位置大小为起始点位置和一个空的大小。
19. `event.accept()`:接受事件,使其不会被其他控件处理。
20. `else:`:如果按下的不是左键,则调用父类的方法处理该事件。
21. `def mouseReleaseEvent(self, event):`:定义了鼠标释放事件的处理方法。
22. `if event.button() == Qt.LeftButton:`:判断是否释放左键。
23. `self.end_point = self.mapToScene(event.pos())`:将鼠标释放位置转换为场景坐标,并保存为结束点位置。
24. `rect = QRectF(self.start_point, self.end_point).normalized()`:根据起始点和结束点计算出选框的位置大小。
25. `x, y, w, h = rect.getRect()`:获取选框的坐标和大小。
26. `print("Selected rectangle: ({}, {}, {}, {})".format(x, y, w, h))`:输出选框坐标和大小。
27. `self.select_rect_item.setVisible(False)`:将选框对象隐藏。
28. `event.accept()`:接受事件,使其不会被其他控件处理。
29. `else:`:如果释放的不是左键,则调用父类的方法处理该事件。
阅读全文
相关推荐
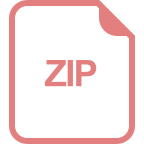
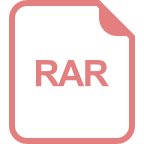
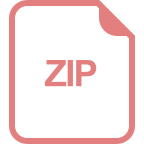














