matlab最小外接矩形
时间: 2023-10-09 17:09:13 浏览: 285
在 Matlab 中,可以使用 `minboundrect` 函数来计算点集的最小外接矩形。
假设有一个点集 `P`,其中每个点的坐标为 `(x, y)`,则可以按照以下步骤计算最小外接矩形:
1. 使用 `convhull` 函数计算点集的凸包。凸包是能够包含点集所有点的最小凸多边形。
```
k = convhull(P);
```
2. 将凸包的边缘按逆时针方向排序。这一步非常重要,因为后面的计算需要按照逆时针方向进行。
```
k = convhull(P);
P = P(k,:);
```
3. 对于每个凸包的边缘,计算其法向量。这可以使用向量叉积来实现。
```
n = size(P, 1);
nrm = zeros(n, 2);
for i = 1:n
v1 = P(i,:);
v2 = P(mod(i, n)+1,:);
edge = v2 - v1;
nrm(i,:) = [-edge(2), edge(1)];
end
```
4. 对于每个法向量,计算点集在该法向量上的投影点集。
```
proj = P * nrm';
```
5. 对于每个法向量,计算点集在该法向量上的最小值和最大值。
```
[min_proj, min_idx] = min(proj);
[max_proj, max_idx] = max(proj);
```
6. 对于每个法向量,计算点集在该法向量上的跨度(即最大值减去最小值)。
```
span = max_proj - min_proj;
```
7. 找到跨度最小的法向量,即为最小外接矩形的方向。
```
[~, dir_idx] = min(span);
dir = nrm(dir_idx,:);
```
8. 计算最小外接矩形的中心点。
```
center = mean(P);
```
9. 计算最小外接矩形的宽度和高度。
```
proj_dir = P * dir';
min_proj = min(proj_dir);
max_proj = max(proj_dir);
width = abs(max_proj - min_proj);
height = span(dir_idx);
```
10. 计算最小外接矩形的角度。
```
theta = atan2(dir(2), dir(1)) * 180 / pi;
```
最后,最小外接矩形的信息可以表示为一个结构体,包括中心点坐标、宽度、高度和角度。
完整的 Matlab 代码如下:
```
function rect = min_bounding_rect(P)
% Compute the minimum bounding rectangle of a set of points
% P: a n x 2 array of points
% Compute the convex hull
k = convhull(P);
P = P(k,:);
% Compute the normal vectors of the convex hull edges
n = size(P, 1);
nrm = zeros(n, 2);
for i = 1:n
v1 = P(i,:);
v2 = P(mod(i, n)+1,:);
edge = v2 - v1;
nrm(i,:) = [-edge(2), edge(1)];
end
% Compute the projection of the points onto each normal vector
proj = P * nrm';
% Compute the span of the points along each normal vector
[min_proj, min_idx] = min(proj);
[max_proj, max_idx] = max(proj);
span = max_proj - min_proj;
% Find the direction of the minimum span
[~, dir_idx] = min(span);
dir = nrm(dir_idx,:);
% Compute the center point of the minimum bounding rectangle
center = mean(P);
% Compute the width and height of the minimum bounding rectangle
proj_dir = P * dir';
min_proj = min(proj_dir);
max_proj = max(proj_dir);
width = abs(max_proj - min_proj);
height = span(dir_idx);
% Compute the angle of the minimum bounding rectangle
theta = atan2(dir(2), dir(1)) * 180 / pi;
% Construct the rectangle struct
rect.center = center;
rect.width = width;
rect.height = height;
rect.angle = theta;
end
```
阅读全文
相关推荐
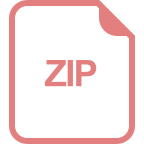



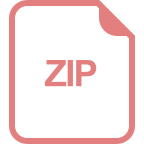
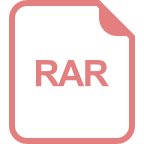
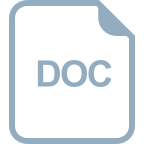
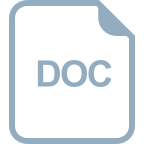






