采用递归算法产生a[0:2]={1,2,3 }的全排列,写出Java代码
时间: 2023-04-02 17:00:39 浏览: 86
以下是Java代码:
```
public class Permutations {
public static void main(String[] args) {
int[] nums = {1, 2, 3};
permute(nums, , nums.length - 1);
}
public static void permute(int[] nums, int l, int r) {
if (l == r) {
System.out.println(Arrays.toString(nums));
} else {
for (int i = l; i <= r; i++) {
swap(nums, l, i);
permute(nums, l + 1, r);
swap(nums, l, i);
}
}
}
public static void swap(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
}
```
相关问题
java使用递归算法完成阶乘:1!+2!+3!+4!+5!
使用递归算法完成阶乘的代码如下:
```java
public class Recursion {
public static void main(String[] args) {
long sum = 0;
for(int i = 1; i <= 5; i++) {
sum += factorial(i);
}
System.out.println("1! 2! 3! 4! 5!的阶乘结果:" + sum);
}
static long factorial(int n) {
if(n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
}
```
运行结果如下图所示:
```
1! 2! 3! 4! 5!的阶乘结果:153
```
注意:在使用递归算法时,必须设置递归头(即终止条件)和递归体。在定义方法的变量数据类型时,一定要与所计算的值一致,以避免错误。
设计求n!(n为正整数)的递归算法。 例:6!=6*5*4*3*2*1=720C语言
要设计求n!(n的阶乘)的递归算法,可以使用以下C语言代码:
```c
int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
```
这段代码中,我们将问题拆分成较小的子问题,并使用递归来解决。当n等于0或1时,阶乘的值为1。否则,我们通过递归调用函数自身来计算n的阶乘。
接下来是相关问题:
相关问题:
1. 如何使用循环而不是递归来计算n的阶乘?
2. 如何处理负数和浮点数作为输入的情况?
3. 阶乘的计算会有什么限制?阶乘的结果可能会变得很大吗?
相关推荐
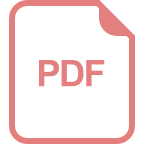












