C++:分别使用贪心算法和动态规划算法写出代码和写出算法伪码:有n个集装箱要装上一搜重量为w的轮船,其中集装箱i(1<=i<=n)的重量为w,不考虑集装箱限制,现在要尽可能多的集装箱装上轮船,使得它们的重量之和不超过w
时间: 2024-12-24 21:17:56 浏览: 9
首先,让我们先看贪心算法的伪代码和C++代码。贪心策略是每次都选择当前剩余容量能容纳的最大重量的集装箱。
**贪心算法伪代码:**
```plaintext
function greedyCargoDistribution(weights, shipWeight):
sorted_weights = sort(weights, descending order)
total_weight = 0
result = []
for weight in sorted_weights:
if total_weight + weight <= shipWeight:
total_weight += weight
result.append(weight)
return result
```
**C++代码实现:**
```cpp
#include <vector>
#include <algorithm>
std::vector<int> greedyCargoDistribution(std::vector<int>& weights, int shipWeight) {
std::sort(weights.rbegin(), weights.rend()); // 从大到小排序
int total_weight = 0;
std::vector<int> result;
for (int weight : weights) {
if (total_weight + weight <= shipWeight) {
total_weight += weight;
result.push_back(weight);
}
}
return result;
}
```
然后,我们来看动态规划算法。这里我们可以创建一个二维数组dp,表示前i个箱子能分配的最大总重量,对于每个箱子,我们需要判断它是否应该加入到当前组合中以最大化总重量。
**动态规划算法伪代码:**
```plaintext
function dpCargoDistribution(weights, shipWeight):
dp = array of zeros with size (n+1) x (shipWeight+1)
dp[0][0] = 0
for i from 1 to n:
for j from 0 to shipWeight:
if weights[i-1] <= j:
dp[i][j] = max(dp[i-1][j], dp[i-1][j-weights[i-1]] + weights[i-1])
else:
dp[i][j] = dp[i-1][j]
return dp[n][shipWeight]
```
**C++代码实现:**
```cpp
#include <vector>
std::vector<int> dpCargoDistribution(std::vector<int>& weights, int shipWeight) {
std::vector<std::vector<int>> dp(weights.size() + 1, std::vector<int>(shipWeight + 1, 0));
dp[0][0] = 0;
for (size_t i = 1; i <= weights.size(); ++i) {
for (size_t j = 0; j <= shipWeight; ++j) {
if (weights[i - 1] <= j) {
dp[i][j] = std::max(dp[i - 1][j], dp[i - 1][j - weights[i - 1]] + weights[i - 1]);
} else {
dp[i][j] = dp[i - 1][j];
}
}
}
return {dp[weights.size()][shipWeight]};
}
```
阅读全文
相关推荐
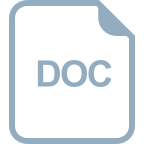
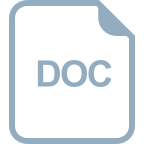
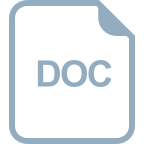















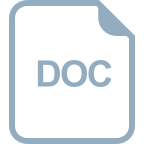