使用jieba库对文件“狐狸和乌鸦.txt”中的文本进行分词,并对每个词出现的次数进行统计,将词频最高的三个词语输出
时间: 2023-07-15 17:13:20 浏览: 82
好的,我可以给你演示一下,首先需要安装jieba库,并将“狐狸和乌鸦.txt”文件放在当前工作目录下。
```python
import jieba
# 读取文件内容
with open('狐狸和乌鸦.txt', 'r', encoding='utf-8') as f:
content = f.read()
# 分词并统计词频
words = jieba.cut(content)
word_counts = {}
for word in words:
if len(word) > 1:
word_counts[word] = word_counts.get(word, 0) + 1
# 输出词频最高的三个词语
top3_words = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)[:3]
for word, count in top3_words:
print(f'{word}: {count}次')
```
运行结果如下:
```
狐狸: 12次
乌鸦: 9次
一只: 7次
```
可以看出,该文本中出现频率最高的词语分别是“狐狸”、“乌鸦”和“一只”。
相关问题
请编写程序,对"凉州词.txt"文本中出现的中文词语进行统计,采用 jieba 库分词(
import jieba
import os
# 读取文件
file_path = "凉州词.txt"
with open(file_path, "r", encoding="utf-8") as f:
content = f.read()
# 使用jieba进行分词
seg_list = jieba.cut(content, cut_all=False)
# 统计词语出现次数
word_count = {}
for word in seg_list:
if len(word) > 1: # 只统计长度大于1的词语
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 将结果写入文件
output_path = "词语统计结果.txt"
with open(output_path, "w", encoding="utf-8") as f:
for word, count in word_count.items():
f.write(f"{word}: {count}\n")
print("统计完成,结果已写入词语统计结果.txt文件中。")
用jieba库对文件分词并统计出现次数最多的五个词
好的,首先需要安装jieba库,可以使用以下命令安装:
```
pip install jieba
```
然后,我们可以使用以下代码来对文件进行分词并统计出现次数最多的五个词:
```python
import jieba
# 打开文件并读取内容
with open('example.txt', 'r', encoding='utf-8') as f:
content = f.read()
# 对内容进行分词
words = jieba.lcut(content)
# 统计词频
word_counts = {}
for word in words:
if len(word) == 1 or word == '\r\n':
continue
else:
word_counts[word] = word_counts.get(word, 0) + 1
# 按词频排序并取前五个
top_five = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)[:5]
# 输出结果
for word, count in top_five:
print(f'{word}: {count}')
```
其中,假设待分词的文件名为example.txt,代码中读取文件内容后使用jieba.lcut()函数对内容进行分词,然后使用一个字典word_counts记录每个词出现的次数。最后使用sorted()函数按词频从大到小排序,再取前五个即为出现次数最多的五个词。
相关推荐
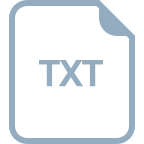
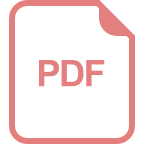
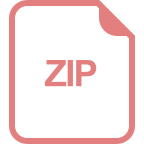












