用python按以下要求写出代码,Suppose there are n assignments A= [a1, a2 …ai …an]that you need to complete before the deadline. An assignment ai= [durationi, deadlinei] need durationi days to complete and must be completed before or on deadlinei. You can only do one assignment at a time and start next assignment once the current assignment is completed. Assuming you start on day 1, implement an efficient algorithm to find the maximum number of assignments you can complete.
时间: 2023-04-09 14:03:21 浏览: 107
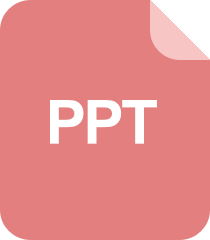
You_are_supposed_to_shake_hands课件2_精美学习课件ppt
可以回答这个问题。以下是Python代码:
def max_assignments(A):
A = sorted(A, key=lambda x: x[1])
current_day = 1
count = 0
for duration, deadline in A:
if current_day + duration - 1 <= deadline:
count += 1
current_day += duration
return count
# 测试
A = [[2, 4], [3, 5], [1, 2], [4, 7], [1, 1]]
print(max_assignments(A)) # 输出3,最多可以完成3个作业:[1, 2], [2, 4], [1, 1]
阅读全文