:输入字符序列,建立二叉树;按先序、中序、后序遍历二叉树(三种递归算法和中序遍历的非递归算法)的代码示例
时间: 2023-10-23 09:20:26 浏览: 42
Sure! Here is an example code in Python for constructing a binary tree and traversing it using pre-order, in-order, and post-order recursive algorithms, as well as an iterative in-order traversal algorithm:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def buildTree(preorder, inorder):
if not inorder:
return None
root = TreeNode(preorder[0])
idx = inorder.index(root.val)
root.left = buildTree(preorder[1:idx+1], inorder[:idx])
root.right = buildTree(preorder[idx+1:], inorder[idx+1:])
return root
def preorderTraversal(root):
if not root:
return []
return [root.val] + preorderTraversal(root.left) + preorderTraversal(root.right)
def inorderTraversal(root):
if not root:
return []
return inorderTraversal(root.left) + [root.val] + inorderTraversal(root.right)
def postorderTraversal(root):
if not root:
return []
return postorderTraversal(root.left) + postorderTraversal(root.right) + [root.val]
def iterativeInorderTraversal(root):
res = []
stack = []
curr = root
while curr or stack:
while curr:
stack.append(curr)
curr = curr.left
curr = stack.pop()
res.append(curr.val)
curr = curr.right
return res
# example usage
preorder = [1, 2, 4, 5, 3, 6]
inorder = [4, 2, 5, 1, 3, 6]
root = buildTree(preorder, inorder)
print(preorderTraversal(root)) # [1, 2, 4, 5, 3, 6]
print(inorderTraversal(root)) # [4, 2, 5, 1, 3, 6]
print(postorderTraversal(root)) # [4, 5, 2, 6, 3, 1]
print(iterativeInorderTraversal(root)) # [4, 2, 5, 1, 3, 6]
```
I hope you find this helpful!
相关推荐
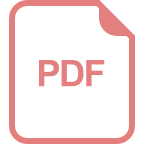














