求矩阵的幂次方c++
时间: 2024-09-27 22:07:30 浏览: 51
在C++中,求解矩阵的幂次方通常涉及到矩阵快速幂算法(Matrix Exponentiation),这是一种高效的计算方法,用于计算大整数幂或者矩阵乘法若干次的结果。以下是基本步骤:
1. 定义一个二维动态数组(如`std::vector<std::vector<int>>`)来存储矩阵。
2. 实现一个函数来完成矩阵乘法,例如`std::vector<std::vector<int>> multiply(const std::vector<std::vector<int>>& a, const std::vector<std::vector<int>>& b)`,该函数将两个矩阵相乘,并返回结果。
3. 使用递归或迭代的方式实现矩阵的幂运算。可以创建一个辅助函数`matrixPower(std::vector<std::vector<int>>& matrix, int n)`,它接收矩阵和指数作为输入,初始条件是当指数为0时返回单位矩阵(所有元素为1的矩阵),当指数为正时,将原矩阵与自身乘n-1次(通过递归实现)。
4. 主函数中,传入矩阵A和所需的幂次n,调用`matrixPower(A, n)`得到最终结果。
下面是简化版的示例代码片段:
```cpp
#include <vector>
#include <cmath>
// 矩阵乘法函数
std::vector<std::vector<int>> multiply(const std::vector<std::vector<int>>& a, const std::vector<std::vector<int>>& b) {
//...
}
// 动态规划版本的矩阵快速幂函数
std::vector<std::vector<int>> matrixPower(std::vector<std::vector<int>>& matrix, int n) {
if (n == 0) return {{1, 0}, {0, 1}}; // 单位矩阵
std::vector<std::vector<int>> result = matrixPower(matrix, n / 2);
result = multiply(result, result);
if (n % 2 == 1) result = multiply(result, matrix); // 如果n是奇数,还需要额外乘一次原矩阵
return result;
}
int main() {
std::vector<std::vector<int>> A = {{1, 2}, {3, 4}};
int n = 3; // 要求A的3次方
std::vector<std::vector<int>> result = matrixPower(A, n);
// 打印结果
//...
阅读全文
相关推荐
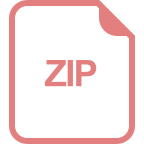
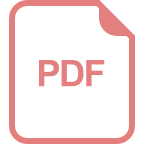
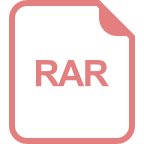
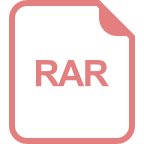
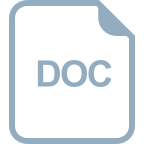
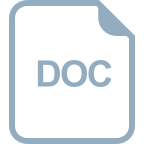












