isomap数据降维matlab代码
时间: 2023-10-24 15:31:02 浏览: 81
Sure! Here is an example of Isomap dimensionality reduction implemented in MATLAB:
```matlab
% Load your data matrix here
data = load('your_data.mat');
% Determine the number of nearest neighbors
k = 10;
% Compute pairwise distances using Euclidean distance
distances = pdist2(data, data);
% Find the k-nearest neighbors for each point
[~, nearest_neighbors] = sort(distances, 2);
nearest_neighbors = nearest_neighbors(:, 2:k+1);
% Create the neighborhood graph
graph = zeros(size(data, 1));
for i = 1:size(data, 1)
for j = nearest_neighbors(i, :)
graph(i, j) = distances(i, j);
end
end
% Apply Floyd-Warshall algorithm to find the shortest path distances
shortest_distances = graph;
for k = 1:size(data, 1)
for i = 1:size(data, 1)
for j = 1:size(data, 1)
if shortest_distances(i, k) + shortest_distances(k, j) < shortest_distances(i, j)
shortest_distances(i, j) = shortest_distances(i, k) + shortest_distances(k, j);
end
end
end
end
% Compute the Gram matrix
squared_distances = shortest_distances.^2;
gram_matrix = -0.5 * (squared_distances - ...
sum(squared_distances, 1)/size(data, 1) - ...
sum(squared_distances, 2)/size(data, 1) + ...
sum(squared_distances(:))/(size(data, 1)^2));
% Perform singular value decomposition
[V, D] = eig(gram_matrix);
[~, sorted_indices] = sort(diag(D), 'descend');
D_sorted = D(sorted_indices, sorted_indices);
V_sorted = V(:, sorted_indices);
% Choose the number of dimensions for the reduced space
num_dimensions = 2;
% Compute the final lower-dimensional representation
reduced_data = V_sorted(:, 1:num_dimensions) * sqrt(D_sorted(1:num_dimensions, 1:num_dimensions));
% Plot the reduced data
scatter(reduced_data(:, 1), reduced_data(:, 2));
title('Isomap Dimensionality Reduction');
xlabel('Dimension 1');
ylabel('Dimension 2');
```
Make sure to replace `'your_data.mat'` with the path to your actual data file. Also, feel free to adjust the parameters such as the number of nearest neighbors (`k`) and the number of dimensions in the reduced space (`num_dimensions`) according to your needs.
相关推荐
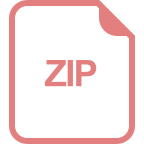
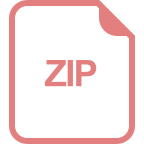














