假设n乘n的稀疏矩阵A采用三元组表示,设计一个c++程序实现以下功能: 生成两个稀疏矩阵三元组a和b;输出 三元组a的第一行第二列的元素值; 输出a转置矩阵的三元组; 输出a+b的三元组。
时间: 2024-12-10 19:04:10 浏览: 31
为了实现这个功能,我们首先需要定义一个稀疏矩阵的数据结构,通常会包含一个存储非零元素的数组(元素值)以及两个一维数组(分别对应行索引和列索引)。以下是简单的C++代码示例:
```cpp
#include <iostream>
#include <vector>
using namespace std;
struct SparseMatrixTriple {
int row;
int col;
double value;
};
// 创建一个稀疏矩阵类
class SparseMatrix {
private:
vector<SparseMatrixTriple> nonZeroElements;
int numRows;
int numCols;
public:
// 构造函数,初始化矩阵大小
SparseMatrix(int n) : numRows(n), numCols(n) {}
// 添加元素到矩阵
void addElement(int r, int c, double val) {
if (r >= 0 && r < numRows && c >= 0 && c < numCols) {
nonZeroElements.push_back({r, c, val});
}
}
// 获取第一行第二列的元素值
double getFirstRowSecondCol() const {
if (numRows > 0 && numCols > 0) {
for (const auto& elem : nonZeroElements) {
if (elem.row == 0 && elem.col == 1) {
return elem.value;
}
}
}
return 0; // 如果找不到,返回默认值
}
// 转置矩阵并返回转置后的三元组
vector<SparseMatrixTriple> transpose() const {
vector<SparseMatrixTriple> transposed;
for (const auto& elem : nonZeroElements) {
transposed.push_back({elem.col, elem.row, elem.value});
}
return transposed;
}
// 实现矩阵加法,这里假设矩阵的维度和非零元素相同
SparseMatrix operator+(const SparseMatrix& other) const {
SparseMatrix result(numRows, numCols);
for (const auto& elemA : nonZeroElements) {
for (const auto& elemB : other.nonZeroElements) {
if (elemA.row == elemB.row && elemA.col == elemB.col) {
result.addElement(elemA.row, elemA.col, elemA.value + elemB.value);
}
}
}
return result;
}
};
int main() {
// 生成两个稀疏矩阵
SparseMatrix a(5); // 假设n=5
a.addElement(0, 1, 4.2);
a.addElement(2, 3, 7.8);
// 其他操作
cout << "First row second column of A: " << a.getFirstRowSecondCol() << endl;
vector<SparseMatrixTriple> transposedA = a.transpose();
for (const auto& elem : transposedA) {
cout << "Transposed element: (" << elem.row << ", " << elem.col << ") = " << elem.value << endl;
}
// 矩阵相加
SparseMatrix b(5);
b.addElement(1, 2, 2.1);
SparseMatrix sum = a + b;
// 输出结果矩阵
cout << "Sum of A and B:\n";
for (const auto& elem : sum.nonZeroElements) {
cout << "(" << elem.row << ", " << elem.col << ") = " << elem.value << endl;
}
return 0;
}
```
在这个代码中,我们首先定义了一个稀疏矩阵的结构`SparseMatrixTriple`,然后在`SparseMatrix`类中实现了添加元素、获取特定位置的值、转置和矩阵相加的功能。在`main`函数里展示了如何创建矩阵并执行相应操作。
阅读全文
相关推荐
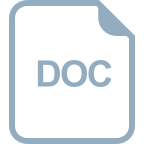
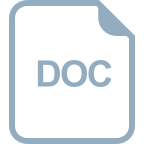
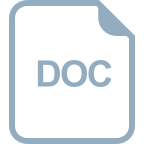

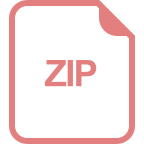
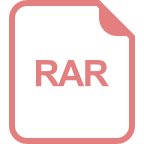
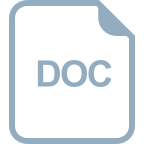
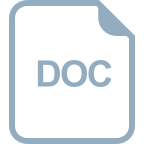
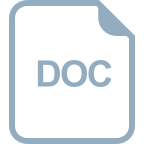








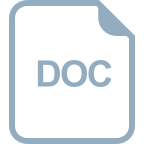
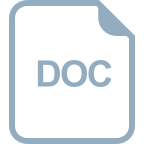