求 ax²+bx+c=0的根
时间: 2024-10-24 22:16:47 浏览: 25
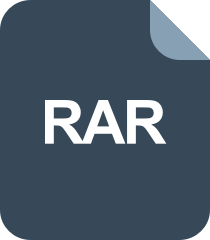
用Java编写程序,求一元二次方程ax²+bx+c=0的根(系数在程序中给定),并输出。
要求解一元二次方程 `ax^2 + bx + c = 0` 的根,我们可以使用著名的二次公式。该公式为:
\[ x = \frac{-b \pm \sqrt{b^2 - 4ac}}{2a} \]
其中 \( a \), \( b \), 和 \( c \) 是方程中的系数。这个公式适用于所有不等于零的 \( a \)。
如果你需要编写一段C代码来计算这个方程的根,你可以这样做:
```c
#include <stdio.h>
#include <math.h> // 引入数学库,用于sqrt()函数
// 函数声明,返回两个根
void calculate_roots(double a, double b, double c, double* roots);
int main() {
double a, b, c;
printf("Enter the coefficients (a, b, c): ");
scanf("%lf %lf %lf", &a, &b, &c);
if (a == 0) { // 如果a为0,方程变为线性,无法直接用二次公式
printf("This is not a quadratic equation.\n");
} else {
double roots[2]; // 存储两个根
calculate_roots(a, b, c, roots);
printf("The roots are:\n");
for (int i = 0; i < 2; i++) {
printf("Root %d: %.2f\n", i + 1, roots[i]);
}
}
return 0;
}
// 函数定义
void calculate_roots(double a, double b, double c, double* roots) {
double discriminant = b * b - 4 * a * c;
if (discriminant >= 0) {
roots[0] = (-b + sqrt(discriminant)) / (2 * a); // 正根
roots[1] = (-b - sqrt(discriminant)) / (2 * a); // 负根
} else {
roots[0] = roots[1] = -b / (2 * a); // 只有一个实根(重根)
printf("The root is real and repeated: %.2f\n", roots[0]);
}
}
```
在运行这段代码时,请确保用户输入的是有效的系数值。如果 \( a = 0 \),则方程变为线性,程序会提示这不是一个二次方程。
阅读全文
相关推荐
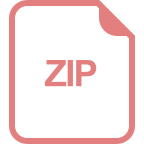















