问题描述:已知递增有序的单链表A,编写算法实现向A中插入或删除一个元素,并保持A的有序性。 实验要求: 1、结点的数据均为整型。 2、若表中已经存在此元素,则不插入
时间: 2024-10-16 17:17:20 浏览: 33
要实现这个功能,我们需要考虑两种情况:插入新元素和删除指定元素。
**插入操作:**
1. 首先,遍历链表A,找到第一个大于等于待插入值的节点。
2. 将新元素插入到该节点之前,如果该节点不存在(即链表为空或所有节点都小于待插入值),则将新元素作为头节点添加。
```c
struct Node {
int data;
struct Node* next;
};
void insert(struct Node** head, int value) {
if (*head == NULL || value > (*head)->data) {
// 如果链表为空或待插入值比当前头节点大
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = *head;
*head = newNode; // 更新头节点
} else {
struct Node* current = *head;
while (current->next != NULL && current->next->data < value) {
current = current->next;
}
// 插入位置确定后,创建新节点并连接它们
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
}
}
```
**删除操作:**
1. 遍历链表,找到第一个等于待删除值的节点(如果存在)。
2. 如果找到了,将找到的节点的前一个节点的`next`指针指向其下一个节点;如果没有找到(即链表中没有该值),则不做任何操作。
```c
void remove(struct Node** head, int value) {
if (*head != NULL) {
struct Node* current = *head;
struct Node* prev = NULL;
// 查找要删除的节点
while (current != NULL && current->data != value) {
prev = current;
current = current->next;
}
// 删除节点
if (current != NULL) {
if (prev == NULL) { // 待删除的是头节点
*head = current->next;
} else {
prev->next = current->next;
}
free(current); // 释放内存
}
}
}
```
阅读全文
相关推荐
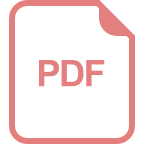
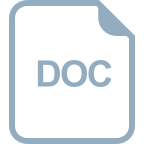
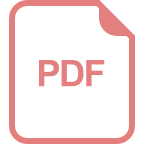




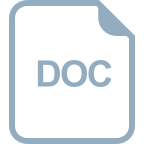










