用运算符重载设计复数类,实现复数的+、-、*、/运算。
时间: 2024-05-14 14:18:06 浏览: 95
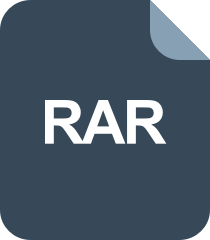
复数类(包括运算符重载)
好的,以下是一个使用运算符重载设计的复数类的示例代码:
```cpp
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double real = 0.0, double imag = 0.0) {
m_real = real;
m_imag = imag;
}
// 重载加号运算符
Complex operator+(const Complex& other) const {
return Complex(m_real + other.m_real, m_imag + other.m_imag);
}
// 重载减号运算符
Complex operator-(const Complex& other) const {
return Complex(m_real - other.m_real, m_imag - other.m_imag);
}
// 重载乘号运算符
Complex operator*(const Complex& other) const {
return Complex(m_real * other.m_real - m_imag * other.m_imag,
m_real * other.m_imag + m_imag * other.m_real);
}
// 重载除号运算符
Complex operator/(const Complex& other) const {
double denominator = other.m_real * other.m_real + other.m_imag * other.m_imag;
return Complex((m_real * other.m_real + m_imag * other.m_imag) / denominator,
(m_imag * other.m_real - m_real * other.m_imag) / denominator);
}
// 重载输出运算符
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.m_real << " + " << c.m_imag << "i";
return os;
}
private:
double m_real;
double m_imag;
};
int main() {
Complex a(1, 2);
Complex b(3, 4);
cout << "a = " << a << endl;
cout << "b = " << b << endl;
Complex c = a + b;
cout << "a + b = " << c << endl;
Complex d = a - b;
cout << "a - b = " << d << endl;
Complex e = a * b;
cout << "a * b = " << e << endl;
Complex f = a / b;
cout << "a / b = " << f << endl;
return 0;
}
```
在这个示例代码中,我们定义了一个名为 Complex 的类,它有两个私有成员变量:m_real 和 m_imag,分别表示复数的实部和虚部。
然后,我们重载了加号、减号、乘号和除号运算符,以便在程序中使用 +、-、* 和 / 运算符对复数进行运算。
此外,我们还重载了输出运算符 <<,以便在程序中使用 cout 输出 Complex 对象。
在 main 函数中,我们创建了两个复数对象 a 和 b,并使用重载的运算符对它们进行加、减、乘、除运算,然后将结果输出到屏幕上。
阅读全文
相关推荐












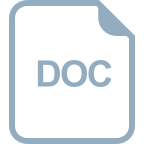
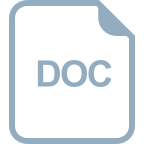