stm32f103c8t6通过编码器测速的代码,标准库
时间: 2024-10-18 11:02:27 浏览: 87
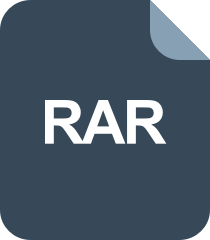
stm32f103c8t6智能小车红外循迹避障实验程序源代码.rar

在STM32F103C8T6单片机上通过编码器进行速度测量,通常需要连接两个方向的编码器脉冲(例如A、B相),然后通过计数的方式计算编码器转过的圈数。这里假设你使用的是霍尔编码器,因为它的信号可以直接输入到单片机的输入捕获单元。下面是一个基础的示例代码:
```c
#include "stm32f10x.h" // 使用STM32标准库
// 编码器通道配置
#define ENCODER_PIN_A GPIO_Pin_0
#define ENCODER_PIN_B GPIO_Pin_1
#define ENCODER_GPIO_PORT GPIOB
// 输入捕获寄存器配置
#define CAPTURE_CHANNEL 1
#define CAPTURE_MAX_COUNT (4095) // 对于10位编码器,最大值减一
void TIM_Configuration(void) {
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef.TIM_TimeBaseStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_TIM2, ENABLE); // 启动TIM2时钟
GPIO_InitStructure.GPIO_Pin = ENCODER_PIN_A | ENCODER_PIN_B;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(ENCODER_GPIO_PORT, &GPIO_InitStructure);
TIM_InitStructure.TIM_Prescaler = (SystemCoreClock / 1000000) - 1;
TIM_InitStructure.TIM_ClockDivision = 0;
TIM_InitStructure.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM2, &TIM_InitStructure);
TIM_ITConfig(TIM2, TIM_IT_Update, ENABLE);
TIM_Cmd(TIM2, ENABLE);
}
uint16_t GetEncoderSpeed(void) {
uint16_t current_count = TIM_GetCaptureCount(TIM_CHANNEL(CAPTURE_CHANNEL)); // 获取当前计数值
uint16_t last_count = TIM_Get CaptureCount(TIM_CHANNEL(CAPTURE_CHANNEL)); // 假设之前已经保存过一次计数
if (current_count > last_count) { // 判断是否转动了编码器
return ((current_count - last_count) * SystemCoreClock / (CAPTURE_MAX_COUNT * 2)); // 计算转速,单位:Hz
} else {
return 0; // 如果没转动,则返回0
}
}
int main(void) {
TIM_Configuration();
while (1) {
float speed = GetEncoderSpeed();
printf("Encoder Speed: %.2f RPM\n", speed); // 输出速度
// ... 其他处理和显示代码
}
return 0;
}
```
阅读全文
相关推荐
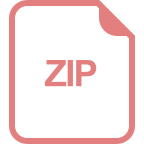
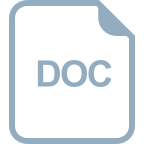
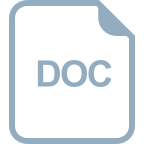
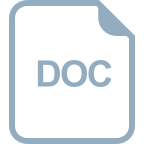





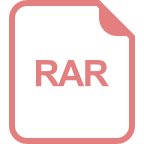
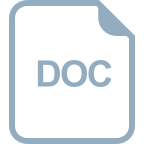
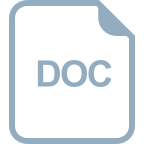





