设计一个complex类,里面有成员变量实部虚部,成员函数+-×/,利用运算符的重载实现
时间: 2024-09-23 12:12:30 浏览: 56
设计一个名为`ComplexNumber`的复杂类,可以包含两个成员变量`real`和`imaginary`分别代表复数的实部和虚部。为了支持复数的加减乘除运算,我们可以实现`+`、`-`、`*`和`/`运算符的重载。
```cpp
class ComplexNumber {
private:
double real;
double imaginary;
public:
// 构造函数
ComplexNumber(double r = 0.0, double i = 0.0) : real(r), imaginary(i) {}
// 获取和设置实部和虚部的getter和setter方法
double getReal() const { return real; }
double getImaginary() const { return imaginary; }
void setReal(double r) { real = r; }
void setImaginary(double i) { imaginary = i; }
// 运算符重载实现基本的四则运算
ComplexNumber operator+(const ComplexNumber& other) const {
double newReal = real + other.real;
double newImaginary = imaginary + other.imaginary;
return ComplexNumber(newReal, newImaginary);
}
ComplexNumber operator-(const ComplexNumber& other) const {
double newReal = real - other.real;
double newImaginary = imaginary - other.imaginary;
return ComplexNumber(newReal, newImaginary);
}
ComplexNumber operator*(const ComplexNumber& other) const {
double newReal = real * other.real - imaginary * other.imaginary;
double newImaginary = real * other.imaginary + imaginary * other.real;
return ComplexNumber(newReal, newImaginary);
}
// 复数除法需要处理除数为零的情况,这里简化处理
// 真正的除法需要考虑复数除法规则,这里仅示例非除零情况
ComplexNumber operator/(const ComplexNumber& other) const {
double denominator = other.real * other.real + other.imaginary * other.imaginary;
if (denominator != 0) {
double newReal = (real * other.real + imaginary * other.imaginary) / denominator;
double newImaginary = (imaginary * other.real - real * other.imaginary) / denominator;
return ComplexNumber(newReal, newImaginary);
} else {
throw std::runtime_error("Division by zero is not allowed.");
}
}
};
阅读全文
相关推荐


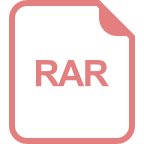















