用C++写一个输入为两条线段,类型为x,y组成的p1,p2,p3,p4,判断两条线段可延长的情况下是否相交
时间: 2024-03-28 16:42:10 浏览: 13
以下是用C++实现的判断两条线段是否相交的代码:
```
#include <iostream>
using namespace std;
struct Point {
double x, y;
};
bool onSegment(Point p, Point q, Point r) {
if (q.x <= max(p.x, r.x) && q.x >= min(p.x, r.x) &&
q.y <= max(p.y, r.y) && q.y >= min(p.y, r.y))
return true;
return false;
}
int orientation(Point p, Point q, Point r) {
double val = (q.y - p.y) * (r.x - q.x) - (q.x - p.x) * (r.y - q.y);
if (val == 0) return 0;
return (val > 0) ? 1 : 2;
}
bool doIntersect(Point p1, Point q1, Point p2, Point q2) {
int o1 = orientation(p1, q1, p2);
int o2 = orientation(p1, q1, q2);
int o3 = orientation(p2, q2, p1);
int o4 = orientation(p2, q2, q1);
if (o1 != o2 && o3 != o4)
return true;
if (o1 == 0 && onSegment(p1, p2, q1)) return true;
if (o2 == 0 && onSegment(p1, q2, q1)) return true;
if (o3 == 0 && onSegment(p2, p1, q2)) return true;
if (o4 == 0 && onSegment(p2, q1, q2)) return true;
return false;
}
int main() {
Point p1 = {1, 1}, q1 = {10, 1};
Point p2 = {1, 2}, q2 = {10, 2};
if (doIntersect(p1, q1, p2, q2))
cout << "Yes\n";
else
cout << "No\n";
return 0;
}
```
其中,`Point` 结构体表示点的坐标,`onSegment` 函数用来判断一个点是否在线段上,`orientation` 函数用来判断三个点的方向关系,`doIntersect` 函数用来判断两条线段是否相交。
在 `main` 函数中,我们定义了两条线段 `p1-q1` 和 `p2-q2`,然后调用 `doIntersect` 函数来判断它们是否相交,如果相交则输出 "Yes",否则输出 "No"。
相关推荐













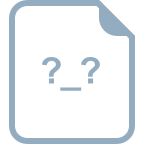