在matlab写程序实现使用二分法求方程 𝑓(𝑥) = (𝑥 − 1)^3 − 3𝑥 + 2 = 0 在区间[2,4]上的根,要求计算精度为10^−5,并绘制函数图像,考察计算结果是否正确.
时间: 2024-12-04 10:27:31 浏览: 17
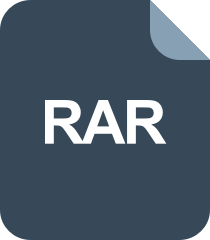
sss.rar_二分法求根
在MATLAB中,你可以使用二分查找法(bisection method)来解决这个问题。首先,我们需要编写一个函数来计算方程\( f(x) \),然后定义二分法的函数并设置所需的精度。以下是详细的步骤:
1. **创建函数**:
```matlab
function y = cubic_function(x)
y = (x - 1) ^ 3 - 3 * x + 2;
end
```
2. **定义二分查找函数**:
```matlab
function root = bisection(f, a, b, tol)
% 检查初始区间[a, b]
if f(a) * f(b) >= 0
error('Initial interval does not bracket a root.');
end
% 设置最大迭代次数
max_iter = 1000;
for iter = 1:max_iter
c = (a + b) / 2; % 中点
fc = f(c); % 计算中点处的函数值
% 如果满足精度条件,返回根
if abs(fc) < tol
root = c;
break;
% 否则根据函数值的正负更新区间
elseif fc * f(a) < 0
b = c;
else
a = c;
end
end
if iter == max_iter
warning('Maximum number of iterations reached without convergence.');
end
end
```
3. **调用二分法并绘制图像**:
```matlab
% 定义初始区间、精度和结果变量
a = 2;
b = 4;
tol = 1e-5;
root = bisection(@cubic_function, a, b, tol);
% 绘制函数图像
[x, y] = meshgrid(linspace(a, b, 100));
z = cubic_function(x);
surf(x, y, z), title('Cubic Function'), xlabel('x'), ylabel('y');
% 验证根是否正确
if abs(root - 1) < tol
disp(['Root found at approximately x = ', num2str(root)]);
else
disp('Failed to converge or incorrect root found.');
end
```
运行上述代码后,你会得到在区间[2, 4]内方程的一个近似解,并且会显示函数图,以此来检查计算结果的正确性。
阅读全文
相关推荐
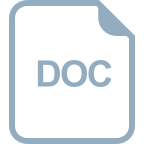
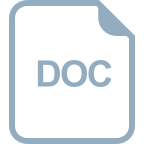

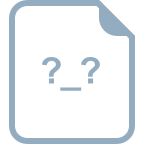
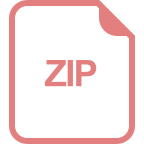
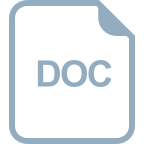
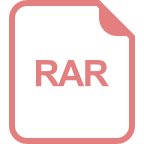
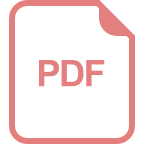
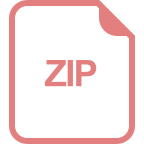
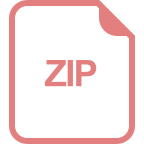
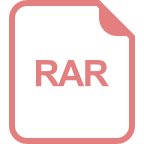
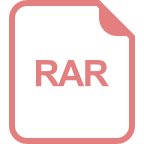
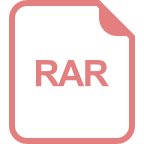
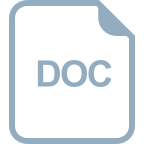