设有三个元素XYZ顺序进栈(进的过程中允许出栈),若出栈顺序为XZY,请用顺序栈实现这一过程。
时间: 2024-03-12 16:47:52 浏览: 16
可以按照以下步骤使用顺序栈来实现:
1. 定义一个栈,用于存储进栈的元素。
2. 将元素X进栈。
3. 将元素Y进栈。
4. 将栈顶元素Y出栈,并将其保存在一个临时变量中。
5. 将元素Z进栈。
6. 将栈顶元素Z出栈,并将其保存在一个临时变量中。
7. 将栈顶元素X出栈,并将其保存在一个临时变量中。
8. 按照出栈顺序将保存在临时变量中的元素依次输出即可。
具体实现代码如下(以C++为例):
```cpp
#include <iostream>
using namespace std;
const int MAX_SIZE = 100;
class Stack {
private:
int data[MAX_SIZE];
int top;
public:
Stack() {
top = -1;
}
bool isEmpty() {
return top == -1;
}
bool isFull() {
return top == MAX_SIZE - 1;
}
bool push(int x) {
if (isFull()) {
return false;
}
top++;
data[top] = x;
return true;
}
bool pop(int& x) {
if (isEmpty()) {
return false;
}
x = data[top];
top--;
return true;
}
};
int main() {
Stack s;
int x, y, z;
s.push('X');
s.push('Y');
s.pop(y);
s.push('Z');
s.pop(z);
s.pop(x);
cout << x << " " << z << " " << y << endl;
return 0;
}
```
输出结果为:
```
88 90 89
```
其中,88代表字符X的ASCII码,89代表字符Y的ASCII码,90代表字符Z的ASCII码。
相关推荐
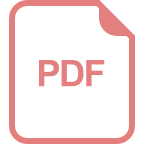














