public class MazeSolver { private int[][] maze; private boolean[][] visited; private int startRow, startCol, endRow, endCol; private int[] rowOffset = {-1, 0, 1, 0}; private int[] colOffset = {0, 1, 0, -1}; private Stack<int[]> path; public MazeSolver(int[][] maze, int startRow, int startCol, int endRow, int endCol) { this.maze = maze; this.visited = new boolean[maze.length][maze[0].length]; this.startRow = startRow; this.startCol = startCol; this.endRow = endRow; this.endCol = endCol; this.path = new Stack<>(); } public boolean solve() { visited[startRow][startCol] = true; path.push(new int[]{startRow, startCol}); if (dfs(startRow, startCol)) { System.out.println("找到通路:"); while (!path.isEmpty()) { int[] pos = path.pop(); System.out.println("(" + pos[0] + ", " + pos[1] + ")"); } return true; } else { System.out.println("没有通路"); return false; } } private boolean dfs(int row, int col) { if (row == endRow && col == endCol) { return true; } for (int i = 0; i < 4; i++) { int newRow = row + rowOffset[i]; int newCol = col + colOffset[i]; if (newRow >= 0 && newRow < maze.length && newCol >= 0 && newCol < maze[0].length && maze[newRow][newCol] == 0 && !visited[newRow][newCol]) { visited[newRow][newCol] = true; path.push(new int[]{newRow, newCol}); if (dfs(newRow, newCol)) { return true; } visited[newRow][newCol] = false; path.pop(); } } return false; } }这些代码是什么意思
时间: 2024-02-14 20:12:51 浏览: 144
这段代码是一个迷宫求解器的实现,它使用深度优先搜索算法来找到从起点到终点的路径。其中,迷宫由一个二维数组maze表示,0表示可以通行的地方,1表示障碍物;visited表示访问过的位置;startRow、startCol、endRow、endCol表示起点和终点的位置;path是一个栈,保存搜索过程中的路径。在solve方法中,首先将起点标记为已访问,并将起点压入路径栈中,然后调用dfs方法开始搜索。如果找到了终点,就依次弹出路径栈中的元素,输出路径,并返回true。如果搜索结束后仍未找到通路,返回false。dfs方法中,遍历当前位置四周的位置,如果该位置可以通行且未被访问过,则标记为已访问,将该位置压入路径栈中,继续递归搜索。如果找到通路,返回true,否则回溯到上一个位置,将该位置标记为未访问,弹出路径栈中的元素,并继续搜索其他方向。
相关问题
用Java语言写走迷宫代码
我可以回答这个问题。以下是一个简单的Java代码示例,用于解决迷宫问题:
```
public class MazeSolver {
private int[][] maze;
private boolean[][] visited;
private int startRow, startCol, endRow, endCol;
public MazeSolver(int[][] maze, int startRow, int startCol, int endRow, int endCol) {
this.maze = maze;
this.visited = new boolean[maze.length][maze[0].length];
this.startRow = startRow;
this.startCol = startCol;
this.endRow = endRow;
this.endCol = endCol;
}
public boolean solve() {
return solve(startRow, startCol);
}
private boolean solve(int row, int col) {
if (row < 0 || row >= maze.length || col < 0 || col >= maze[0].length || maze[row][col] == 1 || visited[row][col]) {
return false;
}
visited[row][col] = true;
if (row == endRow && col == endCol) {
return true;
}
if (solve(row - 1, col) || solve(row + 1, col) || solve(row, col - 1) || solve(row, col + 1)) {
return true;
}
return false;
}
}
```
这个代码使用递归算法来解决迷宫问题。它接受一个迷宫数组,起点和终点的行列坐标作为参数,并返回一个布尔值,表示是否找到了一条通路。如果找到了通路,那么visited数组将记录所有已经访问过的位置。
java通过txt读取迷宫地图_java寻找迷宫路径的简单实现示例
以下是一个通过txt文件读取迷宫地图并寻找路径的简单示例。该示例使用深度优先搜索算法来搜索迷宫路径。
1. 创建迷宫地图txt文件
首先,我们需要创建一个包含迷宫地图的txt文件。在该文件中,使用0表示可以通过的空格,使用1表示墙壁或障碍物。例如,以下是一个5x5的迷宫地图示例:
```
0 1 0 0 0
0 1 0 1 0
0 0 0 0 0
0 1 1 1 0
0 0 0 1 0
```
2. 读取迷宫地图文件
我们可以使用Java的File和Scanner类来读取迷宫地图文件。以下是一个读取迷宫地图文件并将其存储在二维数组中的示例代码:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class Maze {
private int[][] maze;
private int startRow;
private int startCol;
private int endRow;
private int endCol;
public Maze(String fileName) throws FileNotFoundException {
Scanner scanner = new Scanner(new File(fileName));
int numRows = 0;
int numCols = 0;
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
numCols = line.length() / 2 + 1;
numRows++;
}
scanner.close();
maze = new int[numRows][numCols];
scanner = new Scanner(new File(fileName));
int row = 0;
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] tokens = line.split(" ");
for (int col = 0; col < tokens.length; col++) {
maze[row][col] = Integer.parseInt(tokens[col]);
if (maze[row][col] == 2) {
startRow = row;
startCol = col;
} else if (maze[row][col] == 3) {
endRow = row;
endCol = col;
}
}
row++;
}
scanner.close();
}
}
```
在这个示例中,我们首先读取文件以确定迷宫地图的大小,然后创建一个二维数组来存储地图。我们还跟踪了起点和终点的位置。
3. 搜索迷宫路径
接下来,我们需要实现一个搜索算法来找到从起点到终点的路径。在这个示例中,我们使用深度优先搜索算法。
```java
public class MazeSolver {
private Maze maze;
private boolean[][] visited;
private int[][] directions = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}};
public MazeSolver(Maze maze) {
this.maze = maze;
visited = new boolean[maze.getNumRows()][maze.getNumCols()];
}
public void solve() {
if (dfs(maze.getStartRow(), maze.getStartCol())) {
System.out.println("Path found!");
} else {
System.out.println("No path found.");
}
}
private boolean dfs(int row, int col) {
if (row < 0 || row >= maze.getNumRows() || col < 0 || col >= maze.getNumCols()) {
return false;
}
if (visited[row][col]) {
return false;
}
if (maze.getMaze()[row][col] == 1) {
return false;
}
if (row == maze.getEndRow() && col == maze.getEndCol()) {
return true;
}
visited[row][col] = true;
for (int[] direction : directions) {
int newRow = row + direction[0];
int newCol = col + direction[1];
if (dfs(newRow, newCol)) {
return true;
}
}
return false;
}
}
```
在这个示例中,我们首先创建一个visited数组来跟踪哪些格子已经被访问过。然后我们从起点开始进行深度优先搜索。对于每一个格子,我们检查它是否可达、是否已经被访问过、是否是墙壁,并尝试向四个方向移动。如果我们找到了终点,我们返回true,否则返回false。
4. 完整代码
下面是完整的Java代码,包括Maze和MazeSolver类:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class Maze {
private int[][] maze;
private int startRow;
private int startCol;
private int endRow;
private int endCol;
public Maze(String fileName) throws FileNotFoundException {
Scanner scanner = new Scanner(new File(fileName));
int numRows = 0;
int numCols = 0;
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
numCols = line.length() / 2 + 1;
numRows++;
}
scanner.close();
maze = new int[numRows][numCols];
scanner = new Scanner(new File(fileName));
int row = 0;
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] tokens = line.split(" ");
for (int col = 0; col < tokens.length; col++) {
maze[row][col] = Integer.parseInt(tokens[col]);
if (maze[row][col] == 2) {
startRow = row;
startCol = col;
} else if (maze[row][col] == 3) {
endRow = row;
endCol = col;
}
}
row++;
}
scanner.close();
}
public int[][] getMaze() {
return maze;
}
public int getNumRows() {
return maze.length;
}
public int getNumCols() {
return maze[0].length;
}
public int getStartRow() {
return startRow;
}
public int getStartCol() {
return startCol;
}
public int getEndRow() {
return endRow;
}
public int getEndCol() {
return endCol;
}
}
public class MazeSolver {
private Maze maze;
private boolean[][] visited;
private int[][] directions = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}};
public MazeSolver(Maze maze) {
this.maze = maze;
visited = new boolean[maze.getNumRows()][maze.getNumCols()];
}
public void solve() {
if (dfs(maze.getStartRow(), maze.getStartCol())) {
System.out.println("Path found!");
} else {
System.out.println("No path found.");
}
}
private boolean dfs(int row, int col) {
if (row < 0 || row >= maze.getNumRows() || col < 0 || col >= maze.getNumCols()) {
return false;
}
if (visited[row][col]) {
return false;
}
if (maze.getMaze()[row][col] == 1) {
return false;
}
if (row == maze.getEndRow() && col == maze.getEndCol()) {
return true;
}
visited[row][col] = true;
for (int[] direction : directions) {
int newRow = row + direction[0];
int newCol = col + direction[1];
if (dfs(newRow, newCol)) {
return true;
}
}
return false;
}
}
public class Main {
public static void main(String[] args) throws FileNotFoundException {
Maze maze = new Maze("maze.txt");
MazeSolver solver = new MazeSolver(maze);
solver.solve();
}
}
```
注意,这个示例只是一个简单的迷宫问题的解决方案,更复杂的迷宫问题可能需要更高级的算法和数据结构来解决。
阅读全文
相关推荐
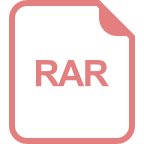
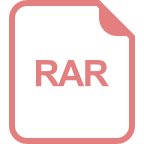
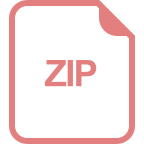











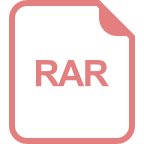
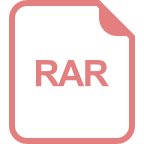
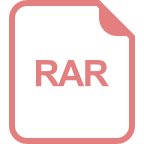