用C语言简单编写一个程序:已知一个顺序表A,请设计一个算法,将A分解为两个顺序表B,C,其中顺序表B中的值是A中小于0的元素,C中的值是A中大于0的元素。
时间: 2024-10-07 12:01:20 浏览: 32
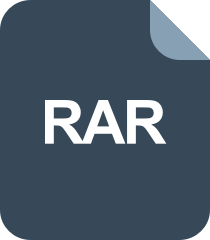
c语言数据结构顺序表学习
在C语言中,你可以通过遍历顺序表A并利用指针来完成这个任务。这里提供一个简单的示例程序:
```c
#include <stdio.h>
// 定义顺序表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* create_node(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 分解顺序表
void split_list(Node* A, Node** B, Node** C) {
*B = NULL; // 初始化B链表头
*C = NULL; // 初始化C链表头
Node* current = A;
while (current != NULL) {
if (current->data < 0) { // 如果当前元素小于0,添加到B链表
if (*B == NULL) {
*B = current;
} else {
(*B)->next = current;
}
*B = current; // 更新B链表头
} else { // 否则添加到C链表
if (*C == NULL) {
*C = current;
} else {
(*C)->next = current;
}
*C = current; // 更新C链表头
}
current = current->next;
}
}
// 打印链表
void print_list(Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
int main() {
Node* A = create_node(-5); // 创建一个示例顺序表
A->next = create_node(10);
A->next->next = create_node(-3);
A->next->next->next = create_node(7);
A->next->next->next->next = create_node(-1);
Node* B = NULL;
Node* C = NULL;
split_list(A, &B, &C);
printf("List A: ");
print_list(A);
printf("\nList B: ");
print_list(B);
printf("\nList C: ");
print_list(C);
return 0;
}
```
在这个程序中,我们首先创建了一个顺序表A,然后通过`split_list`函数将其分解为小于0的元素在B链表,大于0的元素在C链表。最后打印出原始列表A以及分解后的列表B和C。
阅读全文
相关推荐
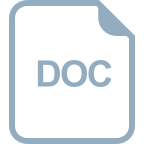
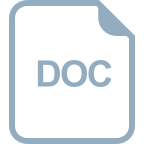















