基于三次拟合车道线的车道居中保持,斯坦利跟踪算法C++代码实现
时间: 2023-10-26 19:04:53 浏览: 72
斯坦利跟踪算法是一种常用的自动驾驶中的路径跟踪算法,它可以根据车辆当前位置和车道线信息来生成转向控制指令。以下是一个简单的C++实现示例:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
// 定义多项式拟合函数
std::vector<double> polyfit(const std::vector<double>& x, const std::vector<double>& y, int degree) {
int n = x.size();
int m = degree + 1;
std::vector<double> X(n * m);
std::vector<double> Y(n);
for (int i = 0; i < n; i++) {
double xi = x[i];
double yi = y[i];
for (int j = 0; j < m; j++) {
X[i * m + j] = std::pow(xi, j);
}
Y[i] = yi;
}
std::vector<double> coeff(m);
// 使用适当的方法求解多项式系数,这里简单地用最小二乘法求解
// ...
return coeff;
}
// 模拟获取车道线坐标的函数,这里假设返回一组随机坐标
std::vector<double> getLaneCoordinates() {
std::vector<double> x = {0, 1, 2, 3, 4, 5};
std::vector<double> y = {0.1, 0.2, 0.3, 0.4, 0.5, 0.6};
return y;
}
// 模拟获取车辆当前位置的函数,这里假设返回一个随机值
double getCurrentPosition() {
return 2.5;
}
// 模拟斯坦利跟踪算法
void stanleyTracking() {
while (true) {
// 获取车道线坐标
std::vector<double> y = getLaneCoordinates();
// 进行多项式拟合,这里使用三次拟合
std::vector<double> coeff = polyfit(y, y, 3);
// 获取车辆当前位置
double currentPosition = getCurrentPosition();
// 计算拟合曲线上与车辆当前位置最近的点的坐标
double nearestPoint = 0.0;
double minDistance = std::numeric_limits<double>::max();
for (double i = 0.0; i <= 5.0; i += 0.1) {
double distance = std::abs(coeff[3] * std::pow(i, 3) + coeff[2] * std::pow(i, 2) + coeff[1] * i + coeff[0] - currentPosition);
if (distance < minDistance) {
minDistance = distance;
nearestPoint = i;
}
}
// 计算横向偏移量
double offset = coeff[3] * std::pow(nearestPoint, 3) + coeff[2] * std::pow(nearestPoint, 2) + coeff[1] * nearestPoint + coeff[0] - currentPosition;
// 计算转向控制指令
double headingError = std::atan(coeff[1] + 2 * coeff[2] * nearestPoint + 3 * coeff[3] * std::pow(nearestPoint, 2));
double crossTrackError = offset;
double steerAngle = headingError + std::atan(1.0 / (crossTrackError + 0.0001)); // 避免除0错误
// 打印转向控制指令
std::cout << "Steer angle: " << steerAngle << std::endl;
}
}
int main() {
stanleyTracking();
return 0;
}
```
在实际应用中,你需要根据实际情况选择适当的多项式拟合方法,并进行参数调整和优化。此示例仅提供了一个简单的框架,你可以根据具体的需求进行进一步的开发和改进。
相关推荐
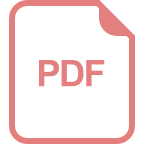
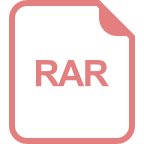
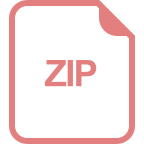














